Suggested Video Tutorials
Part 38 - Bootstrap accordion
Part 39 - Bootstrap accordion with arrows
Part 40 - Bootstrap modal popup
In this video we will discuss some of the useful methods and events of Bootstrap modal.
In Part 40 of Bootstrap tutorial, we have used the data-attributes to show and hide the modal. We can also manually show and hide the modal using the methods provided by Bootstrap Modal plugin.
Methods of Bootstrap modal plugin
In the example below we have removed all the data attributes (data-toggle, data-target and data-dismiss). Instead, we are using modal('show') and modal('hide') methods to show and hide the login modal.
Events of Bootstrap modal plugin
The following example, handles all the bootstrap modal events
Part 38 - Bootstrap accordion
Part 39 - Bootstrap accordion with arrows
Part 40 - Bootstrap modal popup
In this video we will discuss some of the useful methods and events of Bootstrap modal.
In Part 40 of Bootstrap tutorial, we have used the data-attributes to show and hide the modal. We can also manually show and hide the modal using the methods provided by Bootstrap Modal plugin.

Methods of Bootstrap modal plugin
Method | Purpose |
---|---|
modal('show') | Shows the modal |
modal(hide') | Hides the modal |
In the example below we have removed all the data attributes (data-toggle, data-target and data-dismiss). Instead, we are using modal('show') and modal('hide') methods to show and hide the login modal.
<html>
<head>
<meta name="viewport" content="width=device-width,
initial-scale=1">
<title>Bootstrap tutorial for
begineers</title>
<link href="bootstrap/css/bootstrap.min.css" rel="stylesheet">
</head>
<body>
<br />
<div class="container">
<div class="row">
<div class="col-xs-12">
<button id="btnShowModal" type="button"
class="btn btn-sm btn-default
pull-right">
Login
</button>
<div class="modal fade" tabindex="-1" id="loginModal"
data-keyboard="false" data-backdrop="static">
<div class="modal-dialog
modal-sm">
<div class="modal-content">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal">
×
</button>
<h4 class="modal-title">Login</h4>
</div>
<div class="modal-body">
<form>
<div class="form-group">
<label for="inputUserName">Username</label>
<input class="form-control" type="text"
placeholder="Login Username" id="inputUserName" />
</div>
<div class="form-group">
<label for="inputPassword">Password</label>
<input class="form-control" placeholder="Login Password"
type="password" id="inputPassword" />
</div>
</form>
</div>
<div class="modal-footer">
<button type="submit" class="btn
btn-primary">Login</button>
<button type="button" id="btnHideModal" class="btn
btn-primary">
Close
</button>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.3/jquery.min.js">
</script>
<script src="bootstrap/js/bootstrap.min.js"></script>
<script type="text/javascript">
$(document).ready(function () {
$("#btnShowModal").click(function () {
$("#loginModal").modal('show');
});
$("#btnHideModal").click(function () {
$("#loginModal").modal('hide');
});
});
</script>
</body>
</html>
Events of Bootstrap modal plugin
Event | Description |
---|---|
show.bs.modal | Fired immediately after modal('show') method is called |
shown.bs.modal | Fired after the modal is completely shown |
hide.bs.modal | Fired immediately after modal('hide') method is called |
hidden.bs.modal | Fired after the modal is completely hidden |
The following example, handles all the bootstrap modal events
<html>
<head>
<meta name="viewport" content="width=device-width,
initial-scale=1">
<title>Bootstrap tutorial for
begineers</title>
<link href="bootstrap/css/bootstrap.min.css" rel="stylesheet">
</head>
<body>
<br />
<div class="container">
<div class="row">
<div class="col-xs-12">
<button id="btnShowModal" type="button"
class="btn btn-sm btn-default
pull-right">
Login
</button>
<div class="modal fade" tabindex="-1" id="loginModal"
data-keyboard="false" data-backdrop="static">
<div class="modal-dialog
modal-sm">
<div class="modal-content">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal">
×
</button>
<h4 class="modal-title">Login</h4>
</div>
<div class="modal-body">
<form>
<div class="form-group">
<label for="inputUserName">Username</label>
<input class="form-control" placeholder="Login Username"
type="text" id="inputUserName" />
</div>
<div class="form-group">
<label for="inputPassword">Password</label>
<input class="form-control" placeholder="Login Password"
type="password" id="inputPassword" />
</div>
</form>
</div>
<div class="modal-footer">
<button type="submit" class="btn
btn-primary">Login</button>
<button type="button" id="btnHideModal" class="btn
btn-primary">
Close
</button>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.3/jquery.min.js">
</script>
<script src="bootstrap/js/bootstrap.min.js"></script>
<script type="text/javascript">
$(document).ready(function () {
$("#btnShowModal").click(function () {
$("#loginModal").modal('show');
});
$("#btnHideModal").click(function () {
$("#loginModal").modal('hide');
});
$('#loginModal').on('show.bs.modal', function () {
alert('Modal is about to be
displayed');
});
$('#loginModal').on('shown.bs.modal', function () {
alert('Modal is displayed');
});
$('#loginModal').on('hide.bs.modal', function () {
alert('Modal is about to be
hidden');
});
$('#loginModal').on('hidden.bs.modal', function () {
alert('Modal is hidden');
});
});
</script>
</body>
</html>
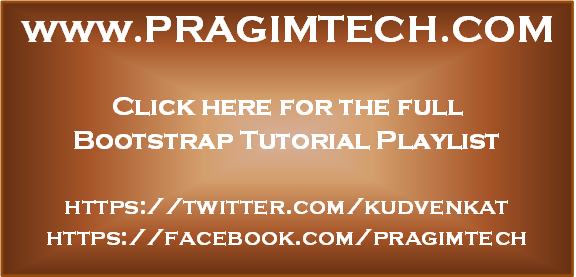
No comments:
Post a Comment
It would be great if you can help share these free resources