Suggested Videos
Part 70 - Load data on page scroll using jquery
Part 71 - Calling live json web service using jquery ajax
Part 72 - Autocomplete textbox using jquery in asp.net
In this video we will discuss, how to implement autocomplete textbox using jquery and asp.net web service. Along the way, we also discuss the jquery autocomplete source option and minLength option.
jquery autocomplete source option can be set to
1. Array : An array of strings: [ "Choice1", "Choice2" ]
OR
An array of objects with label and value properties:
[ { label: "Choice1", value: "value1" }, ... ]
The label property is displayed in the suggestion menu. The value will be inserted
into the input element when a user selects an item.
2. String : When a string is used, the Autocomplete plugin expects that string to point to a URL resource that will return JSON data. Discussed in Part 71.
3. Callback function : This function has two parameters. request object and a response callback function. The term property of the request object contains the value the user typed in the autocomplete textbox and to the response callback function you will have to pass the data to suggest to the user.
jquery autocomplete minLength option : The minimum number of characters a user must type before a search is performed.
Steps to implement autocomplete textbox in an asp.net webforms application using jQuery and asp.net web service.
Step 1 : SQL Script to create and populate table tblStudents
Step 2 : Create a stored procedure to retrieve employee names
Step 3 : Create new asp.net web application project. Name it Demo.
Step 4 : Include a connection string in the web.config file to your database.
Step 5 : Add a WebService (ASMX) to the project. Name it StudentService.asmx. Copy and paste the following code.
Step 6 : Download jQuery UI from jqueryui.com. Copy and paste the following files in your project.
a) jquery-ui.js
b) jquery-ui.css
c) images
Step 7 : Add a WebForm to the ASP.NET project. Copy and paste the following HTML and jQuery code.
Part 70 - Load data on page scroll using jquery
Part 71 - Calling live json web service using jquery ajax
Part 72 - Autocomplete textbox using jquery in asp.net
In this video we will discuss, how to implement autocomplete textbox using jquery and asp.net web service. Along the way, we also discuss the jquery autocomplete source option and minLength option.
jquery autocomplete source option can be set to
1. Array : An array of strings: [ "Choice1", "Choice2" ]
OR
An array of objects with label and value properties:
[ { label: "Choice1", value: "value1" }, ... ]
The label property is displayed in the suggestion menu. The value will be inserted
into the input element when a user selects an item.
2. String : When a string is used, the Autocomplete plugin expects that string to point to a URL resource that will return JSON data. Discussed in Part 71.
3. Callback function : This function has two parameters. request object and a response callback function. The term property of the request object contains the value the user typed in the autocomplete textbox and to the response callback function you will have to pass the data to suggest to the user.
jquery autocomplete minLength option : The minimum number of characters a user must type before a search is performed.
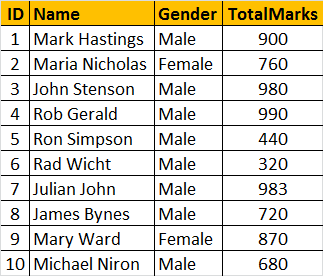
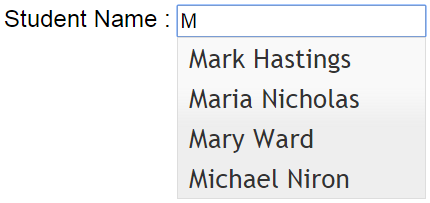
Steps to implement autocomplete textbox in an asp.net webforms application using jQuery and asp.net web service.
