Suggested Videos
Part 58 - jquery ajax get xml data
Part 59 - jquery make a post request
Part 60 - jquery ajax method
In this video we will discuss how to call asp.net webservice using jQuery AJAX.
We want to retrieve data from the following database table tblEmployee using asp.net web service and jQuery AJAX
The retrieved data should be displayed as shown below
Step 1 : Create SQL Server table and insert employee data
Step 2 : Create a stored procedure to retrieve employee data by ID
Step 3 : Create new asp.net web application project. Name it Demo.
Step 4 : Include a connection string in the web.config file to your database.
Step 5 : Add a class file to the project. Name it Employee.cs. Copy and paste the following code.
Step 6 : Add a new WebService (ASMX). Name it EmployeeService.asmx. Copy and paste the following code.
Step 7 : Add an HTML page to the ASP.NET project. Copy and paste the following HTML and jQuery code
In our next video we will discuss how to call an asp.net web service that returns JSON data using jQuery AJAX.
Part 58 - jquery ajax get xml data
Part 59 - jquery make a post request
Part 60 - jquery ajax method
In this video we will discuss how to call asp.net webservice using jQuery AJAX.
We want to retrieve data from the following database table tblEmployee using asp.net web service and jQuery AJAX
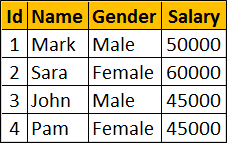
The retrieved data should be displayed as shown below
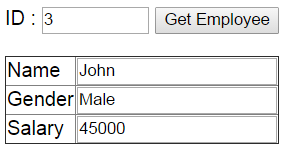
Step 1 : Create SQL Server table and insert employee data
Create table tblEmployee
(
Id int primary
key identity,
Name nvarchar(50),
Gender nvarchar(10),
Salary int
)
GO
Insert into tblEmployee values ('Mark', 'Male', 50000)
Insert into tblEmployee values ('Sara', 'Female', 60000)
Insert into tblEmployee values ('John', 'Male', 45000)
Insert into tblEmployee values ('Pam', 'Female', 45000)
GO
Step 2 : Create a stored procedure to retrieve employee data by ID
Create procedure spGetEmployeeById
@Id int
as
Begin
Select ID, Name, Gender, Salary
from tblEmployee
where ID = @Id
End
Step 3 : Create new asp.net web application project. Name it Demo.
Step 4 : Include a connection string in the web.config file to your database.
<add name="DBCS"
connectionString="server=.;database=SampleDB;integrated security=SSPI" />
Step 5 : Add a class file to the project. Name it Employee.cs. Copy and paste the following code.
namespace Demo
{
public class Employee
{
public int ID { get; set; }
public string Name { get; set; }
public string Gender { get; set; }
public int Salary { get; set; }
}
}
Step 6 : Add a new WebService (ASMX). Name it EmployeeService.asmx. Copy and paste the following code.
