Suggested Videos
Part 14 - Calling ASP.NET Web API service in a cross domain using jQuery ajax
Part 15 - Cross-origin resource sharing ASP.NET Web API
Part 16 - Enable SSL in Visual Studio Development Server
In this video we will discuss how to enable HTTPS for ASP.NET Web API service. After HTTPS is enabled, if a request is issued using HTTP we want it to be automatically redirected to HTTPS.
This is continuation to Part 16. Please watch Part 16 from ASP.NET Web API tutorial before proceeding.
Two simple steps to enable HTTPS for ASP.NET Web API service.
Step 1 : Right click on the ASP.NET Web API project and add a class file. Name it RequireHttpsAttribute. Copy and paste the following code
Step 2 : Include the following line of code in Register() method of WebApiConfig class in WebApiConfig.cs file in App_Start folder. This adds RequireHttpsAttribute as a filter to the filters collection. So for every request the code in this filter is executed. If the request is issued using HTTP, it will be automatically redirected to HTTPS.
Please note : If you don't want to enable HTTPS for the entire application then don't add RequireHttpsAttribute to the filters collection on the config object in the register method. Simply decorate the controller class or the action method with RequireHttpsAttribute for which you want HTTPS to be enabled. For the rest of the controllers and action methods HTTPS will not be enabled.
Part 14 - Calling ASP.NET Web API service in a cross domain using jQuery ajax
Part 15 - Cross-origin resource sharing ASP.NET Web API
Part 16 - Enable SSL in Visual Studio Development Server
In this video we will discuss how to enable HTTPS for ASP.NET Web API service. After HTTPS is enabled, if a request is issued using HTTP we want it to be automatically redirected to HTTPS.
This is continuation to Part 16. Please watch Part 16 from ASP.NET Web API tutorial before proceeding.
Two simple steps to enable HTTPS for ASP.NET Web API service.
Step 1 : Right click on the ASP.NET Web API project and add a class file. Name it RequireHttpsAttribute. Copy and paste the following code
using System;
using System.Net;
using System.Net.Http;
using System.Text;
using System.Web.Http.Controllers;
using System.Web.Http.Filters;
namespace EmployeeService
{
public class RequireHttpsAttribute : AuthorizationFilterAttribute
{
public override void OnAuthorization(HttpActionContext actionContext)
{
if
(actionContext.Request.RequestUri.Scheme != Uri.UriSchemeHttps)
{
actionContext.Response =
actionContext.Request
.CreateResponse(HttpStatusCode.Found);
actionContext.Response.Content
= new StringContent
("<p>Use https
instead of http</p>", Encoding.UTF8, "text/html");
UriBuilder uriBuilder = new UriBuilder(actionContext.Request.RequestUri);
uriBuilder.Scheme = Uri.UriSchemeHttps;
uriBuilder.Port = 44337;
actionContext.Response.Headers.Location = uriBuilder.Uri;
}
else
{
base.OnAuthorization(actionContext);
}
}
}
}
Step 2 : Include the following line of code in Register() method of WebApiConfig class in WebApiConfig.cs file in App_Start folder. This adds RequireHttpsAttribute as a filter to the filters collection. So for every request the code in this filter is executed. If the request is issued using HTTP, it will be automatically redirected to HTTPS.
config.Filters.Add(new RequireHttpsAttribute());
Please note : If you don't want to enable HTTPS for the entire application then don't add RequireHttpsAttribute to the filters collection on the config object in the register method. Simply decorate the controller class or the action method with RequireHttpsAttribute for which you want HTTPS to be enabled. For the rest of the controllers and action methods HTTPS will not be enabled.
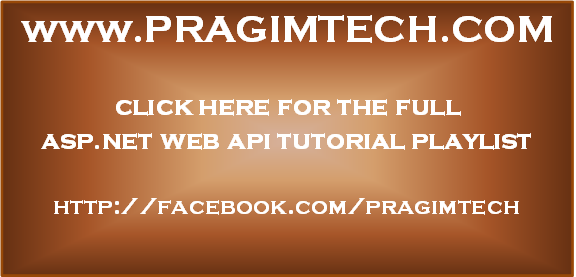
How make Authentication in webApi?
ReplyDeleteHi venkat my onauthorization method been called twice ?
ReplyDeleteYes.
DeleteFor 1st time, it will execute 'if' condition and for 2nd time, it will execute 'else' condition.
First time, if Uri contains http, then 'if' condition will execute and it will converted into https. Hence again same method will call 'else' condition. Because now Uri contains https with different port number.
Don't we have [RequireHttps] attribute directly, as we used in MVC?
ReplyDeleteThanks,
Himanshu Pareek.
RequireHttps is not available in MVC API so devs need to replicate the same functionality just like Venkat did.
DeleteRead More here : https://blogs.msdn.microsoft.com/carlosfigueira/2012/03/09/implementing-requirehttps-with-asp-net-web-api/
Venkat Sir, Can you please add a tag/label for tutorials under one topic so that it's easier to find code used in a previous examples. Switching between Youtube and the blog becomes little hard.
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteNot working. Instead of adding the line config.Filters.Add(new new RequireHttpsAttribute()) to WebApiConfig's Register method, it should be added to FilterConfig.cs in the RegisterGlobalFilters method like this:
ReplyDeletefilters.Add(new RequireHttpsAttribute());
Hi Venkat,
ReplyDeleteCan you explain what is the term "handshake" used for in terms of Web Api