Suggested Videos
Part 54 - jquery ajax load aspx page
Part 55 - jquery load callback function
Part 56 - jquery ajax get function
In this video we will discuss how to load JSON data from the server using jQuery get function. This is continuation to Part 56. Please watch Part 56 before proceeding.
Syntax
$.get( url [, data ] [, success ] [, dataType ] )
dataType parameter specifies the type of data expected from the server. The dataType can be xml, json, script, or html. By default jQuery makes an intelligent guess.
The following steps modify the example we worked with in Part 56, so that HtmlPage1.html will be able to retrieve and display JSON data from the server.
Step 1 : Modify GetHelpText.aspx to return JSON data. Remove the following div element from GetHelpText.aspx.
Step 2 : Modify the code in GetHelpText.aspx.cs as shown below.
Step 3 : Modify the jQuery code in HtmlPage1.html as shown below
Part 54 - jquery ajax load aspx page
Part 55 - jquery load callback function
Part 56 - jquery ajax get function
In this video we will discuss how to load JSON data from the server using jQuery get function. This is continuation to Part 56. Please watch Part 56 before proceeding.
Syntax
$.get( url [, data ] [, success ] [, dataType ] )
dataType parameter specifies the type of data expected from the server. The dataType can be xml, json, script, or html. By default jQuery makes an intelligent guess.
The following steps modify the example we worked with in Part 56, so that HtmlPage1.html will be able to retrieve and display JSON data from the server.
Step 1 : Modify GetHelpText.aspx to return JSON data. Remove the following div element from GetHelpText.aspx.
<div id="divResult" runat="server"></div>
Step 2 : Modify the code in GetHelpText.aspx.cs as shown below.
using System;
using System.Configuration;
using System.Data;
using System.Data.SqlClient;
using System.Web.Script.Serialization;
namespace Demo
{
public partial class GetHelpText : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
JavaScriptSerializer js = new JavaScriptSerializer();
string JSONString = js.Serialize(GetHelpTextByKey(Request["HelpTextKey"]));
Response.Write(JSONString);
}
private HelpText GetHelpTextByKey(string key)
{
HelpText helpText = new HelpText();
string cs = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(cs))
{
SqlCommand cmd = new SqlCommand("spGetHelpTextByKey", con);
cmd.CommandType = CommandType.StoredProcedure;
SqlParameter parameter = new SqlParameter("@HelpTextKey", key);
cmd.Parameters.Add(parameter);
con.Open();
helpText.Text =
cmd.ExecuteScalar().ToString();
helpText.Key = key;
}
return helpText;
}
}
public class HelpText
{
public string Key { get; set; }
public string Text { get; set; }
}
}
Step 3 : Modify the jQuery code in HtmlPage1.html as shown below
$(document).ready(function () {
var textBoxes = $('input[type="text"]');
textBoxes.focus(function () {
var helpDiv = $(this).attr('id');
$.get('GetHelpText.aspx', { HelpTextKey: helpDiv }, function (response) {
$('#' + helpDiv + 'HelpDiv').html(response.Text);
}, 'json');
});
textBoxes.blur(function () {
var helpDiv = $(this).attr('id') + 'HelpDiv';
$('#' + helpDiv).html('');
});
});
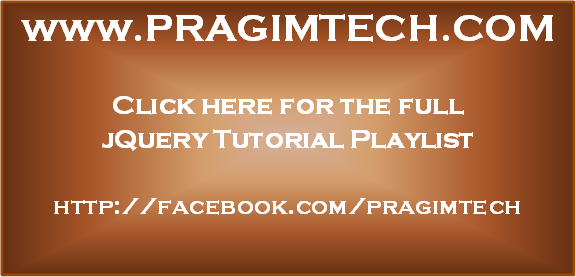
Every thing works fine except the last step when adding the fourth parameter [datatype] 'json' of the $.get function and changing the argument of the html to response.Text.
ReplyDeleteAll the preceding steps worked fine and the texts are displayed next to the input boxes.
Please note i am testing with visual studio 2010 sp1
I found the problem......you need to delete the div section from the aspx webform
ReplyDelete