Suggested Videos
Part 2 - Angular modules and controllers
Part 3 - Controllers in AngularJS
Part 4 - AngularJS ng-src directive
In this video we will discuss, Two way data binding in AngularJS. Along the way we also discuss one of the very useful directive in angular ng-model.
When the model changes the view is automatically updated. This is achieved using the data binding expression in the view.
Script.js : The code in the controller attaches message property to the scope which is the model.
HtmlPage1.html : Whenever the message property value changes, the data binding expression in the view updates the view automatically.
How about the other way round. How to keep the model up to date when the view changes. That's exactly is the purpose of ng-model directive.
In the html below, notice the input element is decorated with ng-model directive. This ensures that whenever the value in the textbox is changed, angular will automatically update the message property of the $scope object. This means the ng-model directive automatically takes the form values and updates the model. The binding expression does the opposite, i.e whenever the model changes the view is automatically updated.
Because of the two way data binding provided by angular, as you type in the textbox, the value is immediately displayed on the view just below the textbox. This two way binding feature provided by angular, eliminates the need to write any custom code to move data from the model to the view or from the view to the model.
ng-model directive can be used with the following 3 html elements
Script.js code : In the following example, the model is employee which is a complex object with properties like firstName, lastName and gender.
HtmlPage1.html : When the view loads, the model data is display in both, the textbox and td elements on the page. As you start to type in any of the textboxes, the respective employee model object property is updated, and the change is immediately reflected in the respective td element.
Part 2 - Angular modules and controllers
Part 3 - Controllers in AngularJS
Part 4 - AngularJS ng-src directive
In this video we will discuss, Two way data binding in AngularJS. Along the way we also discuss one of the very useful directive in angular ng-model.

When the model changes the view is automatically updated. This is achieved using the data binding expression in the view.
Script.js : The code in the controller attaches message property to the scope which is the model.
var app = angular
.module("myModule", [])
.controller("myController",
function
($scope) {
$scope.message = "Hello Angular"
});
HtmlPage1.html : Whenever the message property value changes, the data binding expression in the view updates the view automatically.
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<title></title>
<script src="Scripts/angular.min.js"></script>
<script src="Scripts/Script.js"></script>
</head>
<body ng-app="myModule">
<div ng-controller="myController">
{{ message }}
</div>
</body>
</html>
How about the other way round. How to keep the model up to date when the view changes. That's exactly is the purpose of ng-model directive.
In the html below, notice the input element is decorated with ng-model directive. This ensures that whenever the value in the textbox is changed, angular will automatically update the message property of the $scope object. This means the ng-model directive automatically takes the form values and updates the model. The binding expression does the opposite, i.e whenever the model changes the view is automatically updated.
Because of the two way data binding provided by angular, as you type in the textbox, the value is immediately displayed on the view just below the textbox. This two way binding feature provided by angular, eliminates the need to write any custom code to move data from the model to the view or from the view to the model.
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<title></title>
<script src="Scripts/angular.min.js"></script>
<script src="Scripts/Script.js"></script>
</head>
<body ng-app="myModule">
<div ng-controller="myController">
<input type="text" placeholder="Type your message here" ng-model="message" />
<br /><br />
{{ message }}
</div>
</body>
</html>
ng-model directive can be used with the following 3 html elements
- input
- select
- textarea

Script.js code : In the following example, the model is employee which is a complex object with properties like firstName, lastName and gender.
var app = angular
.module("myModule", [])
.controller("myController",
function
($scope) {
var employee = {
firstName: "Ben",
lastName: "Hastings",
gender: "Male"
};
$scope.employee = employee;
});
HtmlPage1.html : When the view loads, the model data is display in both, the textbox and td elements on the page. As you start to type in any of the textboxes, the respective employee model object property is updated, and the change is immediately reflected in the respective td element.
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<title></title>
<script src="Scripts/angular.min.js"></script>
<script src="Scripts/Script.js"></script>
</head>
<body ng-app="myModule">
<div ng-controller="myController">
<table>
<tr>
<td>
First Name
</td>
<td>
<input type="text" placeholder="Firstname"
ng-model="employee.firstName" />
</td>
</tr>
<tr>
<td>
Last Name
</td>
<td>
<input type="text" placeholder="Lastname"
ng-model="employee.lastName" />
</td>
</tr>
<tr>
<td>
Gender
</td>
<td>
<input type="text" placeholder="Gender"
ng-model="employee.gender" />
</td>
</tr>
</table>
<br />
<table>
<tr>
<td>
First Name
</td>
<td>
{{ employee.firstName }}
</td>
</tr>
<tr>
<td>
Last Name
</td>
<td>
{{ employee.lastName }}
</td>
</tr>
<tr>
<td>
Gender
</td>
<td>
{{ employee.gender }}
</td>
</tr>
</table>
</div>
</body>
</html>
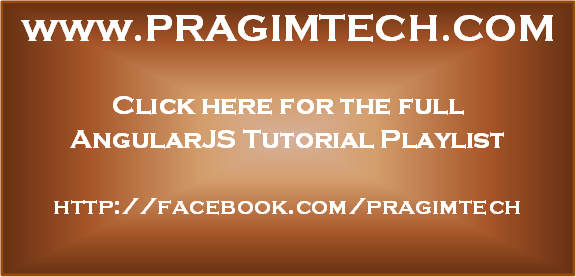
hi venkat your teaching skills are excellent and the way your explaining the concepts are very well...:)
ReplyDeleteGreat Job.......
hi venkat your really an awesome guy... the way your explaining is awestruck... no one can beat you in my point of view... keep going on...
ReplyDeletethank you soooo much for everything, You are the best best best teacher in the world... God bless you... may god will give you all what you need...
ReplyDeleteThank you so much for sharing the knowledge here. I don't have words for your great work.
ReplyDeleteThanks so much for Knowledge sharing, Your Explanation is so good.
ReplyDeleteHi Venkat,
ReplyDeleteCan you please explain objects like $watch, $digest, $apply,$q..etc that is objects which are frequently used in the application.
When you have "...ng-model="message", does that correspond to the scope property: $Scope.message?
ReplyDeleteHi venkat,Your teaching skills are excellent man keep it up :) :)
ReplyDeleteand one request need one hands on project using angular as FE and C# as BE.
Hii venkat sir, it's a really good article
ReplyDeleteHi Venkat,
ReplyDeleteThanks for your wonderful!!!!! sessions :) :)
I really like your way of presentation written and video format.
Excellent!!!