Suggested Videos
Part 41 - jQuery preventdefault
Part 42 - jQuery scroll event
Part 43 - jQuery image gallery
In this video we will discuss how to optimise the image gallery we created in Part 43, using the concept of event bubbling. We will also enhance the example, using a dropdownlist for effects and duration. We will also be able to enlarge and shrink the height and width of the main image. Please watch Part 43 from jQuery tutorial before proceeding.
The problem with the image gallery that we created in Part 43 is that we are binding event handlers (mouseover, mouseout & click) to every image element. This means if you have 500 image elements, then there will be 1500 event handlers (mouseover, mouseout & click) in the memory and this may negatively affect the performance of your application.
A better way of doing the same from a performance standpoint is shown below. In this example, the event handlers are attached to the div element and not to the individual img elements. So, even if you have 500 img elements, there are only 3 event handlers in memory.
So how does this work
1. When you click on an img element, the event gets bubbled up to its parent (div) as the img element does not have an event handler
2. The bubbled event is handled by the the parent (div) element, as it has a click event handler.
Part 41 - jQuery preventdefault
Part 42 - jQuery scroll event
Part 43 - jQuery image gallery
In this video we will discuss how to optimise the image gallery we created in Part 43, using the concept of event bubbling. We will also enhance the example, using a dropdownlist for effects and duration. We will also be able to enlarge and shrink the height and width of the main image. Please watch Part 43 from jQuery tutorial before proceeding.

The problem with the image gallery that we created in Part 43 is that we are binding event handlers (mouseover, mouseout & click) to every image element. This means if you have 500 image elements, then there will be 1500 event handlers (mouseover, mouseout & click) in the memory and this may negatively affect the performance of your application.
A better way of doing the same from a performance standpoint is shown below. In this example, the event handlers are attached to the div element and not to the individual img elements. So, even if you have 500 img elements, there are only 3 event handlers in memory.
So how does this work
1. When you click on an img element, the event gets bubbled up to its parent (div) as the img element does not have an event handler
2. The bubbled event is handled by the the parent (div) element, as it has a click event handler.
<html>
<head>
<style type="text/css">
.imgStyle {
width: 100px;
height: 100px;
border: 3px solid grey;
}
</style>
<script src="jquery-1.11.2.js"></script>
<script type="text/javascript">
$(document).ready(function () {
$('#divId').on({
mouseover: function () {
$(this).css({
'cursor': 'hand',
'border-Color': 'red'
});
},
mouseout: function () {
$(this).css({
'cursor': 'default',
'border-Color': 'grey'
});
},
click: function () {
var imageURL = $(this).attr('src');
var effect = $('#selectImgEffect').val();
var duration = $('#selectImgDuration').val()
* 1000;
if (effect == 'Slide') {
$('#mainImage').slideUp(duration,
function
() {
$(this).attr('src', imageURL);
}).slideDown(duration);
}
else {
$('#mainImage').fadeOut(duration,
function
() {
$(this).attr('src', imageURL);
}).fadeIn(duration);
}
}
}, 'img');
var mainImageElement = $('#mainImage');
var height = parseInt(mainImageElement.attr('height'));
var width = parseInt(mainImageElement.attr('width'))
$('#btnEnlarge').click(function () {
height += 100;
width += 100;
mainImageElement.animate({ 'height': height, 'width': width });
});
$('#btnShrink').click(function () {
height -= 100;
width -= 100;
mainImageElement.animate({ 'height': height, 'width': width });
});
});
</script>
</head>
<body style="font-family:Arial">
Select Effect :
<select id="selectImgEffect">
<option value="Fade">Fade</option>
<option value="Slide">Slide</option>
</select>
Time in seconds:
<select id="selectImgDuration">
<option value="0.5">0.5</option>
<option value="1">1</option>
<option value="2">2</option>
<option value="3">3</option>
<option value="4">4</option>
<option value="5">5</option>
</select>
<input id="btnEnlarge" type="button" value="Enlarge" />
<input id="btnShrink" type="button" value="Shrink" />
<br /><br />
<img id="mainImage" style="border:3px solid grey"
src="/Images/Hydrangeas.jpg" height="500" width="540" />
<br />
<div id="divId">
<img class="imgStyle" src="/Images/Hydrangeas.jpg" />
<img class="imgStyle" src="/Images/Jellyfish.jpg" />
<img class="imgStyle" src="/Images/Koala.jpg" />
<img class="imgStyle" src="/Images/Penguins.jpg" />
<img class="imgStyle" src="/Images/Tulips.jpg" />
</div>
</body>
</html>
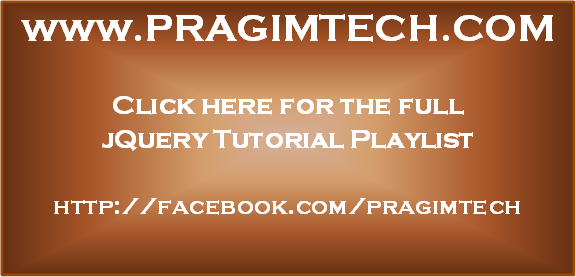
No comments:
Post a Comment
It would be great if you can help share these free resources