Suggested Videos
Part 32 - JavaScript window.onerror event
Part 33 - Working with dates in javascript
Part 34 - JavaScript timing events
In this video, we will discuss creating a simple image slideshow using JavaScript. We will be using setInterval() and clearInterval() JavaScript methods to achieve this. We discussed these functions in detail in Part 34 of JavaScript Tutorial.
The slideshow should be as shown in the image below. When you click "Start Slide Show" button the image slideshow should start and when you click the "Stop Slide Show" button the image slideshow should stop.
For the purpose of this demo we will be using the images that can be found on any windows machine at the following path.
C:\Users\Public\Pictures\Sample Pictures
At the above location, on my machine I have 8 images. Here are the steps to create the image slideshow using JavaScript.
Step 1 : Open Visual Studio and create a new empty asp.net web application project. Name it Demo.
Step 2 : Right click on the Project Name in Solution Explorer in Visual Studio and create a new folder with name = Images.
Step 3 : Copy the 8 images from C:\Users\Public\Pictures\Sample Pictures to Images folder in your project. Change the names of the images to 1.jpg, 2.jpg etc.
Step 4 : Right click on the Project Name in Solution Explorer in Visual Studio and add a new HTML Page. It should automatically add HTMLPage1.htm. At this point your solution explorer should look as shown below.
Step 5 : Copy and paste the following HTML and JavaScript code in HTMLPage1.htm page.
<img id="image" src="/Images/1.jpg" style="width: 150px; height: 150px" />
Finally run the application and test it.
Part 32 - JavaScript window.onerror event
Part 33 - Working with dates in javascript
Part 34 - JavaScript timing events
In this video, we will discuss creating a simple image slideshow using JavaScript. We will be using setInterval() and clearInterval() JavaScript methods to achieve this. We discussed these functions in detail in Part 34 of JavaScript Tutorial.
The slideshow should be as shown in the image below. When you click "Start Slide Show" button the image slideshow should start and when you click the "Stop Slide Show" button the image slideshow should stop.

For the purpose of this demo we will be using the images that can be found on any windows machine at the following path.
C:\Users\Public\Pictures\Sample Pictures
At the above location, on my machine I have 8 images. Here are the steps to create the image slideshow using JavaScript.
Step 1 : Open Visual Studio and create a new empty asp.net web application project. Name it Demo.
Step 2 : Right click on the Project Name in Solution Explorer in Visual Studio and create a new folder with name = Images.
Step 3 : Copy the 8 images from C:\Users\Public\Pictures\Sample Pictures to Images folder in your project. Change the names of the images to 1.jpg, 2.jpg etc.
Step 4 : Right click on the Project Name in Solution Explorer in Visual Studio and add a new HTML Page. It should automatically add HTMLPage1.htm. At this point your solution explorer should look as shown below.

Step 5 : Copy and paste the following HTML and JavaScript code in HTMLPage1.htm page.
<img id="image" src="/Images/1.jpg" style="width: 150px; height: 150px" />
<br />
<input type="button" value="Start Slide Show" onclick="startImageSlideShow()" />
<input type="button" value="Stop Slide Show" onclick="stopImageSlideShow()" />
<script type="text/javascript">
var intervalId;
function startImageSlideShow()
{
intervalId = setInterval(setImage,
500);
}
function stopImageSlideShow()
{
clearInterval(intervalId);
}
function setImage()
{
var imageSrc = document.getElementById("image").getAttribute("src");
var currentImageNumber = imageSrc.substring(imageSrc.lastIndexOf("/") + 1,
imageSrc.lastIndexOf("/") + 2);
if (currentImageNumber == 8)
{
currentImageNumber = 0;
}
document.getElementById("image").setAttribute("src", "/Images/"
+
(Number(currentImageNumber)
+ 1) + ".jpg");
}
</script>
Finally run the application and test it.
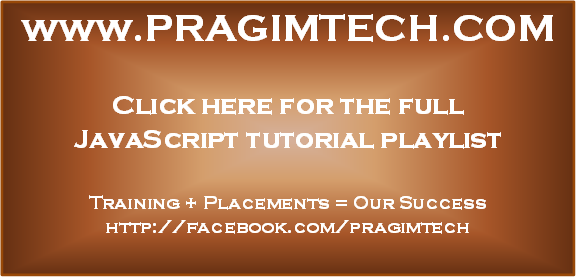
I have a question about the slideshow. In this situation we have a static situation with 8 pictures. But in a dynamic situation we have more or less pictures to show. How can you made this slideshow dynamic without recompeling the application?
ReplyDeleteI used same code in asp.net Page and i also put the script tag before body section but its not workig what i have to do if i wanna same thing in aspx page please guide me i also search in internet ,but i didnot get any solution
ReplyDeletehow to handle slide show.
ReplyDelete1)if we clicks dirctly on Stop Slide Show with out click on start Slide Show .it is giving undefined interval
Hi i am trying to create image slideshow using javascript in visual studio code instead of visual studio , and am getting error: Not allowed to load local resource: ~/Desktop/js-basics/images/1.jpg . Can anyone help please ? And i am using Chrome browser to run my html + javascript code.
ReplyDelete