Suggested Videos
Part 1 - What is ASP.NET Web API
In this video we will discuss
1. Creating a new ASP.NET Web API Project
2. Explore and understand the Web API code auto-generated by Visual Studio
For this course, we will be using Visual Studio 2015.
Creating a new ASP.NET Web API Project
1. Open Visual Studio and select File - New - Project
2. In the "New Project" window
Select "Visual C#" under "Installed - Templates"
From the middle pane select, ASP.NET Web Application
Name the project "WebAPIDemo" and click "OK"
3. On the next window, select "Web API" and click "OK"
While creating the Web API project, you may get the following errors
Package Installation Error - Could not add all required packages to the project. The following packages failed to install from 'C:\Program Files (x86)\Microsoft ASP.NET\ASP.NET Web Stack 5\Packages'
Failed to initialize the PowerShell host.
If you do then follow the below steps which may help resolve the issue
1. Close all instances of Visual Studio
2. Open Windows Powershell as an Administrator and execute the following command
Set ExecutionPolicy AllSigned
3. Run Visual Studio 2015 as an Administrator
4. Open Package Manager Console window in Visual Studio. To do this click on Tools - NuGet Package Manager - Package Manager Console
5 In the Package Manager Console, type [R] for Run once and press the Enter key
At this point, you will be able to create a new Web API project.
Now, let us explore and understand the Web API code auto-generated by Visual Studio
1. If you have worked with ASP.NET MVC, then project folder structure should be familiar to you. Notice with in the Controllers folder we have ValuesController which inherits from ApiController class that is present in System.Web.Http namespace. This is different from the MVC controller. The MVC Controller class inherits from the Controller class that is present in System.Web.Mvc namespace. The HomeController class which is an MVC controller inherits from the Controller class.
2. Notice in the ValuesController class we have methods (Get, Put, Post & Delete) that map to the HTTP verbs (GET, PUT, POST, DELETE) respectively. We have 2 overloaded versions of Get() method - One without any parameters and the other with id parameter. Both of these methods respond to the GET http verb depending on whether the id parameter is specified in the URI or not.
3. Now let's look at the default route that is in place for our Web API project. We have the Application_Start() event handler In Global.asax file. This event is raised when the application starts. In the Application_Start() event handler method we have configuration for Filters, Bundles etc. The one that we are interested in is the configuration for our Web API project, which is in WebApiConfig.Register() method. Right click on WebApiConfig.Register and select "Go To Definition" from the context menu. This will take you to the Register() method in the WebApiConfig class. This class is in App_Start folder.
4. In the Register() method we have the default route configured for our Web API project. Web API routes are different from the MVC routes. You can find the MVC routes in RouteConfig.cs file in App_Start folder.
5. The default Web API route starts with the word api and then / and then the name of the controller and another / and an optiontion id parameter.
"api/{controller}/{id}"
6. At this point if we use the following URI in the browser, we get an error - Authorization has been denied for this request.
http://localhost/api/values
7. To get rid of this error, comment Authorize attribute on the ValuesController class. This is related to security which we will discuss in a later video.
8. Now if you visit, http://localhost/api/values, you should see the following XML as the result
9. Let us understand what is going on here. The name of the controller is values. So if we use a URI http://localhost:portnumber/api/values, then the web api is going to look for a controller with name Values + the word controller in your project. So if you have specified values in the URI it is going to look for ValuesController, if you specify Products, then it is going to look for ProductsController. This is all by convention and works this way out of the box.
10. In a real world application this might be the domain name, for example
http://pragimtech.com/api/values
11. The browser is issuing a GET request which maps to the Get() method in the ValuesController class. The GET() in the values controller is returning value1 and value2 which is what we see in the browser.
