Suggested Videos
Part 22 - Using asp.net identity with Web API
Part 23 - Using fiddler to test ASP.NET Web API token based authentication
Part 24 - ASP.NET Web API login page
In this video we will discuss how to use bearer token for authentication and retrieving data from the server. This is continuation to Part 24. Please watch Part 24 from ASP.NET Web API tutorial before proceeding.
We want to implement a page that retrieves employee data from the server. If the user is not authenticated, he should be automatically redirected to the login page. The user should be able to get to the data page, only if he is logged in.
Add a new HTML page to the EmployeeService project. Name it Data.html page. Copy and paste the following HTML and jQuery code.
At the moment, the only way to log off the user is by closing the browser window. As we are storing the bearer token in browser session storage, when we close the browser we loose it from the session. In our next video we will discuss, how to explicitly log out the user without closing the browser window.
Part 22 - Using asp.net identity with Web API
Part 23 - Using fiddler to test ASP.NET Web API token based authentication
Part 24 - ASP.NET Web API login page
In this video we will discuss how to use bearer token for authentication and retrieving data from the server. This is continuation to Part 24. Please watch Part 24 from ASP.NET Web API tutorial before proceeding.
We want to implement a page that retrieves employee data from the server. If the user is not authenticated, he should be automatically redirected to the login page. The user should be able to get to the data page, only if he is logged in.

Add a new HTML page to the EmployeeService project. Name it Data.html page. Copy and paste the following HTML and jQuery code.
<!DOCTYPE html>
<html>
<head>
<title></title>
<meta charset="utf-8" />
<link href="Content/bootstrap.min.css" rel="stylesheet" />
</head>
<body
style="padding-top:20px">
<div class="col-md-10
col-md-offset-1">
<div class="well">
<input id="btnLoadEmployees" class="btn btn-success"
type="button" value="Load Employees" />
</div>
<div id="divData" class="well hidden">
<table class="table
table-bordered"
id="tblData">
<thead>
<tr class="success">
<td>ID</td>
<td>First Name</td>
<td>Last Name</td>
<td>Gender</td>
<td>Salary</td>
</tr>
</thead>
<tbody
id="tblBody"></tbody>
</table>
</div>
<div class="modal fade" tabindex="-1" id="errorModal"
data-keyboard="false" data-backdrop="static">
<div class="modal-dialog
modal-sm">
<div
class="modal-content">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal">
×
</button>
<h4 class="modal-title">Session Expired</h4>
</div>
<div class="modal-body">
<form>
<h2
class="modal-title">Close this message to login
again</h2>
</form>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-danger"
data-dismiss="modal">
Close
</button>
</div>
</div>
</div>
</div>
<div id="divError" class="alert alert-danger
collapse">
<a id="linkClose" href="#" class="close">×</a>
<div id="divErrorText"></div>
</div>
</div>
<script src="Scripts/jquery-1.10.2.min.js"></script>
<script src="Scripts/bootstrap.min.js"></script>
<script type="text/javascript">
$(document).ready(function () {
if
(sessionStorage.getItem('accessToken')
== null) {
window.location.href = "Login.html";
}
$('#linkClose').click(function () {
$('#divError').hide('fade');
});
$('#errorModal').on('hidden.bs.modal', function () {
window.location.href = "Login.html";
});
$('#btnLoadEmployees').click(function () {
$.ajax({
url: '/api/employees',
method: 'GET',
headers: {
'Authorization': 'Bearer '
+
sessionStorage.getItem("accessToken")
},
success: function (data) {
$('#divData').removeClass('hidden');
$('#tblBody').empty();
$.each(data, function (index, value) {
var row = $('<tr><td>' + value.ID + '</td><td>'
+
value.FirstName + '</td><td>'
+
value.LastName + '</td><td>'
+ value.Gender
+ '</td><td>'
+ value.Salary
+ '</td></tr>');
$('#tblData').append(row);
});
},
error: function (jQXHR) {
// If status code is 401,
access token expired, so
// redirect the user to the
login page
if (jQXHR.status == "401") {
$('#errorModal').modal('show');
}
else {
$('#divErrorText').text(jqXHR.responseText);
$('#divError').show('fade');
}
}
});
});
});
</script>
</body>
</html>
At the moment, the only way to log off the user is by closing the browser window. As we are storing the bearer token in browser session storage, when we close the browser we loose it from the session. In our next video we will discuss, how to explicitly log out the user without closing the browser window.
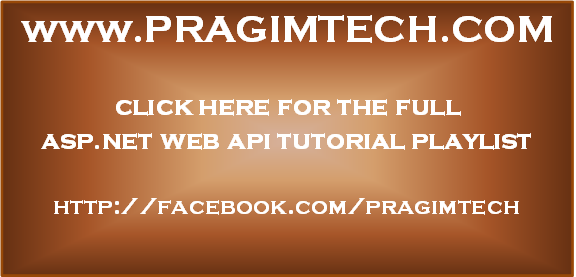
You forgot to add this line of code in error function $('#errorModal').click(function () {
ReplyDeletewindow.location.href = "Login.html"
correct me if I am wrong.
$('#errorModal').on('hidden.bs.modal', function () {
Deletewindow.location.href = "Login.html";
});
Very Special Thanks from Egypt
ReplyDeleteHow can I secure a JWT access token by tying it to one machine?
ReplyDeleteI can take token value and cookie identity from a browser which is signed in to my site and has a valid token, to another browser and access authorized actions?
I tried to exactly same but my token is not expiring. I have made changed in Startup class too.. Please help someone!!! Urgently
ReplyDeleteIn App_Start folder -> Startup.Auth.cs -->
ReplyDeleteGiven line:
AccessTokenExpireTimeSpan = TimeSpan.FromDays(14),
Replace it as:
AccessTokenExpireTimeSpan = TimeSpan.FromSeconds(10), //Check for 10 Seconds
After clicking 'Load Employees' button on Data.html page, wait for 10 seconds and then click on 'Load Employees' button, then it will show Session expired popup window.
This step has already explained in video by Kudvenkat sir.
Nice tutorial. Working well.
even then it is not expirirng
ReplyDeleteHave you added [Authorize] keyword before your EmployeeController?
Delete