Suggested Videos
Part 33 - Working with dates in javascript
Part 34 - JavaScript timing events
Part 35 - How to create image slideshow using JavaScript
What is an event
An event is a signal from the browser that something has happened. For example,
1. When a user clicks on an HTML element, click event occurs
2. When a user moves the mouse over an HTML element, mouseover event occurs
When events occur, we can execute JavaScript code or functions in response to those events. To do this we need to associate JavaScript code or functions to the events. The function that executes in response to an event is called event handler.
In JavaScript, there are several ways to associate an event handler to the event
1. Using the attributes of an HTML tag
2. Using DOM object property
3. Using special methods
In this video we will discuss associating event handler methods to events using the attributes of HTML tags.
In the following example, the code to execute in response to onmouseover & onmouseout events is set directly in the HTML markup. The keyword "this" references the current element. In this example "this" references the button control.
The above example, can be rewritten as shown below. In this case the code to execute in response to the event is placed inside a function and then the function is associated with the event.
Events are very useful in real-world applications. For example they can be used to
1. Display confirmation dialog box on submitting a form
2. Form data validation and many more
How to show confirmation dialog in JavaScript
JavaScript form validation example : In this example, both First Name and Last Name fields are required fields. When you type the first character in any of the textbox, the background colour is automatically changed to green. If you delete all the characters you typed or if you leave the textbox without entering any characters the background colour changes to red indicating the field is required. We made this possible using onkeyup and onblur events.
onkeyup occurs when the user releases a key.
onblur occurs when an element loses focus.
Part 33 - Working with dates in javascript
Part 34 - JavaScript timing events
Part 35 - How to create image slideshow using JavaScript
What is an event
An event is a signal from the browser that something has happened. For example,
1. When a user clicks on an HTML element, click event occurs
2. When a user moves the mouse over an HTML element, mouseover event occurs
When events occur, we can execute JavaScript code or functions in response to those events. To do this we need to associate JavaScript code or functions to the events. The function that executes in response to an event is called event handler.
In JavaScript, there are several ways to associate an event handler to the event
1. Using the attributes of an HTML tag
2. Using DOM object property
3. Using special methods
In this video we will discuss associating event handler methods to events using the attributes of HTML tags.
In the following example, the code to execute in response to onmouseover & onmouseout events is set directly in the HTML markup. The keyword "this" references the current element. In this example "this" references the button control.
<input type="button" value="Click me" id="btn"
onmouseover="this.style.background=
'red'; this.style.color = 'yellow'"
onmouseout="this.style.background= 'black';
this.style.color = 'white'" />
The above example, can be rewritten as shown below. In this case the code to execute in response to the event is placed inside a function and then the function is associated with the event.
<input type="button" value="Click me" id="btn"
onmouseover="changeColorOnMouseOver()"
onmouseout="changeColorOnMouseOut()" />
<script type="text/javascript">
function changeColorOnMouseOver()
{
var control = document.getElementById("btn");
control.style.background = 'red';
control.style.color = 'yellow';
}
function changeColorOnMouseOut()
{
var control = document.getElementById("btn");
control.style.background = 'black';
control.style.color = 'white';
}
</script>
Events are very useful in real-world applications. For example they can be used to
1. Display confirmation dialog box on submitting a form
2. Form data validation and many more
How to show confirmation dialog in JavaScript
<input type="submit" value="Submit" id="btn" onclick="return confirmSubmit()" />
<script type="text/javascript">
function confirmSubmit()
{
if (confirm("Are you sure you want
to submit"))
{
alert("You
selected OK");
return true;
}
else
{
return false;
confirm("You
selected cancel");
}
}
</script>
JavaScript form validation example : In this example, both First Name and Last Name fields are required fields. When you type the first character in any of the textbox, the background colour is automatically changed to green. If you delete all the characters you typed or if you leave the textbox without entering any characters the background colour changes to red indicating the field is required. We made this possible using onkeyup and onblur events.
onkeyup occurs when the user releases a key.
onblur occurs when an element loses focus.
<table>
<tr>
<td>
First Name
</td>
<td>
<input type="text" id="txtFirstName"
onkeyup="validateRequiredField('txtFirstName')"
onblur="validateRequiredField('txtFirstName')"/>
</td>
</tr>
<tr>
<td>
Last Name
</td>
<td>
<input type="text" id="txtLastName"
onkeyup="validateRequiredField('txtLastName')"
onblur="validateRequiredField('txtLastName')"/>
</td>
</tr>
</table>
<script type="text/javascript">
function validateRequiredField(controlId)
{
var control = document.getElementById(controlId);
control.style.color = 'white';
if (control.value == "")
{
control.style.background =
'red';
}
else
{
control.style.background =
'green';
}
}
</script>
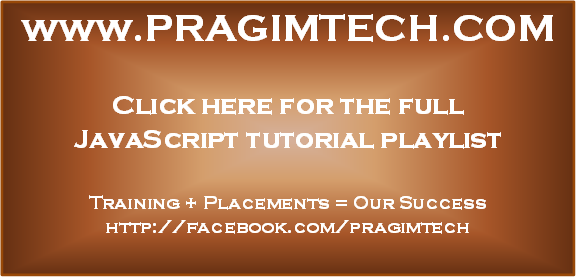
No comments:
Post a Comment
It would be great if you can help share these free resources