Suggested Videos
Part 34 - JavaScript timing events
Part 35 - How to create image slideshow using JavaScript
Part 36 - Events in JavaScript
In JavaScript there are several ways to associate an event handler to the event. In Part 36, we discussed, associating event handler methods to events using the attributes of HTML tags. In this video we will discuss using DOM object property to assign event handlers to events.
First let us understand, what is DOM
DOM stands for Document Object Model. When a browser loads a web page, the browser creates a Document Object Model of that page. The HTML DOM is created as a tree of Objects.
Example :
For the above HTML a graphical representation of the Document Object Model is shown below.
JavaScript can be used to access and modify these DOM objects and their properties. For example, you can add, modify and remove HTML elements and their attributes. Along the same lines, you can use DOM object properties to assign event handlers to events. We will discuss the DOM object in detail in a later video session.
We will continue with the same examples that we worked with in Part 36. Notice that in this case, we are assigning event handlers using the DOM object properties (onmouseover & onmouseout) instead of using the attributes of the HTML tag. We are using this keyword to reference the current HTML element. In this example "this" references the button control.
The following example is same as the above. In this case we are assigning an anonymous function to onmouseover & onmouseout properties.
If an event handler is assigned using both, i.e an HTML attribute and DOM object property, the handler that is assigned using the DOM object property overwrites the one assigned using HTML attribute. Here is an example.
Using this approach you can only assign one event handler method to a given event. The handler that is assigned last wins. In the following example, Handler2() is assigned after Handler1. So Handler2() owerites Handler1().
Part 34 - JavaScript timing events
Part 35 - How to create image slideshow using JavaScript
Part 36 - Events in JavaScript
In JavaScript there are several ways to associate an event handler to the event. In Part 36, we discussed, associating event handler methods to events using the attributes of HTML tags. In this video we will discuss using DOM object property to assign event handlers to events.
First let us understand, what is DOM
DOM stands for Document Object Model. When a browser loads a web page, the browser creates a Document Object Model of that page. The HTML DOM is created as a tree of Objects.
Example :
<html>
<head>
<title>My Page Title</title>
</head>
<body>
<script type="text/javascript">
</script>
<div>
<h1>This is browser DOM</h1>
</div>
</body>
</html>
For the above HTML a graphical representation of the Document Object Model is shown below.

JavaScript can be used to access and modify these DOM objects and their properties. For example, you can add, modify and remove HTML elements and their attributes. Along the same lines, you can use DOM object properties to assign event handlers to events. We will discuss the DOM object in detail in a later video session.
We will continue with the same examples that we worked with in Part 36. Notice that in this case, we are assigning event handlers using the DOM object properties (onmouseover & onmouseout) instead of using the attributes of the HTML tag. We are using this keyword to reference the current HTML element. In this example "this" references the button control.
<input type="button" value="Click me" id="btn"/>
<script type="text/javascript">
document.getElementById("btn").onmouseover = changeColorOnMouseOver;
document.getElementById("btn").onmouseout = changeColorOnMouseOut;
function changeColorOnMouseOver()
{
this.style.background = 'red';
this.style.color = 'yellow';
}
function changeColorOnMouseOut()
{
this.style.background = 'black';
this.style.color = 'white';
}
</script>
The following example is same as the above. In this case we are assigning an anonymous function to onmouseover & onmouseout properties.
<input type="button" value="Click me" id="btn" />
<script type="text/javascript">
document.getElementById("btn").onmouseover = function ()
{
this.style.background = 'red';
this.style.color = 'yellow';
}
document.getElementById("btn").onmouseout = function ()
{
this.style.background = 'black';
this.style.color = 'white';
}
</script>
If an event handler is assigned using both, i.e an HTML attribute and DOM object property, the handler that is assigned using the DOM object property overwrites the one assigned using HTML attribute. Here is an example.
<input type="button" value="Click me" id="btn" onclick="clickHandler1()"/>
<script type="text/javascript">
document.getElementById("btn").onclick = clickHandler2;
function clickHandler1()
{
alert("Handler set
using HTML attribute");
}
function clickHandler2()
{
alert("Handler set
using DOM object property");
}
</script>
Using this approach you can only assign one event handler method to a given event. The handler that is assigned last wins. In the following example, Handler2() is assigned after Handler1. So Handler2() owerites Handler1().
<input type="button" value="Click me" id="btn"/>
<script type="text/javascript">
document.getElementById("btn").onclick = clickHandler1;
document.getElementById("btn").onclick = clickHandler2;
function clickHandler1()
{
alert("Handler
1");
}
function clickHandler2()
{
alert("Handler
2");
}
</script>
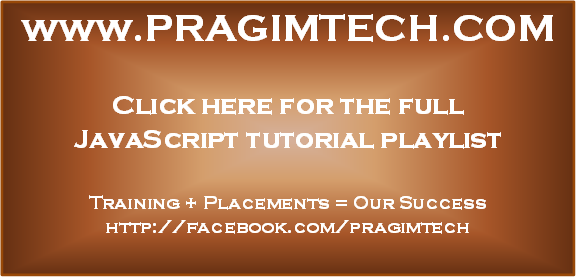
How to pass parameters here :
ReplyDeletedocument.getElementById("btn").onclick = clickHandler1
document.getElementById("btn").onclick = function(){clickHandler1(passparameterhere)}
DeleteHere is the example of changing the color the button onmouseover
//
//we create the function of OnMouseover
function changeColorOnMouseOver(id)
{
var ids = document.getElementById(id);
ids.style.background = "black";
ids.style.color = "white";
}
//calling the above function with parameter
document.onmouseover = function () { changeColorOnMouseOver("startSlideShow") };
//
thanx sir,
ReplyDeleteplease provide a tutorial on
difference beween calling a function with paranthesis and without parenthesis