Suggested Videos
Part 9 - Property binding in Angular 2 | Text | Slides
Part 10 - html attribute vs dom property | Text | Slides
Part 11 - Angular attribute binding | Text | Slides
In this video we will discuss CSS Class binding in Angular with examples.
For the demos in this video, we will use same example we have been working with so far in this video series. In styles.css file include the following 3 CSS classes. If you recollect styles.css is already referenced in our host page - index.html.
In app.component.ts, include a button element as shown below. Notice we have set the class attribute of the button element to 'colorClass'.
Replace all the existing css classes with one or more classes
Modify the code in app.component.ts as shown below.
Run the application and notice 'colorClass' is removed and these classes (italicsClass & boldClass) are added.
Adding or removing a single class : To add or remove a single class, include the prefix 'class' in a pair of square brackets, followed by a DOT and then the name of the class that you want to add or remove. The following example adds boldClass to the button element. Notice it does not remove the existing colorClass already added using the class attribute. If you change applyBoldClass property to false or remove the property altogether from the AppComponent class, css class boldClass is not added to the button element.
With class binding we can also use ! symbol. Notice in the example below applyBoldClass is set to false. Since we have used ! in the class binding the class is added as expected.
You can also removed an existing class that is already applied. Consider the following example. Notice we have 3 classes (colorClass, boldClass & italicsClass) added to the button element using the class attribute. The class binding removes the boldClass.
To add or remove multiple classes use ngClass directive as shown in the example below.
We have included our css classes in a external stylesheet - styles.css. Please note we can also include these classes in the styles property instead of a separate stylesheet as shown below.
Part 9 - Property binding in Angular 2 | Text | Slides
Part 10 - html attribute vs dom property | Text | Slides
Part 11 - Angular attribute binding | Text | Slides
In this video we will discuss CSS Class binding in Angular with examples.
For the demos in this video, we will use same example we have been working with so far in this video series. In styles.css file include the following 3 CSS classes. If you recollect styles.css is already referenced in our host page - index.html.
.boldClass{
font-weight:bold;
}
.italicsClass{
font-style:italic;
}
.colorClass{
color:red;
}
In app.component.ts, include a button element as shown below. Notice we have set the class attribute of the button element to 'colorClass'.
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
template: `
<button
class='colorClass'>My Button</button>
`
})
export class
AppComponent {
}
At this point, run the application and notice that the 'colorClass' is added to the button element as expected.Replace all the existing css classes with one or more classes
Modify the code in app.component.ts as shown below.
- We have introduced a property 'classesToApply' in AppComponent class
- We have also specified class binding for the button element. The word 'class' is in a pair of square brackets and it is binded to the property 'classesToApply'
- This will replace the existing css classes of the button with classes specified in the class binding
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
template: `
<button class='colorClass'
[class]='classesToApply'>My Button</button>
`
})
export class
AppComponent {
classesToApply: string = 'italicsClass
boldClass';
}
Run the application and notice 'colorClass' is removed and these classes (italicsClass & boldClass) are added.
Adding or removing a single class : To add or remove a single class, include the prefix 'class' in a pair of square brackets, followed by a DOT and then the name of the class that you want to add or remove. The following example adds boldClass to the button element. Notice it does not remove the existing colorClass already added using the class attribute. If you change applyBoldClass property to false or remove the property altogether from the AppComponent class, css class boldClass is not added to the button element.
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
template: `
<button class='colorClass'
[class.boldClass]='applyBoldClass'>My Button</button>
`
})
export class
AppComponent {
applyBoldClass: boolean = true;
}
With class binding we can also use ! symbol. Notice in the example below applyBoldClass is set to false. Since we have used ! in the class binding the class is added as expected.
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
template: `
<button class='colorClass'
[class.boldClass]='!applyBoldClass'>My Button</button>
`
})
export class
AppComponent {
applyBoldClass: boolean = false;
}
You can also removed an existing class that is already applied. Consider the following example. Notice we have 3 classes (colorClass, boldClass & italicsClass) added to the button element using the class attribute. The class binding removes the boldClass.
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
template: `
<button class='colorClass
boldClass italicsClass'
[class.boldClass]='applyBoldClass'>My Button</button>
`
})
export class
AppComponent {
applyBoldClass: boolean = false;
}
To add or remove multiple classes use ngClass directive as shown in the example below.
- Notice the colorClass is added using the class attribute
- ngClass is binded to addClasses() method of the AppComponent class
- addClasses() method returns an object with 2 key/value pairs. The key is a CSS class name. The value can be true or false. True to add the class and false to remove the class.
- Since both the keys (boldClass & italicsClass) are set to true, both classes will be added to the button element
- let is a new type of variable declaration in JavaScript.
- let is similar to var in some respects but allows us to avoid some of the common gotchas that we run into when using var.
- The differences between let and var are beyond the scope of this video. For our example, var also works fine.
- As TypeScript is a superset of JavaScript, it supports let
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
template: `
<button class='colorClass'
[ngClass]='addClasses()'>My Button</button>
`
})
export class AppComponent
{
applyBoldClass: boolean = true;
applyItalicsClass: boolean = true;
addClasses() {
let classes = {
boldClass: this.applyBoldClass,
italicsClass: this.applyItalicsClass
};
return classes;
}
}
We have included our css classes in a external stylesheet - styles.css. Please note we can also include these classes in the styles property instead of a separate stylesheet as shown below.
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
template: `
<button class='colorClass'
[ngClass]='addClasses()'>My Button</button>
`,
styles: [`
.boldClass{
font-weight:bold;
}
.italicsClass{
font-style:italic;
}
.colorClass{
color:red;
}
`]
})
export class
AppComponent {
applyBoldClass: boolean = true;
applyItalicsClass: boolean = true;
addClasses() {
let classes = {
boldClass: this.applyBoldClass,
italicsClass: this.applyItalicsClass
};
return classes;
}
}
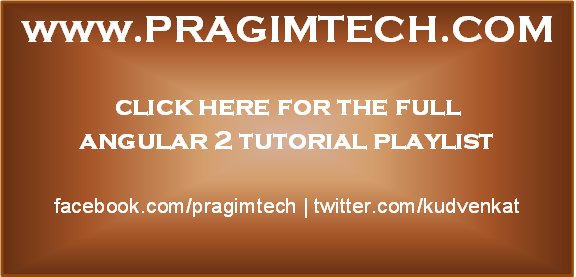
I tried to add multiple classes using above example, I ended up with following error.
ReplyDeleteAppComponent.ngfactory.js:16 ERROR TypeError: _co.addClasses is not a function
at Object.View_AppComponent_0._co [as updateDirectives] (app.component.html:7)
at Object.debugUpdateDirectives [as updateDirectives] (core.umd.js:13107)
Enclosing the classes in single quotes fixed this for me as below.
Deletelet classes = {
'boldClass': this.applyBoldClass,
'italicsClass': this.applyItalicsClass
};