Suggested Videos
Part 40 - Event bubbling in JavaScript
Part 41 - Image gallery with thumbnails in JavaScript
Part 42 - JavaScript event capturing
In this video we will discuss how to prevent browser default action. First let's look at some of the browser default actions. For example,
1. When you click on a link, the browser navigates to the page specified in the link
2. When you right click on a web page, the browser displays the context menu
In some situations you may want to prevent these default actions of the browser. For example some of the websites prevent you from right clicking on the page. Disabling right click is annoying users. Many people say they disabled right click for security, because they do not want their content to be copied. But if you disable JavaScript in the browser, you will still be able to right click and copy the content. So you are achieving nothing by disabling right click.
Having said that, now let us see how to prevent the context menu from appearing when you right click on the web page. There are 2 ways you can do this.
Using oncontextmenu attribute of the body element to disable right click
Using the event object to disable right click
IE 8 and earlier versions
event.returnValue = false;
IE 9 & later versions and all other browsers
event.preventDefault();
When you click on a link, how to prevent the browser from navigating to the page specified in the link
OR
Part 40 - Event bubbling in JavaScript
Part 41 - Image gallery with thumbnails in JavaScript
Part 42 - JavaScript event capturing
In this video we will discuss how to prevent browser default action. First let's look at some of the browser default actions. For example,
1. When you click on a link, the browser navigates to the page specified in the link
2. When you right click on a web page, the browser displays the context menu
In some situations you may want to prevent these default actions of the browser. For example some of the websites prevent you from right clicking on the page. Disabling right click is annoying users. Many people say they disabled right click for security, because they do not want their content to be copied. But if you disable JavaScript in the browser, you will still be able to right click and copy the content. So you are achieving nothing by disabling right click.
Having said that, now let us see how to prevent the context menu from appearing when you right click on the web page. There are 2 ways you can do this.
Using oncontextmenu attribute of the body element to disable right click
<html>
<head>
</head>
<body oncontextmenu="return false">
<h1>On this page right click is disabled</h1>
</body>
</html>
Using the event object to disable right click
IE 8 and earlier versions
event.returnValue = false;
IE 9 & later versions and all other browsers
event.preventDefault();
<h1>On this page right click is disabled</h1>
<script type="text/javascript">
document.oncontextmenu
= disableRightClick;
function disableRightClick(event)
{
event = event || window.event;
if (event.preventDefault)
{
event.preventDefault();
}
else
{
event.returnValue = false
}
}
</script>
When you click on a link, how to prevent the browser from navigating to the page specified in the link
<a href="http://pragimtech.com" onclick="return false">
Clicking on the link will not take you to
PragimTech
</a>
OR
<a href="http://pragimtech.com" onclick="preventLinkNavigation(event)">
Clicking on the link will not take you to
PragimTech
</a>
<script type="text/javascript">
function preventLinkNavigation(event)
{
event = event || window.event;
if (event.preventDefault)
{
event.preventDefault();
}
else
{
event.returnValue = false
}
}
</script>
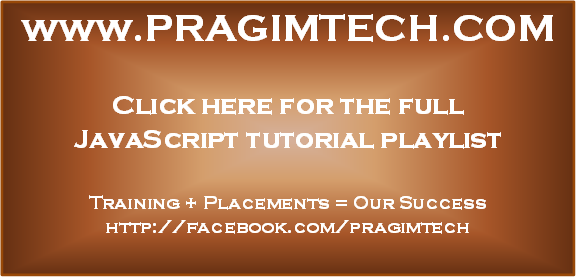
Awesome. I have used Prevent default in window.onbeforeunload. But confirmation message box will pop up asking "Leaving the page/Stay on the Page" Is there a way to avoid this default message box. instead i should use custom dialog modal.
ReplyDelete