Suggested Videos
Part 10 - ASP.NET control client id in JavaScript
Part 11 - JavaScript calendar date picker for ASP.NET
Part 12 - Warn user before leaving web page with unsaved changes
In this video we will discuss how to implement a simple password strength checker using JavaScript.
As we type the password in the password TextBox, the strength of the password should be displayed in a label control.
The password strength is determined based on the following scoring criteria.
The password strength is determined using the following scale.
Example :
Part 10 - ASP.NET control client id in JavaScript
Part 11 - JavaScript calendar date picker for ASP.NET
Part 12 - Warn user before leaving web page with unsaved changes
In this video we will discuss how to implement a simple password strength checker using JavaScript.
As we type the password in the password TextBox, the strength of the password should be displayed in a label control.

The password strength is determined based on the following scoring criteria.
If the password contain at least 8 characters | 20 Points |
If the password contain at least 1 lowercase letter | 20 Points |
If the password contain at least 1 uppercase letter | 20 Points |
If the password contain at least 1 special character | 20 Points |
The password strength is determined using the following scale.
Password score | Password Strength |
>= 100 Strong | Strong |
>= 80 | Medium |
>= 60 | Weak |
< 60 | Very Weak |
Example :
<asp:TextBox ID="TextBox1" runat="server" TextMode="Password"
onkeyup="checkPasswordStrength()">
</asp:TextBox>
<asp:Label ID="lblMessage" runat="server"></asp:Label>
<script type="text/javascript">
function checkPasswordStrength()
{
var passwordTextBox = document.getElementById("TextBox1");
var password = passwordTextBox.value;
var specialCharacters = "!£$%^&*_@#~?";
var passwordScore = 0;
passwordTextBox.style.color = "white";
// Contains special characters
for (var i =
0; i < password.length; i++)
{
if (specialCharacters.indexOf(password.charAt(i))
> -1)
{
passwordScore += 20;
break;
}
}
// Contains numbers
if (/\d/.test(password))
passwordScore += 20;
// Contains lower case letter
if (/[a-z]/.test(password))
passwordScore += 20;
// Contains upper case letter
if (/[A-Z]/.test(password))
passwordScore += 20;
if (password.length >= 8)
passwordScore += 20;
var strength = "";
var backgroundColor = "red";
if (passwordScore >= 100)
{
strength = "Strong";
backgroundColor = "green";
}
else if (passwordScore
>= 80)
{
strength = "Medium";
backgroundColor = "gray";
}
else if (passwordScore
>= 60)
{
strength = "Weak";
backgroundColor = "maroon";
}
else
{
strength = "Very
Weak";
backgroundColor = "red";
}
document.getElementById("lblMessage").innerHTML =
strength;
passwordTextBox.style.backgroundColor =
backgroundColor;
}
</script>
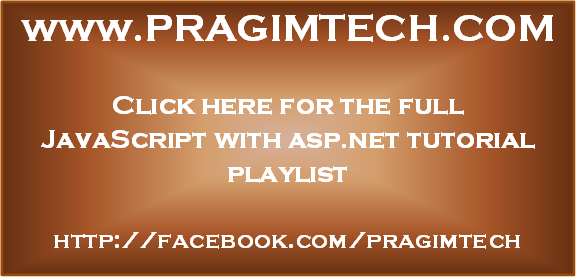
No comments:
Post a Comment
It would be great if you can help share these free resources