Suggested Videos
Part 20 - Angular 2 container and nested components | Text | Slides
Part 21 - Angular component input properties | Text | Slides
Part 22 - Angular component output properties | Text | Slides
In this video we will discuss, what are interfaces and when to use them in Angular.
What is an Interface in TypeScript
If you have experience with any object oriented oriented programming language like C#, Java or C++, then you know an interface is an abstract type. It only contain declarations of properties, methods and events. The implementation for the interface members is provided by a class that implements the interface.
If a class that implements the interface fails to provide implementation for all the interface members, the language compiler raises an error alerting the developer that something has been missed.
In general, an interface defines a contract (i.e the shape or structure of an API), that an implementing class must adhere to. Even in TypeScript we use the interface for the same purpose.
We know TypeScript is a strongly typed language. This means every property we define in a TypeScript class has a type associated with it. Similarly every method parameter and return type has a type. As you know by now, TypeScript has several built-in pre-defined types like string, number, boolean etc.
However, the business objects that we usually create in real-world applications like Employee, Customer, Order, Invoice etc, does not have a pre-defined type. In this case, we can use an interface to create a custom type for our business object.
Let us understand this with an example. We will use the same example we worked with in Part 22 of Angular 2 tutorial.
Notice the below line of code in EmployeeListComponent class. The property "employees" is defined as an array of any type.
employees: any[];
Since we do not have a Type for employee object, we specified the type as any.
There are 2 problems with the above line of code
1. For the object properties in the array we do not get intellisense
2. Since we do not get intellisense, we are prone to making typographical errors and the compiler will not be able to flag them as errors. We will come to know about these errors only at runtime.
Let's create a Type for employee using an interface as shown below. Add a new TypeScript file to the employee folder. Name it employee.ts. Copy and paste the following code.
With this IEmployee interface in place, we can now import and use the interface type as the type for "employees" property. The code in EmployeeListComponent class is shown below.
Since we have specified a type for the "employees" property, we now get intellisense for the object properties in the array
If we make any typographical errors with the property names, we will get to know these errors right away at compile time.
While we are here, let's discuss some of the concepts related to TypeScript interfaces. Conside the following interface. The code is commented and self-explanatory.
Here is the shorthand syntax to initialise class properties with constructor parameters
The above shorthand syntax is not limited to public class properties. We can also use it with private class properties. In the example below we have 2 private properties (firstName & lastName) which are initialised with class constructor.
We can rewrite the above code using shorthand syntax as shown below. In both the cases the generated JavaScript code is the same.
Interfaces in TypeScript
Part 20 - Angular 2 container and nested components | Text | Slides
Part 21 - Angular component input properties | Text | Slides
Part 22 - Angular component output properties | Text | Slides
In this video we will discuss, what are interfaces and when to use them in Angular.
What is an Interface in TypeScript
If you have experience with any object oriented oriented programming language like C#, Java or C++, then you know an interface is an abstract type. It only contain declarations of properties, methods and events. The implementation for the interface members is provided by a class that implements the interface.
If a class that implements the interface fails to provide implementation for all the interface members, the language compiler raises an error alerting the developer that something has been missed.
In general, an interface defines a contract (i.e the shape or structure of an API), that an implementing class must adhere to. Even in TypeScript we use the interface for the same purpose.
We know TypeScript is a strongly typed language. This means every property we define in a TypeScript class has a type associated with it. Similarly every method parameter and return type has a type. As you know by now, TypeScript has several built-in pre-defined types like string, number, boolean etc.
However, the business objects that we usually create in real-world applications like Employee, Customer, Order, Invoice etc, does not have a pre-defined type. In this case, we can use an interface to create a custom type for our business object.
Let us understand this with an example. We will use the same example we worked with in Part 22 of Angular 2 tutorial.
Notice the below line of code in EmployeeListComponent class. The property "employees" is defined as an array of any type.
employees: any[];
Since we do not have a Type for employee object, we specified the type as any.
There are 2 problems with the above line of code
1. For the object properties in the array we do not get intellisense

2. Since we do not get intellisense, we are prone to making typographical errors and the compiler will not be able to flag them as errors. We will come to know about these errors only at runtime.
