Suggested Videos
Part 10 - html attribute vs dom property | Text | Slides
Part 11 - Angular attribute binding | Text | Slides
Part 12 - Class binding in angular 2 | Text | Slides
In this video we will discuss Style binding in Angular with examples.
Setting inline styles with style binding is very similar to setting CSS classes with class binding. Please watch Class binding video from Angular 2 tutorial before proceeding with this video.
Notice in the example below, we have set the font color of the button using the style attribute.
The following example sets a single style (font-weight). If the property 'isBold' is true, then font-weight style is set to bold else normal.
style property name can be written in either dash-case or camelCase. For example, font-weight style can also be written using camel case - fontWeight.
Some styles like font-size have a unit extension. To set font-size in pixels use the following syntax. This example sets font-size to 30 pixels.
To set multiple inline styles use NgStyle directive as shown below
Part 10 - html attribute vs dom property | Text | Slides
Part 11 - Angular attribute binding | Text | Slides
Part 12 - Class binding in angular 2 | Text | Slides
In this video we will discuss Style binding in Angular with examples.
Setting inline styles with style binding is very similar to setting CSS classes with class binding. Please watch Class binding video from Angular 2 tutorial before proceeding with this video.
Notice in the example below, we have set the font color of the button using the style attribute.
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
template: `
<button
style="color:red">My Button</button>
`
})
export class
AppComponent {
}
The following example sets a single style (font-weight). If the property 'isBold' is true, then font-weight style is set to bold else normal.
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
template: `
<button style='color:red'
[style.font-weight]="isBold
? 'bold' : 'normal'">My Button
</button>
`
})
export class
AppComponent {
isBold: boolean = true;
}
style property name can be written in either dash-case or camelCase. For example, font-weight style can also be written using camel case - fontWeight.
Some styles like font-size have a unit extension. To set font-size in pixels use the following syntax. This example sets font-size to 30 pixels.
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
template: `
<button style='color:red'
[style.font-size.px]="fontSize">My Button
</button>
`
})
export class
AppComponent {
fontSize: number = 30;
}
To set multiple inline styles use NgStyle directive as shown below
- Notice the color style is added using the style attribute
- ngStyle is binded to addStyles() method of the AppComponent class
- addStyles() method returns an object with 2 key/value pairs. The key is a style name, and the value is a value for the respective style property or an expression that returns the style value.
- let is a new type of variable declaration in JavaScript.
- let is similar to var in some respects but allows us to avoid some of the common gotchas that we run into when using var.
- The differences between let and var are beyond the scope of this video. For our example, var also works fine.
- As TypeScript is a superset of JavaScript, it supports let
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
template: `
<button style='color:red' [ngStyle]="addStyles()">My
Button</button>
`
})
export class
AppComponent {
isBold: boolean = true;
fontSize: number = 30;
isItalic: boolean = true;
addStyles() {
let styles = {
'font-weight': this.isBold ?
'bold' : 'normal',
'font-style': this.isItalic
? 'italic' : 'normal',
'font-size.px': this.fontSize
};
return styles;
}
}
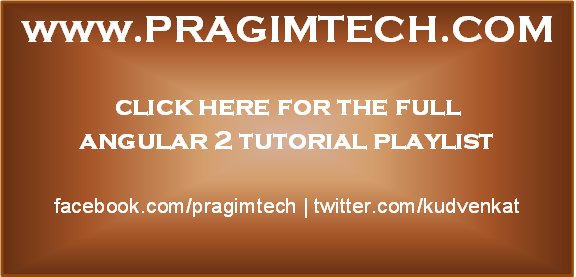
No comments:
Post a Comment
It would be great if you can help share these free resources