Suggested Videos
Part 90 - jquery dialog save to database
Part 91 - jquery button widget
Part 92 - jquery draggable widget
In this video we will discuss
1. How to keep draggable element always on top of other draggable elements on the page
2. How to bring the element on top of other draggable elements on the page as soon as the mouse down event is triggered
Consider the following code
HTML
CSS
jQuery
All the div elements on this page are draggable. The problem here is that, when you drag Red Div it does not stay on top of other draggable div elements on the page. Green Div on the other hand stays on top of Red Div but stays below Blue and Red Div elements. This is the default behaviour.
To bring a Div Element that is being dragged on top of other draggable div's on the page, use stack option. The way this works is jQuery sets the z-index of the element that is being dragged to higher than the z-index of any other draggable div element on the page. You can see the value of the z-index in browser developer tools.
However, if you simply click on a Div element without dragging then the element is not brought on to the top. Here is the code to bring the element on top as soon as mousedown event is triggered. All this function does is change the z-index of the div element to a value greater than the z-index of any other div element.
Part 90 - jquery dialog save to database
Part 91 - jquery button widget
Part 92 - jquery draggable widget
In this video we will discuss
1. How to keep draggable element always on top of other draggable elements on the page
2. How to bring the element on top of other draggable elements on the page as soon as the mouse down event is triggered
Consider the following code
HTML
<div id="redDiv" class="divClass" style="background-color:
red">
Red Div
</div>
<div id="greeDiv" class="divClass" style="background-color:
green">
Green Div
</div>
<div id="blueDiv" class="divClass" style="background-color:
blue">
Blue Div
</div>
<div id="brownDiv" class="divClass" style="background-color:
brown">
Brown Div
</div>
CSS
.divClass {
font-family: Arial;
height: 200px;
width: 200px;
color: white;
display: table-cell;
vertical-align: middle;
text-align: center;
z-index: 0;
}
jQuery
$(document).ready(function () {
$('.divClass').draggable();
});
All the div elements on this page are draggable. The problem here is that, when you drag Red Div it does not stay on top of other draggable div elements on the page. Green Div on the other hand stays on top of Red Div but stays below Blue and Red Div elements. This is the default behaviour.

To bring a Div Element that is being dragged on top of other draggable div's on the page, use stack option. The way this works is jQuery sets the z-index of the element that is being dragged to higher than the z-index of any other draggable div element on the page. You can see the value of the z-index in browser developer tools.
$(document).ready(function () {
$('.divClass').draggable({
stack: '.divClass'
});
});
However, if you simply click on a Div element without dragging then the element is not brought on to the top. Here is the code to bring the element on top as soon as mousedown event is triggered. All this function does is change the z-index of the div element to a value greater than the z-index of any other div element.
$(document).ready(function () {
$('.divClass').draggable();
$('.divClass').mousedown(function () {
var maxZindex = 0;
$(this).siblings('.divClass').each(function () {
var currentZindex = Number($(this).css('z-index'));
maxZindex = currentZindex >
maxZindex ? currentZindex : maxZindex;
});
$(this).css('z-index', maxZindex + 1);
});
});
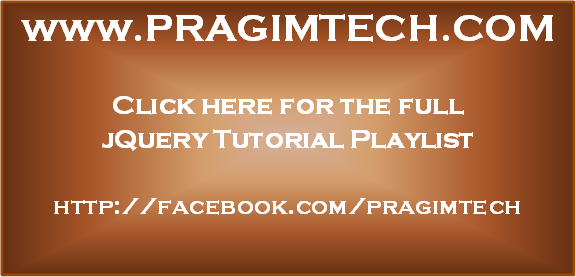
div is displaying on the screen but we are unable to move the div why . i also imported
ReplyDelete