Suggested Videos
Part 18 - Implementing basic authentication in ASP.NET Web API
Part 19 - Call web api service with basic authentication using jquery ajax
Part 20 - ASP.NET Web API token authentication
In this video we will discuss implementing new user registration page. This is continuation to Part 20. Please watch Part 20 from ASP.NET Web API tutorial before proceeding.
The registration page should be as shown below.
For a new user to register they have to provide
1. Email address
2. Password
3. Confirm password
When all the fields are provided and when the Register button is clicked, the new user details should be saved and a modal dialog should be displayed as shown below.
To achieve this, add an HTML page to EmployeeService project. Name it Register.html. Copy and paste the following HTML and jQuery code.
Please note :
1. The ajax() method posts the data to '/api/account/register'
2. You will find the Register() method in AccountController in Controllers folder
3. AccountController is provided by ASP.NET Web API, which saves data to a local membership database
In our next video we will discuss where the users registration data is stored and all the customizations that can be made.
Part 18 - Implementing basic authentication in ASP.NET Web API
Part 19 - Call web api service with basic authentication using jquery ajax
Part 20 - ASP.NET Web API token authentication
In this video we will discuss implementing new user registration page. This is continuation to Part 20. Please watch Part 20 from ASP.NET Web API tutorial before proceeding.
The registration page should be as shown below.

For a new user to register they have to provide
1. Email address
2. Password
3. Confirm password
When all the fields are provided and when the Register button is clicked, the new user details should be saved and a modal dialog should be displayed as shown below.

To achieve this, add an HTML page to EmployeeService project. Name it Register.html. Copy and paste the following HTML and jQuery code.
<!DOCTYPE html>
<html>
<head>
<title></title>
<meta charset="utf-8" />
<link href="Content/bootstrap.min.css" rel="stylesheet" />
</head>
<body
style="padding-top:20px">
<div class="col-md-10
col-md-offset-1">
<div class="well">
<!--This table contains the fields that we want
to capture to register a new user-->
<table class="table
table-bordered">
<thead>
<tr class="success">
<th colspan="2">
New User
Registration
</th>
</tr>
</thead>
<tbody>
<tr>
<td>Email</td>
<td><input type="text" id="txtEmail" placeholder="Email" /> </td>
</tr>
<tr>
<td>Password</td>
<td><input type="password" id="txtPassword"
placeholder="Password" /></td>
</tr>
<tr>
<td>Confirm Password</td>
<td><input type="password" id="txtConfirmPassword"
placeholder="Confirm Password" /></td>
</tr>
<tr class="success">
<td colspan="2">
<input id="btnRegister" class="btn btn-success"
type="button" value="Register" />
</td>
</tr>
</tbody>
</table>
<!--Bootstrap modal dialog that shows up when
regsitration is successful-->
<div class="modal fade" tabindex="-1" id="successModal"
data-keyboard="false" data-backdrop="static">
<div
class="modal-dialog
modal-sm">
<div class="modal-content">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal">
×
</button>
<h4 class="modal-title">Success</h4>
</div>
<div class="modal-body">
<form>
<h2 class="modal-title">Registration Successful!</h2>
</form>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-success"
data-dismiss="modal">
Close
</button>
</div>
</div>
</div>
</div>
<!--Bootstrap alert to display any validation
errors-->
<div id="divError" class="alert alert-danger
collapse">
<a
id="linkClose" href="#" class="close">×</a>
<div
id="divErrorText"></div>
</div>
</div>
</div>
<script src="Scripts/jquery-1.10.2.min.js"></script>
<script src="Scripts/bootstrap.min.js"></script>
<script type="text/javascript">
$(document).ready(function () {
//Close the bootstrap alert
$('#linkClose').click(function () {
$('#divError').hide('fade');
});
// Save the new user details
$('#btnRegister').click(function () {
$.ajax({
url: '/api/account/register',
method: 'POST',
data: {
email: $('#txtEmail').val(),
password: $('#txtPassword').val(),
confirmPassword: $('#txtConfirmPassword').val()
},
success: function () {
$('#successModal').modal('show');
},
error: function (jqXHR) {
$('#divErrorText').text(jqXHR.responseText);
$('#divError').show('fade');
}
});
});
});
</script>
</body>
</html>
Please note :
1. The ajax() method posts the data to '/api/account/register'
2. You will find the Register() method in AccountController in Controllers folder
3. AccountController is provided by ASP.NET Web API, which saves data to a local membership database
In our next video we will discuss where the users registration data is stored and all the customizations that can be made.
