Suggested Videos
Part 42 - AngularJS ui-router tutorial
Part 43 - AngularJS ui-router configuring states
Part 44 - AngularJS ui router parameters
In this video we will discuss
1. How to use optional URL parameters with ui router
2. Programmatically activating a state using $state service go method
With ngRoute module to make a parameter optional we include a question mark (?) at the end of the parameter name. With ui-router the parameters are optional by default, so there is nothing special that we have to do. Let us understand ui-router optional parameters with an example. Here is what we want to do.
On the list of students page, we want to search employees by name. For example if we type "Ma" and click search button, on the subsequent page we want to display all the student names that start with "Ma".
The name parameter value "ma" should be passed in the URL as shown below
http://localhost:51983/#/studentsSearch/ma
On the other hand, if we do not enter any name in the search text box and click search button, on the subsequent page we want to display all the student names. In this case the name parameter value should not be passed in the URL. The URL should be as shown below.
http://localhost:51983/#/studentsSearch/
So in summary, the name parameter should be optional. Here are the steps.
Step 1 : Define studentsSearch state. Notice in the url property we have included name parameter. Notice, we have not done anything special to make it optional. By default UI router parameters are optional.
Step 2 : The studentSearch() function that gets called when the search button is clicked is in studentsController function. Notice we are using $state service go() method to activate studentsSearch state. We are also passing a value for the name parameter. If there is something typed in the search text box, the value is passed in the URL to the state, otherwise nothing is passed. So name URL parameter is effectively optional.
Step 3 : Modify studentsSearchController function to retrieve name URL parameter value. Notice we are using $stateParams service to retrieve name URL parameter value.
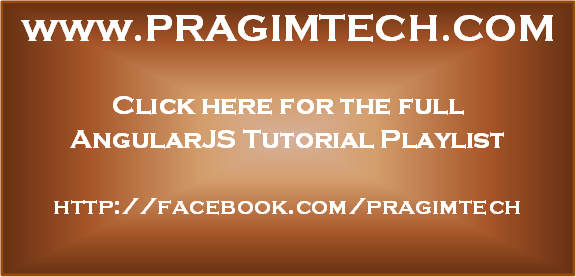
Part 42 - AngularJS ui-router tutorial
Part 43 - AngularJS ui-router configuring states
Part 44 - AngularJS ui router parameters
In this video we will discuss
1. How to use optional URL parameters with ui router
2. Programmatically activating a state using $state service go method
With ngRoute module to make a parameter optional we include a question mark (?) at the end of the parameter name. With ui-router the parameters are optional by default, so there is nothing special that we have to do. Let us understand ui-router optional parameters with an example. Here is what we want to do.
On the list of students page, we want to search employees by name. For example if we type "Ma" and click search button, on the subsequent page we want to display all the student names that start with "Ma".

The name parameter value "ma" should be passed in the URL as shown below
http://localhost:51983/#/studentsSearch/ma
On the other hand, if we do not enter any name in the search text box and click search button, on the subsequent page we want to display all the student names. In this case the name parameter value should not be passed in the URL. The URL should be as shown below.
http://localhost:51983/#/studentsSearch/
So in summary, the name parameter should be optional. Here are the steps.
Step 1 : Define studentsSearch state. Notice in the url property we have included name parameter. Notice, we have not done anything special to make it optional. By default UI router parameters are optional.
.state("studentsSearch", {
url:"/studentsSearch/:name",
templateUrl: "Templates/studentsSearch.html",
controller: "studentsSearchController",
controllerAs: "studentsSearchCtrl"
})
Step 2 : The studentSearch() function that gets called when the search button is clicked is in studentsController function. Notice we are using $state service go() method to activate studentsSearch state. We are also passing a value for the name parameter. If there is something typed in the search text box, the value is passed in the URL to the state, otherwise nothing is passed. So name URL parameter is effectively optional.
.controller("studentsController", function (studentslist, $state,
$location) {
var vm = this;
vm.studentSearch = function
() {
$state.go("studentsSearch", { name: vm.name });
}
vm.reloadData = function () {
$state.reload();
}
vm.students = studentslist;
})
Step 3 : Modify studentsSearchController function to retrieve name URL parameter value. Notice we are using $stateParams service to retrieve name URL parameter value.
.controller("studentsSearchController", function ($http, $stateParams) {
var vm = this;
if ($stateParams.name) {
$http({
url: "StudentService.asmx/GetStudentsByName",
method: "get",
params: { name: $stateParams.name }
}).then(function (response) {
vm.students = response.data;
})
}
else {
$http.get("StudentService.asmx/GetAllStudents")
.then(function (response) {
vm.students =
response.data;
})
}
})
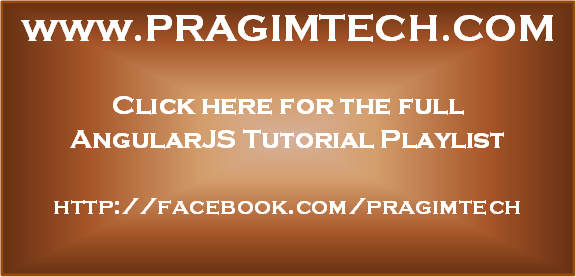
Excellent AngularJs 1.5 tutorial. Many Thanks for keeping this valuable resources for free.
ReplyDeleteHi Sir,
ReplyDeleteI have implemented the same way you have. Still I'm not able to fetch all the Students when I'm clicking search button without entering any value in text box.
I'm getting below error messages on console.
stateService.ts:533 Transition Rejection($id: 2 type: 6, message: The transition errored, detail: Error: Transition Rejection($id: 1 type: 4, message: This transition is invalid, detail: The following parameter values are not valid for state 'studentsSearch': [name:undefined]))
$defaultErrorHandler @ stateService.ts:533
(anonymous) @ stateService.ts:375
processQueue @ angular.js:17945
(anonymous) @ angular.js:17993
$digest @ angular.js:19112
$apply @ angular.js:19500
(anonymous) @ angular.js:28955
defaultHandlerWrapper @ angular.js:3824
eventHandler @ angular.js:3812
stateService.ts:534 Error: Transition Rejection($id: 1 type: 4, message: This transition is invalid, detail: The following parameter values are not valid for state 'studentsSearch': [name:undefined])
at invalidTransitionHook (invalidTransition.ts:15)
at invokeCallback (transitionHook.ts:143)
at TransitionHook.invokeHook (transitionHook.ts:152)
at Function.TransitionHook.invokeHooks (transitionHook.ts:98)
at Transition.run (transition.ts:814)
at StateService.transitionTo (stateService.ts:381)
at StateService.go (stateService.ts:272)
at Object.vm.searchStudent (DemoScript.js:50)
at fn (eval at compile (angular.js:16418), :2:199)
at callback (angular.js:28951)
When I'm entering anything in textbox it's redirecting to the search result correct way. Only the problem occurring when i'm not entering.
Please advise the changes.
Regards,
Ashish Swami