using System;
using System.Configuration;
using System.Data;
using System.Data.SqlClient;
using System.Web.Services;
namespace Demo
{
[WebService(Namespace = "http://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
[System.ComponentModel.ToolboxItem(false)]
[System.Web.Script.Services.ScriptService]
public class EmployeeService : System.Web.Services.WebService
{
[WebMethod]
public Employee GetEmployeeById(int employeeId)
{
Employee employee = new Employee();
string cs = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(cs))
{
SqlCommand cmd = new SqlCommand("spGetEmployeeById", con);
cmd.CommandType = CommandType.StoredProcedure;
SqlParameter parameter = new SqlParameter();
parameter.ParameterName = "@Id";
parameter.Value = employeeId;
cmd.Parameters.Add(parameter);
con.Open();
SqlDataReader rdr = cmd.ExecuteReader();
while (rdr.Read())
{
employee.ID = Convert.ToInt32(rdr["Id"]);
employee.Name = rdr["Name"].ToString();
employee.Gender = rdr["Gender"].ToString();
employee.Salary = Convert.ToInt32(rdr["Salary"]);
}
}
return employee;
}
}
}
Step 7 : Add an HTML page to the ASP.NET project. Copy and paste the following HTML and jQuery code
<html>
<head>
<script src="jquery-1.11.2.js"></script>
<script type="text/javascript">
$(document).ready(function () {
$('#btnGetEmployee').click(function () {
var empId = $('#txtId').val();
$.ajax({
url: 'EmployeeService.asmx/GetEmployeeById',
data: { employeeId: empId
},
method: 'post',
dataType: 'xml',
success: function (data) {
var jQueryXml = $(data);
$('#txtName').val(jQueryXml.find('Name').text());
$('#txtGender').val(jQueryXml.find('Gender').text());
$('#txtSalary').val(jQueryXml.find('Salary').text());
},
error: function (err) {
alert(err);
}
});
});
});
</script>
</head>
<body style="font-family:Arial">
ID : <input id="txtId" type="text" style="width:100px" />
<input type="button" id="btnGetEmployee" value="Get
Employee" />
<br /><br />
<table border="1" style="border-collapse:collapse">
<tr>
<td>Name</td>
<td><input id="txtName" type="text" /></td>
</tr>
<tr>
<td>Gender</td>
<td><input id="txtGender" type="text" /></td>
</tr>
<tr>
<td>Salary</td>
<td><input id="txtSalary" type="text" /></td>
</tr>
</table>
</body>
</html>
In our next video we will discuss how to call an asp.net web service that returns JSON data using jQuery AJAX.
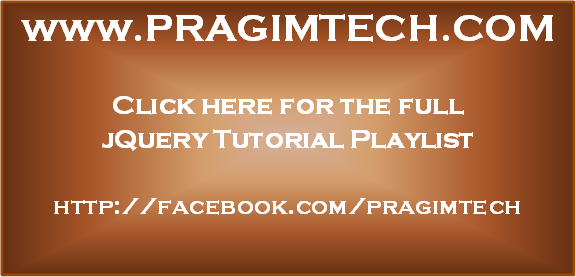
hi venkat...i wrote thee same code...its not giving me expected result...is there some other setting too? coz i dont think there is any issue with the code.
ReplyDeleteIt only shows in alert [object object]
u r using webpage.
ReplyDeleteinstated of webpage use html page u will get correct data
Delete$(document).ready(function () {
$('#btnGetEmployee').click(function () {
var empId = $('#txtId').val();
$.ajax({
url: 'http://localhost:54085/EmployeeService.asmx/GetEmployeeById',
data: { employeeId: empId },
dataType: "json",
method: 'post',
success: function (data) {
var obj = $.parseJSON(data);
for (var i = 0; i < obj.length; i++) {
alert(obj[i].Name);
}
//$('#txtName').val(data.Name);
//$('#txtGender').val(data.Gender);
// $('#txtSalary').val(data.Salary);
},
error: function (err) {
alert(err);
}
});
});
});
I have tried like this it is not working (in html page ) please help me
still getting same error [object][object] in alertbox
ReplyDeletedid u find a solution to object object problem?
Deleteasmx works just fine and it brings xml data from the server. but, the html page doesn't fetch any data. Can I get help in the last step? I get no error nor fail when build and run. here is the output
ReplyDelete========== Build: 1 succeeded, 0 failed, 0 up-to-date, 0 skipped ==========
Hello sir, thank you for this very clear and easy to follow explanation. I have one question about contentType/dataType parameter of ajax. My intellisense shows me contentType, and when I set it to "xml", app doesn't work, error occurs. But if I put dataType:"xml" then everything work, even intellisense is not offering dataType. Why is this?
ReplyDeleteI am UI developer .. Should I have to learn Web Service (ASMX) configuration to post and retrieve the data for database????
ReplyDelete