Step 1 : SQL Script to create and populate table tblStudents
Create Table tblStudents
(
ID int identity primary key,
Name nvarchar(50),
Gender nvarchar(20),
TotalMarks int
)
GO
Insert into tblStudents values('Mark Hastings','Male',900)
Insert into tblStudents values('Maria Nicholas','Female',760)
Insert into tblStudents values('John Stenson','Male',980)
Insert into tblStudents values('Rob Gerald','Male',990)
Insert into tblStudents values('Ron Simpson','Male',440)
Insert into tblStudents values('Rad Wicht','Male',320)
Insert into tblStudents values('Julian John','Male',983)
Insert into tblStudents values('James Bynes','Male',720)
Insert into tblStudents values('Mary Ward','Female',870)
Insert into tblStudents values('Michael Niron','Male',680)
Step 2 : Create a stored procedure to retrieve employee names
Create proc spGetStudentNames
@term nvarchar(50)
as
Begin
Select Name
from tblStudents where
Name like @term +
'%'
End
Step 3 : Create new asp.net web application project. Name it Demo.
Step 4 : Include a connection string in the web.config file to your database.
<add name="DBCS"
connectionString="server=.;database=SampleDB;integrated security=SSPI"/>
Step 5 : Add a WebService (ASMX) to the project. Name it StudentService.asmx. Copy and paste the following code.
using System.Collections.Generic;
using System.Configuration;
using System.Data;
using System.Data.SqlClient;
using System.Web.Services;
namespace Demo
{
[WebService(Namespace = "http://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
[System.ComponentModel.ToolboxItem(false)]
[System.Web.Script.Services.ScriptService]
public class StudentService : System.Web.Services.WebService
{
[WebMethod]
public List<string>
GetStudentNames(string term)
{
List<string> listStudentNames = new List<string>();
string cs = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(cs))
{
SqlCommand cmd = new SqlCommand("spGetStudentNames", con);
cmd.CommandType = CommandType.StoredProcedure;
cmd.Parameters.Add(new SqlParameter()
{
ParameterName = "@term",
Value = term
});
con.Open();
SqlDataReader rdr = cmd.ExecuteReader();
while (rdr.Read())
{
listStudentNames.Add(rdr["Name"].ToString());
}
}
return listStudentNames;
}
}
}
Step 6 : Download jQuery UI from jqueryui.com. Copy and paste the following files in your project.
a) jquery-ui.js
b) jquery-ui.css
c) images
Step 7 : Add a WebForm to the ASP.NET project. Copy and paste the following HTML and jQuery code.
<%@ Page Language="C#" AutoEventWireup="true"
CodeBehind="WebForm1.aspx.cs" Inherits="Demo.WebForm1" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<script src="jquery-1.11.2.js"></script>
<script src="jquery-ui.js"></script>
<link href="jquery-ui.css" rel="stylesheet" />
<script type="text/javascript">
$(document).ready(function () {
$('#txtName').autocomplete({
minLength: 2,
source: function (request, response)
{
$.ajax({
url: 'StudentService.asmx/GetStudentNames',
method: 'post',
contentType: 'application/json;charset=utf-8',
data: JSON.stringify({
term: request.term }),
dataType: 'json',
success: function (data) {
response(data.d);
},
error: function (err) {
alert(err);
}
});
}
});
});
</script>
</head>
<body>
<form id="form1" runat="server">
Student Name :
<asp:TextBox ID="txtName" runat="server">
</asp:TextBox>
</form>
</body>
</html>
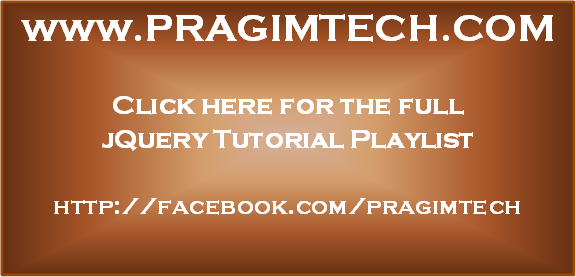
if webform2.aspx pages are stored in folders called "Pages", the url in $ .ajax ({url: '']) would like
ReplyDeleteIs there any difference in using normal HTML page and HTML text box instead of an ASPX page and ASP text box with the same generic handler. Also would like learn more about the difference of using generic handler and a web service. Thanks in advance...
ReplyDeleteThanks but just a small note
ReplyDeletethat code will not work in case working with master page because it will not able to find txtName ,
to make it work with master pages , we should add ClientIDMode=static
or call the id with <%%>