Suggested Videos
Part 12 - Select values of checkbox group with jquery
Part 13 - jQuery get selected checkbox text
Part 14 - jQuery selected selector
In this video we will discuss
1. Use of jQuery each function
2. How to exit from each function in jQuery
3. Implicit iteration in jQuery
4. Performance considerations when using jquery each function
jQuery each function is used to iterate over the items in a collection. For each item in the collection the anonymous function is called. The index of the element and the element itself are passed to the anonymous function.
To refer to the current element that we are iterating over you can also use this keyword.
If you don't care about the index and just need the text of the list item, then you can get rid of the index parameter
How to exit from each function in jQuery : To exit from each function, return false.
Implicit iteration in jQuery : The $('li') selector returns all list item elements. Notice that we are calling the css() jquery function on the jquery collection itself. In this case, css() method implicitly iterates over each element in the entire collection.
There is no need to explicitly iterate over each element in the collection. Most jQuery methods implicitly iterate over the entire collection.
Performance considerations when using jquery each function
From a performance standpoint, there are 2 problems with the above code
1. jQuery needs to search the DOM for div element with id = divResult, for each list item in the collection. Since there are 5 list items, jquery searches the DOM 5 times for the same div element with id = divResult. The performance of the above code can be improved by caching the divResult element.
2. The DOM element (div element with id = divResult) is being updated on each iteration. Again this is bad for performance. To improve the performance build a string variable with the required data on each iteration of the loop. Once the loop has completed, update the DOM element with the value that is present in the string varibale. This will ensure that the DOM element is updated only once and this is much better for performance.
The following is much better from a performance standpoint
Part 12 - Select values of checkbox group with jquery
Part 13 - jQuery get selected checkbox text
Part 14 - jQuery selected selector
In this video we will discuss
1. Use of jQuery each function
2. How to exit from each function in jQuery
3. Implicit iteration in jQuery
4. Performance considerations when using jquery each function
jQuery each function is used to iterate over the items in a collection. For each item in the collection the anonymous function is called. The index of the element and the element itself are passed to the anonymous function.
$('li').each(function (index, element) {
alert(index + ' : ' +
$(element).text());
});
To refer to the current element that we are iterating over you can also use this keyword.
$('li').each(function (index) {
alert(index + ' : ' + $(this).text());
});
If you don't care about the index and just need the text of the list item, then you can get rid of the index parameter
$('li').each(function () {
alert($(this).text());
});
How to exit from each function in jQuery : To exit from each function, return false.
<script type="text/javascript">
$(document).ready(function () {
$('li').each(function () {
if ($(this).text() == 'UK') {
return false;
}
alert($(this).text());
});
});
</script>
Implicit iteration in jQuery : The $('li') selector returns all list item elements. Notice that we are calling the css() jquery function on the jquery collection itself. In this case, css() method implicitly iterates over each element in the entire collection.
<html>
<head>
<title></title>
<script src="jquery-1.11.2.js"></script>
<script type="text/javascript">
$(document).ready(function () {
$('li').css('color', 'red');
});
</script>
</head>
<body style="font-family:Arial">
<ul>
<li>US</li>
<li>India</li>
<li>UK</li>
<li>Canada</li>
<li>Australia</li>
</ul>
</body>
</html>There is no need to explicitly iterate over each element in the collection. Most jQuery methods implicitly iterate over the entire collection.
<script type="text/javascript">
$(document).ready(function () {
$('li').each(function () {
$(this).css('color', 'red');
});
});
</script>
Performance considerations when using jquery each function
<html>
<head>
<title></title>
<script src="jquery-1.11.2.js"></script>
<script type="text/javascript">
$(document).ready(function () {
$('li').each(function () {
$('#divResult').html($('#divResult').html()
+ '<br/>' + $(this).text())
});
});
</script>
</head>
<body style="font-family:Arial">
<ul>
<li>US</li>
<li>India</li>
<li>UK</li>
<li>Canada</li>
<li>Australia</li>
</ul>
<div id="divResult"></div>
</body>
</html>
From a performance standpoint, there are 2 problems with the above code
1. jQuery needs to search the DOM for div element with id = divResult, for each list item in the collection. Since there are 5 list items, jquery searches the DOM 5 times for the same div element with id = divResult. The performance of the above code can be improved by caching the divResult element.
2. The DOM element (div element with id = divResult) is being updated on each iteration. Again this is bad for performance. To improve the performance build a string variable with the required data on each iteration of the loop. Once the loop has completed, update the DOM element with the value that is present in the string varibale. This will ensure that the DOM element is updated only once and this is much better for performance.
The following is much better from a performance standpoint
<script type="text/javascript">
$(document).ready(function () {
var result = '';
$('li').each(function () {
result += '<br/>' + $(this).text();
});
$('#divResult').html(result);
});
</script>
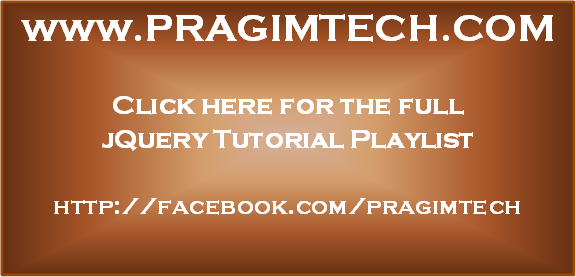
You are the best teacher I have ever had in my life :). Stay Happy Stay Blessed :)
ReplyDeleteyou are very very great teacher
ReplyDeleteI have never seen such kind of training till date.
ReplyDeleteYou are code guru. Thank you Venkat. May god bless you.
ReplyDelete