12. We have another overload of GET() method which takes Id parameter. Remember with the default route, the id parameter is optional. That is the reason we are able to call the GET method with or without the Id parameter. If the id parameter is specified in the URI, then the Get() method with the parameter in values controller is called
13. If a controller with the specified name is not found you will get an error. For example, in your project if you comment the ValuesController class in your project and then use the URI /api/values you will get the following error
No HTTP resource was found that matches the request URI 'http://localhost:15648/api/values'. No type was found that matches the controller named 'values'.
In our next video we will discuss how to perform the rest of the actions PUT, POST and DELETE.
Part 1 - What is ASP.NET Web API
In this video we will discuss
1. Creating a new ASP.NET Web API Project
2. Explore and understand the Web API code auto-generated by Visual Studio
For this course, we will be using Visual Studio 2015.
Creating a new ASP.NET Web API Project
1. Open Visual Studio and select File - New - Project
2. In the "New Project" window
Select "Visual C#" under "Installed - Templates"
From the middle pane select, ASP.NET Web Application
Name the project "WebAPIDemo" and click "OK"

3. On the next window, select "Web API" and click "OK"
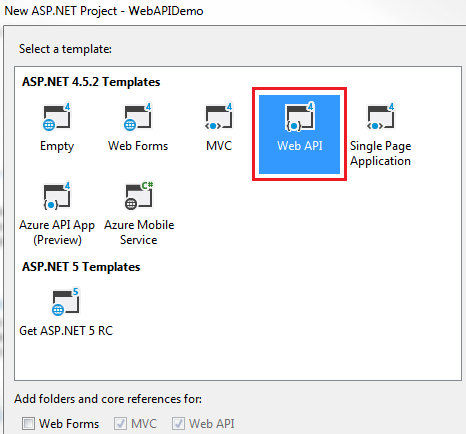
While creating the Web API project, you may get the following errors
Package Installation Error - Could not add all required packages to the project. The following packages failed to install from 'C:\Program Files (x86)\Microsoft ASP.NET\ASP.NET Web Stack 5\Packages'
Failed to initialize the PowerShell host.
If you do then follow the below steps which may help resolve the issue
1. Close all instances of Visual Studio
2. Open Windows Powershell as an Administrator and execute the following command
Set ExecutionPolicy AllSigned
3. Run Visual Studio 2015 as an Administrator
4. Open Package Manager Console window in Visual Studio. To do this click on Tools - NuGet Package Manager - Package Manager Console
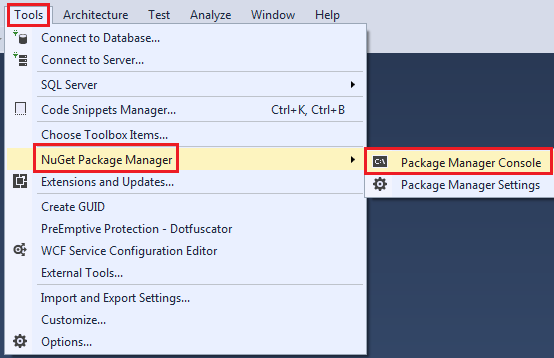
5 In the Package Manager Console, type [R] for Run once and press the Enter key
At this point, you will be able to create a new Web API project.
Now, let us explore and understand the Web API code auto-generated by Visual Studio
1. If you have worked with ASP.NET MVC, then project folder structure should be familiar to you. Notice with in the Controllers folder we have ValuesController which inherits from ApiController class that is present in System.Web.Http namespace. This is different from the MVC controller. The MVC Controller class inherits from the Controller class that is present in System.Web.Mvc namespace. The HomeController class which is an MVC controller inherits from the Controller class.
2. Notice in the ValuesController class we have methods (Get, Put, Post & Delete) that map to the HTTP verbs (GET, PUT, POST, DELETE) respectively. We have 2 overloaded versions of Get() method - One without any parameters and the other with id parameter. Both of these methods respond to the GET http verb depending on whether the id parameter is specified in the URI or not.
