Suggested Videos
Part 66 - Strict Mode in JavaScript
Part 67 - JavaScript Cookies
Part 68 - JavaScript cookie attributes
In this video we will discuss, if it's possible to store multiple key value pairs in a cookie. Let us understand this with an example.
Design a web page as shown in the image below
When we click "Set Cookie" button, we want to store name, email & gender along with their values in the cookie.
Copy and paste the following code in HTMLPage1.htm
1. Run the application.
2. Fill in data for Name, Email and Gender.
3. Click "Set Cookie" button
4. Click "Get Cookie" button
Notice that we only get the first key-value pair. This is because you can only store one key-value pair in one cookie
name=Venkat
If you want to store all the 3 key-value pairs, you have 2 options
1. Create a custom object, serialize the object to a JSON string and store the serialized string in a cookie
2. Use a different cookie for each key-value pair you want to store
Create a custom object, serialize the object to a JSON string and store the serialized string in a cookie :
Modify the code in setCookie() function as shown below.
JSON.stringify() method converts a JavaScript object to a JavaScript Object Notation (JSON) string.
Modify the code in getCookie() function as shown below.
JSON.parse() method parses a JSON string and returns the corresponding object.
In our next video we will discuss the second option, i.e using a different cookie for each key-value pair that we want to store.
Part 66 - Strict Mode in JavaScript
Part 67 - JavaScript Cookies
Part 68 - JavaScript cookie attributes
In this video we will discuss, if it's possible to store multiple key value pairs in a cookie. Let us understand this with an example.
Design a web page as shown in the image below
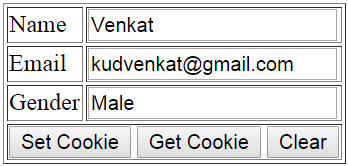
When we click "Set Cookie" button, we want to store name, email & gender along with their values in the cookie.
Copy and paste the following code in HTMLPage1.htm
<html>
<head></head>
<body>
<table border="1">
<tr>
<td>Name</td>
<td><input type="text" id="txtName" /></td>
</tr>
<tr>
<td>Email</td>
<td><input type="text" id="txtEmail" /></td>
</tr>
<tr>
<td>Gender</td>
<td><input type="text" id="txtGender" /></td>
</tr>
<tr>
<td colspan="2">
<input type="button" value="Set
Cookie" onclick="setCookie()" />
<input type="button" value="Get Cookie" onclick="getCookie()" />
<input type="button" value="Clear" onclick="clearTextBoxes()" />
</td>
</tr>
</table>
<script type="text/javascript">
function setCookie()
{
var cookieString = "name="
+ document.getElementById("txtName").value
+
";email=" + document.getElementById("txtEmail").value +
";gender=" + document.getElementById("txtGender").value;
document.cookie = cookieString;
}
function getCookie()
{
alert(document.cookie);
}
function clearTextBoxes()
{
document.getElementById("txtName").value = "";
document.getElementById("txtEmail").value = "";
document.getElementById("txtGender").value = "";
}
</script>
</body>
</html>
1. Run the application.
2. Fill in data for Name, Email and Gender.
3. Click "Set Cookie" button
4. Click "Get Cookie" button
Notice that we only get the first key-value pair. This is because you can only store one key-value pair in one cookie
name=Venkat
If you want to store all the 3 key-value pairs, you have 2 options
1. Create a custom object, serialize the object to a JSON string and store the serialized string in a cookie
2. Use a different cookie for each key-value pair you want to store
Create a custom object, serialize the object to a JSON string and store the serialized string in a cookie :
Modify the code in setCookie() function as shown below.
function setCookie()
{
var customObject = {};
customObject.name = document.getElementById("txtName").value;
customObject.email = document.getElementById("txtEmail").value;
customObject.gender = document.getElementById("txtGender").value;
var jsonString = JSON.stringify(customObject);
document.cookie = "cookieObject=" + jsonString;
}
JSON.stringify() method converts a JavaScript object to a JavaScript Object Notation (JSON) string.
Modify the code in getCookie() function as shown below.
function getCookie()
{
var nameValueArray = document.cookie.split("=");
var customObject = JSON.parse(nameValueArray[1]);
document.getElementById("txtName").value = customObject.name;
document.getElementById("txtEmail").value = customObject.email;
document.getElementById("txtGender").value = customObject.gender;
}
JSON.parse() method parses a JSON string and returns the corresponding object.
In our next video we will discuss the second option, i.e using a different cookie for each key-value pair that we want to store.
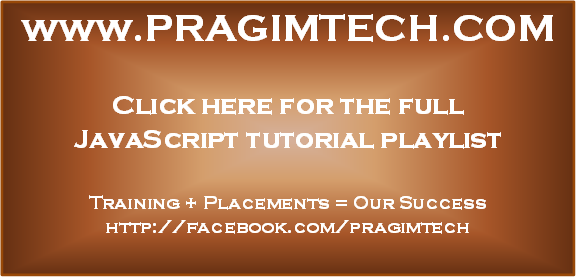
can you have MVVM + WPF video if yes then pls upload it, if no then pls make soon.
ReplyDeleteI am seen many video regarding .net over internet some are very good but not completed, but you know how to teach beginning to advance, your all video in sequence this is big + point for us, i hope in soon from your side we get more video, my request to you only please make video and give example there using code those are using in real time scenario .
ReplyDeleteGod Bless you. you are the best
If any one need to learn how to teach other then Kud Sir is best. He know how to teach all kind of students and processionals. many one have good knowledge but they do not how to teach. This is art.
ReplyDeletePls always share our experice rest world those are poor and want learn from you.
Please use your real working scenario in your all video examples, this is helpful for working professionals and students too.
ReplyDeletePlease share videos on Design Pattern and Testing.
ReplyDeleteThanks
Hi Venkat, I've tried this example using two browsers, Google Chrome( Version 40.0.2214.115 m (64-bit)) & Firefox(27.0.1). This worked only with Firefox. Any ideas regarding not working with Chrome???
ReplyDeleteDear Venkat, Thanks for sharing this :-)
ReplyDeleteHi Venkat,
ReplyDeleteYour videos are very helpful, please upload videos on Unit Test Projects and Design patterns and Dependency Injection