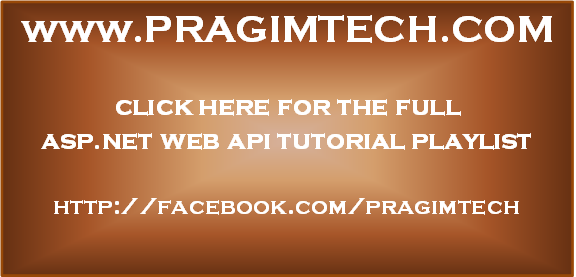
Hello everyone i am showing a webpage using Iframe i am also passing ID and Article from my gridview but now i want to show this webpage using POPUP window.How to do that. Please Help. Here is my code
ReplyDeleteIframe1.Attributes.Add("src", "Drawing2.aspx?ID=" + grdrow.Cells[1].Text + "&Article=" + grdrow.Cells[2].Text + "&testdrawing= kkk");
use window.open
ReplyDeletei am getting 400 error like this
ReplyDeleteFailed to load resource: the server responded with a status of 400 (Bad Request)
When u r doing a ajax request make sure your URL has a absolute address
Deleteif you using MVC then your url should be like this @url.action(action,controller)..or
Deletemake a hidden file in view and specify url.
and get that filed val in jquery...
or
if you using webforms then your url should be like this.
url:'xyz.aspx/funtionName',
thank you
while running same code in my pc this error is coming.please sir help in this
ReplyDelete{"Message":"The request is invalid.","ModelState":{"model.UserName":["The UserName field is required."]}}
in ajax call change property email to UserName
ReplyDeleteyes change it
Deletecan you please add the code of accountcontroller
ReplyDeleteIt is auto-generated code which is already available under Controllers folder --> AccountController.cs file.
DeleteHi I hope you can still see this, but i am still trying to learn how to do all this.
ReplyDeleteBecause I created a Web API project that will be the "Web Service" for my project. My Web API contains the Identity Framework, now I need to create a MVC client project that will connect ( Register/Login/Interact ) to my Web API Project.
I am aware that i need to have the same model structure as my web api project to be able to access the things i need on my Web API.
My question is, do i need to create my MVC client project with Indvidual User Accounts? or Should I just create a plain MVC project?
Thanks
Regards,
~ Pat
i am getting a following error can anyone please help me {"Message":"The request is invalid.","ModelState":{"":["Passwords must have at least one non letter or digit character."]}}
ReplyDeleteHi there, try as password: Welcome$01
ReplyDeleteIt should work. There are some rules for the password!
That's why you got the error.
Could you also make a tutorial on how to change it from uning a LocalDB in the project folder to use a ()external MS SQL Server, i cant get to to work. and since you in the previous episode uses a MS SQL Server for the Employee Data why not continue to use that databse.
ReplyDeleteHI Kim , I am trying to create a login page and registration using Asp.net web api and MSSQL. Can you help me with this.
DeleteThank you in advance
Hi.
ReplyDeleteMy app_data folder is empty, and I have inserted a new user successfully. I dont know where is the probem, I followed all the steps of the video.
If you click on 'Show All Files' icon which is available in 'Solution Explorer', then you will see database under App_Data folder. This step has already explained by Kudvenkat sir in this video.
DeleteHey, did everything just as the video, even copied the code to my Register.html source but once the Register button is clicked nothing happens, not even the errors show. Any idea of why is this happening?
ReplyDeleteJust Change the Jquery version of the source code to the jquery version you are currently using. This tutorial is using a old version of jquery thats why you are facing this problem.
Deletehey, can u plzz tell how to change the jquery version to current version
DeleteIt is working successfully using Visual studio express 2015 with Kudvenkat's mentioned bootstrap & jquery files. Thanks.
ReplyDeleteI think that 'modal-sm' class has not required in above Kudvekat sir's code's following line:
ReplyDeleteclass="modal-dialog modal-sm"
Because 'modal-sm' class has not available under 'Content' Folder --> bootstrap.css file.
I need to know, Mr. Venkat. How you were able to create the mdf file? If it was supposed to be available automatically when we created the application, the mdf should be on the App_Data. I apologize if I am wrong, I am new to ASP.NET Web API. I mainly worked on basic C# and SQL so I don't know much about web security.
ReplyDeleteso when you clicked on Register the connection string name=DefaultConnection which was already available in the solution will create that for you. No it was not automatically available.
Deletei have followed your youtube videos and now this doc about registration. But it still says modelstate is invalid. password and confirmpassword do not match. but they do...
ReplyDeleteJust did everything in the video,even if version of jquery is also same. but still nothing is happening when register button is clicked.
ReplyDelete