3. Now let's look at the default route that is in place for our Web API project. We have the Application_Start() event handler In Global.asax file. This event is raised when the application starts. In the Application_Start() event handler method we have configuration for Filters, Bundles etc. The one that we are interested in is the configuration for our Web API project, which is in WebApiConfig.Register() method. Right click on WebApiConfig.Register and select "Go To Definition" from the context menu. This will take you to the Register() method in the WebApiConfig class. This class is in App_Start folder.
4. In the Register() method we have the default route configured for our Web API project. Web API routes are different from the MVC routes. You can find the MVC routes in RouteConfig.cs file in App_Start folder.
5. The default Web API route starts with the word api and then / and then the name of the controller and another / and an optiontion id parameter.
"api/{controller}/{id}"
6. At this point if we use the following URI in the browser, we get an error - Authorization has been denied for this request.
http://localhost/api/values
7. To get rid of this error, comment Authorize attribute on the ValuesController class. This is related to security which we will discuss in a later video.
8. Now if you visit, http://localhost/api/values, you should see the following XML as the result
<ArrayOfstring xmlns:i="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://schemas.microsoft.com/2003/10/Serialization/Arrays">
<string>value1</string>
<string>value2</string>
</ArrayOfstring>
9. Let us understand what is going on here. The name of the controller is values. So if we use a URI http://localhost:portnumber/api/values, then the web api is going to look for a controller with name Values + the word controller in your project. So if you have specified values in the URI it is going to look for ValuesController, if you specify Products, then it is going to look for ProductsController. This is all by convention and works this way out of the box.
10. In a real world application this might be the domain name, for example
http://pragimtech.com/api/values
11. The browser is issuing a GET request which maps to the Get() method in the ValuesController class. The GET() in the values controller is returning value1 and value2 which is what we see in the browser.
12. We have another overload of GET() method which takes Id parameter. Remember with the default route, the id parameter is optional. That is the reason we are able to call the GET method with or without the Id parameter. If the id parameter is specified in the URI, then the Get() method with the parameter in values controller is called
13. If a controller with the specified name is not found you will get an error. For example, in your project if you comment the ValuesController class in your project and then use the URI /api/values you will get the following error
No HTTP resource was found that matches the request URI 'http://localhost:15648/api/values'. No type was found that matches the controller named 'values'.
In our next video we will discuss how to perform the rest of the actions PUT, POST and DELETE.
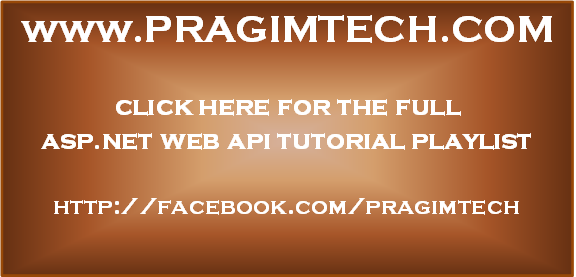
Dear Sir Venkat/Prasad,
ReplyDeleteI just want to thank you for being such an great teacher for all these years. Your video tutorials and written explanations are priceless for both your top expertise and your great effort you are so generously putting into them. You are a great example of an inspiring person.
I wish you the best,
Marinko
The world is still beautiful because of some people like U.
ReplyDeletetrue..you don't know how many lives you have change.
DeleteHello Sir,
ReplyDeleteThanks a lot for your work and efforts in sharing your knowledge and guiding us.I want to know how to obtain output in xml format. Or please provide the link If any video related to this topic already discussed in ur series.
This can achieved through Media Type Formatter. You have to pass the result type in Request header.
ReplyDeletei got my mvc job because of your MVC tutorial
ReplyDeletenow i want to learn JQuery n entityFRamework n webAPI
ReplyDeleteEntity framwork and mvc enables you for great opportunies.... keep learn :)
DeleteHello Sir,
ReplyDeleteWhen to use Asp.net mvc application and Asp.net WebAPI application...which type of scenario?
