Suggested Videos
Part 5 - Angular template vs templateurl | Text | Slides
Part 6 - Angular 2 nested components | Text | Slides
Part 7 - Styling angular 2 components | Text | Slides
In this video we will discuss the concept of Interpolation in Angular.
Interpolation is all about data binding. In Angular data-binding can be broadly classified into 3 categories
In this video, we will discuss the first one way data-binding i.e From Component to View Template. We achieve this using interpolation. We will discuss the rest of the 2 data-binding techniques in our upcoming videos.
One way data-binding - From Component to View Template : To display read-only data on a view template we use one-way data binding technique interpolation. With interpolation, we place the component property name in the view template, enclosed in double curly braces: {{propertyName}}.
In the following example, Angular pulls the value of the firstName property from the component and inserts it between the opening and closing <h1> element.
Output :
It is also possible to concatenate a hard-coded string with the property value
The above expression displayes "Name = Tom" in the browser.
You can specify any valid expression in double curly braces. For example you can have
The above expression evaluates to 60
The expression that is enclosed in double curly braces is commonly called as Template Expression. This template expression can also be a ternary operator as shown in the example below. Since firstName property has a value 'Tom', we see it in the browser.
If we set firstName = null as shown below. The value 'No name specified' is displayed in the browser
firstName: string = null;
You can also use interpolation to set <img> src as shown in the example below.
Output :
We can also call class methods using interpolation as shown below.
Output : Full Name = Tom Hopkins
Part 5 - Angular template vs templateurl | Text | Slides
Part 6 - Angular 2 nested components | Text | Slides
Part 7 - Styling angular 2 components | Text | Slides
In this video we will discuss the concept of Interpolation in Angular.
Interpolation is all about data binding. In Angular data-binding can be broadly classified into 3 categories
Data Binding | Description |
One way data-binding | From Component to View Template |
One way data-binding | From View Template to Component |
Two way data-binding | From Component to View Template & From View template to Component |
In this video, we will discuss the first one way data-binding i.e From Component to View Template. We achieve this using interpolation. We will discuss the rest of the 2 data-binding techniques in our upcoming videos.
One way data-binding - From Component to View Template : To display read-only data on a view template we use one-way data binding technique interpolation. With interpolation, we place the component property name in the view template, enclosed in double curly braces: {{propertyName}}.
In the following example, Angular pulls the value of the firstName property from the component and inserts it between the opening and closing <h1> element.
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
template: `
<h1>{{ firstName
}}</h1>
`
})
export class
AppComponent {
firstName: string = 'Tom';
}
Output :

It is also possible to concatenate a hard-coded string with the property value
<h1>{{'Name = ' +
firstName}}</h1>
The above expression displayes "Name = Tom" in the browser.
You can specify any valid expression in double curly braces. For example you can have
<h1>{{ 10 + 20 + 30
}}</h1>
The above expression evaluates to 60
The expression that is enclosed in double curly braces is commonly called as Template Expression. This template expression can also be a ternary operator as shown in the example below. Since firstName property has a value 'Tom', we see it in the browser.
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
template: `
<h1>{{firstName ?
firstName : 'No name specified'}}</h1>
`
})
export class
AppComponent {
firstName: string = 'Tom';
}
If we set firstName = null as shown below. The value 'No name specified' is displayed in the browser
firstName: string = null;
You can also use interpolation to set <img> src as shown in the example below.
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
template: `<div>
<h1>{{pageHeader}}</h1>
<img
src='{{imagePath}}'/>
</div>`
})
export class
AppComponent {
pageHeader: string = 'Employee Details';
imagePath: string = 'http://pragimtech.com/images/logo.jpg';
}
Output :

We can also call class methods using interpolation as shown below.
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
template: `<div>
<h1>{{'Full Name = '
+ getFullName()}}</h1>
</div>`
})
export class
AppComponent {
firstName: string = 'Tom';
lastName: string = 'Hopkins';
getFullName(): string {
return this.firstName
+ ' ' + this.lastName;
}
}
Output : Full Name = Tom Hopkins
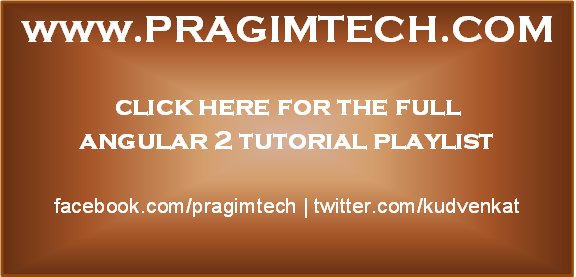
Dear Venkat Garu,
ReplyDeleteI am presently working on c#.net and i would like to learn Angular2 Completly....Can u please tell me how can i start and work
Hi sir,
ReplyDeleteI am getting an error while using method getFullName() in console there have an error like below.
(SystemJS) No template specified for component AppComponent
you cant print the function in console..being inside the same function
Deleteyou might have not mentioned template in the component.
ReplyDeleteHi
ReplyDeletewhatever things i am updating in html is not getting reflected