Suggested Videos
Part 79 - jquery multiple sliders on page
Part 80 - jquery range slider
Part 81 - jquery tooltip widget
In this video, we will discuss how to retrieve tooltip text from database and display using jquery tooltip widget.
We will be using the following database table for the purpose of this demo
The tooltip text should be retrieved from the database using ajax and display it using jquery tooltip widget
Step 1 : Create SQL Server table and insert tooltip data
Step 2 : Create a stored procedure to retrieve tooltip text by fieldname
Step 3 : Create new asp.net web application project. Name it Demo.
Step 4 : Include a connection string in the web.config file to your database.
Step 5 : Add a class file to the project. Name it Tooltip.cs. Copy and paste the following code.
Step 6 : Add a new WebService (ASMX). Name it TooltipService.asmx. Copy and paste the following code.
Step 7 : Add a WebForm to the ASP.NET project. Copy and paste the following HTML and jQuery code
Part 79 - jquery multiple sliders on page
Part 80 - jquery range slider
Part 81 - jquery tooltip widget
In this video, we will discuss how to retrieve tooltip text from database and display using jquery tooltip widget.
We will be using the following database table for the purpose of this demo
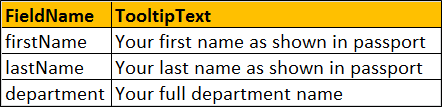
The tooltip text should be retrieved from the database using ajax and display it using jquery tooltip widget
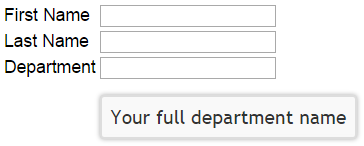
Step 1 : Create SQL Server table and insert tooltip data
Create table tblTooltip
(
FieldName nvarchar(50),
TooltipText nvarchar(1000)
)
Go
Insert into tblTooltip values ('firstName','Your first name as shown in
passport')
Insert into tblTooltip values ('lastName','Your last name as shown in
passport')
Insert into tblTooltip values ('department','Your full department name')
Go
Step 2 : Create a stored procedure to retrieve tooltip text by fieldname
Create proc spGetTooltip
@FieldName nvarchar(50)
as
Begin
Select *
from tblTooltip
where FieldName = @FieldName
End
Step 3 : Create new asp.net web application project. Name it Demo.
Step 4 : Include a connection string in the web.config file to your database.
<add name="DBCS"
connectionString="server=.;database=SampleDB;integrated security=SSPI"/>
Step 5 : Add a class file to the project. Name it Tooltip.cs. Copy and paste the following code.
namespace Demo
{
public class Tooltip
{
public string FieldName { get; set; }
public string TooltipText { get; set; }
}
}
Step 6 : Add a new WebService (ASMX). Name it TooltipService.asmx. Copy and paste the following code.
using System.Configuration;
using System.Data;
using System.Data.SqlClient;
using System.Web.Script.Serialization;
using System.Web.Services;
namespace Demo
{
[WebService(Namespace = "http://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
[System.ComponentModel.ToolboxItem(false)]
[System.Web.Script.Services.ScriptService]
public class TooltipService : System.Web.Services.WebService
{
[WebMethod]
public void GetTooltip(string fieldName)
{
string cs = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
Tooltip tooltip = new Tooltip();
using (SqlConnection con = new SqlConnection(cs))
{
SqlCommand cmd = new SqlCommand("spGetTooltip", con);
cmd.CommandType = CommandType.StoredProcedure;
SqlParameter parameter = new SqlParameter();
parameter.ParameterName = "@FieldName";
parameter.Value = fieldName;
cmd.Parameters.Add(parameter);
con.Open();
SqlDataReader rdr = cmd.ExecuteReader();
while (rdr.Read())
{
tooltip.FieldName = rdr["FieldName"].ToString();
tooltip.TooltipText = rdr["TooltipText"].ToString();
}
}
JavaScriptSerializer js = new JavaScriptSerializer();
Context.Response.Write(js.Serialize(tooltip));
}
}
}
Step 7 : Add a WebForm to the ASP.NET project. Copy and paste the following HTML and jQuery code
<%@ Page Language="C#" AutoEventWireup="true"
CodeBehind="WebForm1.aspx.cs" Inherits="Demo.WebForm1" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<script src="jquery-1.11.2.js"></script>
<script src="jquery-ui.js"></script>
<link href="jquery-ui.css" rel="stylesheet" />
<script type="text/javascript">
$(document).ready(function () {
$('.displayTooltip').tooltip({
content: getTooltip
});
function getTooltip() {
var returnValue = '';
$.ajax({
url: 'TooltipService.asmx/GetTooltip',
method: 'post',
data: { fieldName: $(this).attr('id') },
dataType: 'json',
async: false,
success: function (data) {
returnValue =
data.TooltipText;
}
});
return returnValue;
}
});
</script>
</head>
<body style="font-family:
Arial">
<form id="form1" runat="server">
<table>
<tr>
<td>First Name</td>
<td>
<input id="firstName" class="displayTooltip" title="" type="text" />
</td>
</tr>
<tr>
<td>Last Name</td>
<td>
<input id="lastName" class="displayTooltip" title="" type="text" />
</td>
</tr>
<tr>
<td>Department</td>
<td>
<input id="department" class="displayTooltip" title="" type="text" />
</td>
</tr>
</table>
</form>
</body>
</html>
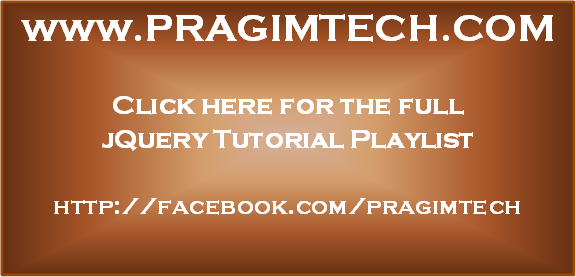
No comments:
Post a Comment
It would be great if you can help share these free resources