Hi Zuner
DeleteA .net Web API controller is an MVC controller, which uses HttpMessageResponse as the base type of its response, instead of ActionResponse. They are the same in most other respects. The main difference between the project is the MVC Application project type adds web specific things like default CSS, JavaScript files and other resources needed for a web site, which are not required for an API application.
MVC is used for creating web sites. In this case Controllers usually return a View (i.e. HTML response) to browser requests. Web APIs on the other hand are usually made to be consumed by other applications i.e. HTTP based services on top of the .NET Framework. If you want to allow other applications to access your data / functionality, you can create a Web API to facilitate this access. For example, Facebook has an API in order to allow App developers to access information about users using the App. Web APIs don't have to be for public consumption. You can also create an API to support your own applications. For example, we created a Web API to support the AJAX functionality of our MVC web site.
Thanks
Raj
Hi Zuber,
ReplyDeleteA .net Web API controller is an MVC controller, which uses HttpMessageResponse as the base type of its response, instead of ActionResponse. They are the same in most other respects. The main difference between the project is the MVC Application project type adds web specific things like default CSS, JavaScript files and other resources needed for a web site, which are not required for an API application.
MVC is used for creating web sites. In this case Controllers usually return a View (i.e. HTML response) to browser requests. Web APIs on the other hand are usually made to be consumed by other applications i.e. HTTP based services on top of the .NET Framework. If you want to allow other applications to access your data / functionality, you can create a Web API to facilitate this access. For example, Facebook has an API in order to allow App developers to access information about users using the App. Web APIs don't have to be for public consumption. You can also create an API to support your own applications. For example, we created a Web API to support the AJAX functionality of our MVC web site.
Thanks
Raj
Hi when i have Running Wen API Application then i got below issue how i have resloved.
ReplyDeleteServer Error in '/' Application.
Specified argument was out of the range of valid values.
Parameter name: site
Description: An unhandled exception occurred during the execution of the current web request. Please review the stack trace for more information about the error and where it originated in the code.
Exception Details: System.ArgumentOutOfRangeException: Specified argument was out of the range of valid values.
Parameter name: site
Source Error:
An unhandled exception was generated during the execution of the current web request. Information regarding the origin and location of the exception can be identified using the exception stack trace below.
Stack Trace:
[ArgumentOutOfRangeException: Specified argument was out of the range of valid values.
Parameter name: site]
System.Web.HttpRuntime.HostingInit(HostingEnvironmentFlags hostingFlags, PolicyLevel policyLevel, Exception appDomainCreationException) +560
[HttpException (0x80004005): Specified argument was out of the range of valid values.
Parameter name: site]
System.Web.HttpRuntime.FirstRequestInit(HttpContext context) +772
System.Web.HttpRuntime.EnsureFirstRequestInit(HttpContext context) +95
System.Web.HttpRuntime.ProcessRequestNotificationPrivate(IIS7WorkerRequest wr, HttpContext context) +195
thank you very much for firs time run my solution the result i get in json type not in xml ?
ReplyDeletei am developing an app in cardova using javascript in visual studio 2015.
ReplyDeletenow i have develop already most of it but now facing problem of connecting the registration and login form with database. though i have done this with some websites. but how to work it in cardova app.
someone suggest me about mysql to json converter and calling it through web api. what is all about .
please help me out
Thank you Kud Venkat. You are a life saver and i have watched most of your videos and cleared interviews
ReplyDeleteSir i had done M.SC., Computer science. I also have 6 + years experience in industry. Many trained me during these years. But i still can say you are the best of all the mentors of my lifetime. No one can replace you. you have no idea how many lives you have changed with your knowledge sharing. God bless you and your family.
ReplyDeleteThank you for your videos.
ReplyDeleteI am new to ASP.Net Core MVC and Web API.
I have created a web api project and now want to consume the api from an MVC project.
I have seen samples, partly from your videos, about using jquery in MVC to consume web api. I want to consume web api from server side MVC code.
Do you have ant recommended tutorials for this?
Thanks