Let's create a Type for employee using an interface as shown below. Add a new TypeScript file to the employee folder. Name it employee.ts. Copy and paste the following code.
export interface IEmployee {
code: string;
name: string;
gender: string;
annualSalary: number;
dateOfBirth: string;
}
With this IEmployee interface in place, we can now import and use the interface type as the type for "employees" property. The code in EmployeeListComponent class is shown below.
import { IEmployee } from './employee';
export class
EmployeeListComponent {
employees: IEmployee[];
}
Since we have specified a type for the "employees" property, we now get intellisense for the object properties in the array
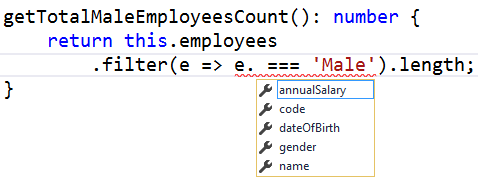
If we make any typographical errors with the property names, we will get to know these errors right away at compile time.
While we are here, let's discuss some of the concepts related to TypeScript interfaces. Conside the following interface. The code is commented and self-explanatory.
export interface IEmployee {
code: string;
name: string;
gender: string;
annualSalary: number;
dateOfBirth: string;
// To make a
property optional use a ?
// A class that
implements this interface need
// not provide
implementation for this property
department?: string;
computeMonthlySalary(annualSalary: number): number;
}
export class Employee
implements IEmployee {
// All the
interface mandatory properties are defined
public code: string;
public name: string;
public gender: string;
public annualSalary: number;
public dateOfBirth: string;
// The above
class properties are then initialized
// using the
constructor parameters. To do something
// like this,
TypeScript has a shorthand syntax which
// reduces the
amount of code we have to write
constructor(code: string, name: string, gender: string,
annualSalary: number, dateOfBirth: string) {
this.code = code;
this.name = name;
this.gender = gender;
this.annualSalary = annualSalary;
this.dateOfBirth = dateOfBirth;
}
//
Implementation of the interface method
computeMonthlySalary(annualSalary: number): number {
return annualSalary / 12;
}
}
Here is the shorthand syntax to initialise class properties with constructor parameters
export interface IEmployee {
code: string;
name: string;
gender: string;
annualSalary: number;
dateOfBirth: string;
}
export class Employee
implements IEmployee {
constructor(public code: string, public name: string, public gender: string,
public annualSalary: number, public
dateOfBirth: string) {
}
}
The above shorthand syntax is not limited to public class properties. We can also use it with private class properties. In the example below we have 2 private properties (firstName & lastName) which are initialised with class constructor.
export class Employee
{
private firstName: string;
private lastName: string;
constructor(firstName: string,
lastName: string) {
this.firstName = firstName;
this.lastName = lastName;
}
}
We can rewrite the above code using shorthand syntax as shown below. In both the cases the generated JavaScript code is the same.
export class Employee
{
constructor(private
firstName: string, private lastName: string) {
}
}
Interfaces in TypeScript
- Use interface keyword to create an interface
- It is common to prefix the interface name with capital letter "I". However, some interfaces in Angular does not have the prefix "I". For example, OnInit interface
- Interface members are public by default and does not require explicit access modifiers. It is a compile time error to include an explicit access modifier. You will see an error message like - public modifier cannot appear on a type member.
- A class that implements an interface must provide implementation for all the interface members unless the members are marked as optional using the ? operator
- Use the implements keyword to make a class implement an interface
- TypeScript interfaces exist for developer convenience and are not used by Angular at runtime. During transpilation, no JavaScript code is generated for an interface. It is only used by Typescript for type checking during development.
- To reduce the amount of code you have to write, consider using short-hand syntax to initialise class properties with constructor parameters
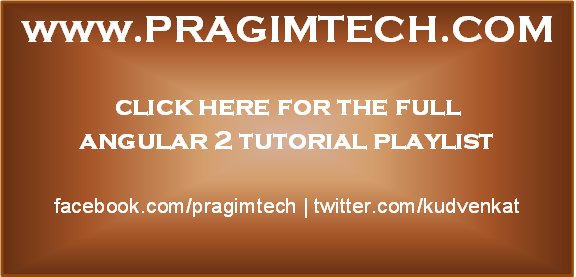
Sir its very good effort thanks alot :)
ReplyDeleteI got an issue when trying this: We can rewrite the above code using shorthand syntax as shown below. In both the cases the generated JavaScript code is the same.
ReplyDeleteexport class Employee {
constructor(private firstName: string, private lastName: string) {
}
}
- Whenever I try to implement an interface and make firstName private, it is telling that firstName is public in interface
Properties in an interface are public by default, you can't have private properties in an "Interface", the above example has 2 private properties in a class called
DeleteEmployee and not in the interface. Move any private properties to your implementation class and then try to use the short hand syntax for the constructor, that should resolve your problem
Possibly you may have specified the access modifier for the firstName property as public instead of private, ensure it is declared as below within the Employee class:
ReplyDeleteprivate firstName: string;
KudVenkat is gift of god to the IT employes.Simple and Clear Explanation.
ReplyDelete