Suggested Videos
Part 16 - jQuery each function
Part 17 - jQuery method chaining
Part 18 - What is JSON
In this video we will discuss
1. How to convert JSON object to string
2. How to convert string to JSON object
This is continuation Part 18. Please watch Part 18 from jQuery tutorial before proceeding.
The following example converts JSON array to a string. JSON.stringify() method converts a JSON object (or array) into a JSON string.
Output :
[{"firstName":"Todd","lastName":"Grover","gender":"Male","salary":50000},
{"firstName":"Sara","lastName":"Baker","gender":"Female","salary":40000}]
The following example converts a JSON string to a JSON array. JSON.parse() method converts a JSON string to JSON array. We then use the jQuery each() method to loop thru each employee JSON object and retrieve the respective property values.
Output :
Part 16 - jQuery each function
Part 17 - jQuery method chaining
Part 18 - What is JSON
In this video we will discuss
1. How to convert JSON object to string
2. How to convert string to JSON object
This is continuation Part 18. Please watch Part 18 from jQuery tutorial before proceeding.
The following example converts JSON array to a string. JSON.stringify() method converts a JSON object (or array) into a JSON string.
<html>
<head>
<title></title>
<script src="jquery-1.11.2.js"></script>
<script type="text/javascript">
$(document).ready(function () {
var employeesJSON = [
{
"firstName": "Todd",
"lastName": "Grover",
"gender": "Male",
"salary": 50000
},
{
"firstName": "Sara",
"lastName": "Baker",
"gender": "Female",
"salary": 40000
}
];
var JSONString = JSON.stringify(employeesJSON);
$('#resultDiv').html(JSONString);
});
</script>
</head>
<body style="font-family:Arial">
<div id="resultDiv"></div>
</body>
</html>
Output :
[{"firstName":"Todd","lastName":"Grover","gender":"Male","salary":50000},
{"firstName":"Sara","lastName":"Baker","gender":"Female","salary":40000}]
The following example converts a JSON string to a JSON array. JSON.parse() method converts a JSON string to JSON array. We then use the jQuery each() method to loop thru each employee JSON object and retrieve the respective property values.
<html>
<head>
<title></title>
<script src="jquery-1.11.2.js"></script>
<script type="text/javascript">
$(document).ready(function () {
var JSONString = '[{
"firstName": "Todd", "lastName":
"Grover", "gender": "Male", "salary":
50000 }, { "firstName": "Sara", "lastName":
"Baker", "gender": "Female", "salary":
40000 }]';
var employeesJSON = JSON.parse(JSONString);
var result = '';
$.each(employeesJSON, function (i, item) {
result += 'First Name = ' +
item.firstName + '<br/>';
result += 'Last Name = ' +
item.lastName + '<br/>';
result += 'Gender = ' +
item.gender + '<br/>';
result += 'Salary = ' +
item.salary + '<br/><br/>';
});
$('#resultDiv').html(result);
});
</script>
</head>
<body style="font-family:Arial">
<div id="resultDiv"></div>
</body>
</html>
Output :

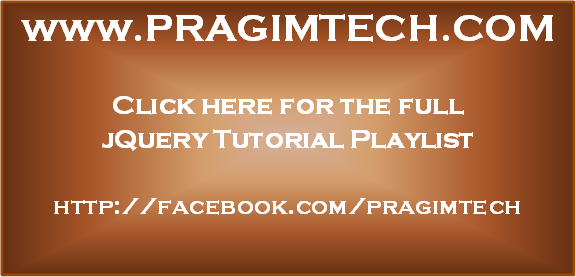
Hi sir , i am not able to see the JSON keyword in the intellisense. Is there any problem somewhere
ReplyDeleteImport the Package "using Newtonsoft.Json;"
Deleteor right-click and select resolve.