Suggested Videos
Part 8 - JavaScript Basics
Part 9 - Converting strings to numbers in JavaScript
Part 10 - Strings in JavaScript
The following are the 3 methods in JavaScript that can be used to retrieve a substring from a given string.
substring()
substr()
slice()
substring() method : This method has 2 parameters start and end. start parameter is required and specifies the position where to start the extraction. end parameter is optional and specifies the position where the extraction should end. The character at the end position is not included in the substring. If the end parameter is not specified, all the characters from the start position till the end of the string are extracted. If the value of start parameter is greater than the value of the end parameter, this method will swap the two arguments. This means start will be used as end and end will be used as start.
Extract the first 10 characters
Output : JavaScript
If the value of start parameter is greater than the value of the end parameter, then start will be used as end and end will be used as start
Output : JavaScript
substr() method : This method has 2 parameters start and count. start parameter is required and specifies the position where to start the extraction. count parameter is optional and specifies the number of characters to extract. If the count parameter is not specified, all the characters from the start position till the end of the string are extracted. If count is 0 or negative, an empty string is returned.
Extract the first 10 characters
Output : JavaScript
If the count parameter is not specified, all the characters from the start position till the end of the string are extracted
Output : Tutorial
slice() method : This method has 2 parameters start and end. start parameter is required and specifies the position where to start the extraction. end parameter is optional and specifies the position where the extraction should end. The character at the end position is not included in the substring. If the end parameter is not specified, all the characters from the start position till the end of the string are extracted.
Extract the first 10 characters
Output : JavaScript
If the end parameter is not specified, all the characters from the start position till the end of the string are extracted
Output : Tutorial
What is the difference between substr and substring methods
The difference is in the second parameter. The second parameter of substring() method specifies the index position where the extraction should stop. The character at the end position is not included in the substring. The second parameter of substr() method specifies the number of characters to return.
Another difference is substr() method does not work in IE8 and earlier versions.
What is the difference between slice and substring
If start parameter is greater than stop parameter, then substring will swap those 2 parameters, where as slice will not swap.
Another method that is very useful when extracting a substring is indexOf() method. This method returns the position of the first occurrence of a specified value in a string. If the specified value is not present then -1 is returned.
Example : Retrieve the index position of @ character in the email
Output : 6
In our next video, we will discuss a simple real time example of where we can use indexOf() and substring() methods
Part 8 - JavaScript Basics
Part 9 - Converting strings to numbers in JavaScript
Part 10 - Strings in JavaScript
The following are the 3 methods in JavaScript that can be used to retrieve a substring from a given string.
substring()
substr()
slice()
substring() method : This method has 2 parameters start and end. start parameter is required and specifies the position where to start the extraction. end parameter is optional and specifies the position where the extraction should end. The character at the end position is not included in the substring. If the end parameter is not specified, all the characters from the start position till the end of the string are extracted. If the value of start parameter is greater than the value of the end parameter, this method will swap the two arguments. This means start will be used as end and end will be used as start.
Extract the first 10 characters
var str = "JavaScript
Tutorial";
var result = str.substring(0, 10);
alert(result);
Output : JavaScript
If the value of start parameter is greater than the value of the end parameter, then start will be used as end and end will be used as start
var str = "JavaScript
Tutorial";
var result = str.substring(10,
0);
alert(result);
Output : JavaScript
substr() method : This method has 2 parameters start and count. start parameter is required and specifies the position where to start the extraction. count parameter is optional and specifies the number of characters to extract. If the count parameter is not specified, all the characters from the start position till the end of the string are extracted. If count is 0 or negative, an empty string is returned.
Extract the first 10 characters
var str = "JavaScript
Tutorial";
var result = str.substr(0, 10);
alert(result);
Output : JavaScript
If the count parameter is not specified, all the characters from the start position till the end of the string are extracted
var str = "JavaScript
Tutorial";
var result = str.substr(11);
alert(result);
Output : Tutorial
slice() method : This method has 2 parameters start and end. start parameter is required and specifies the position where to start the extraction. end parameter is optional and specifies the position where the extraction should end. The character at the end position is not included in the substring. If the end parameter is not specified, all the characters from the start position till the end of the string are extracted.
Extract the first 10 characters
var str = "JavaScript
Tutorial";
var result = str.slice(0, 10);
alert(result);
Output : JavaScript
If the end parameter is not specified, all the characters from the start position till the end of the string are extracted
var str = "JavaScript
Tutorial";
var result = str.slice(11);
alert(result);
Output : Tutorial
What is the difference between substr and substring methods
The difference is in the second parameter. The second parameter of substring() method specifies the index position where the extraction should stop. The character at the end position is not included in the substring. The second parameter of substr() method specifies the number of characters to return.
Another difference is substr() method does not work in IE8 and earlier versions.
What is the difference between slice and substring
If start parameter is greater than stop parameter, then substring will swap those 2 parameters, where as slice will not swap.
Another method that is very useful when extracting a substring is indexOf() method. This method returns the position of the first occurrence of a specified value in a string. If the specified value is not present then -1 is returned.
Example : Retrieve the index position of @ character in the email
var str = "pragim@pragimtech.com";
var result = str.indexOf("@");
alert(result);
Output : 6
In our next video, we will discuss a simple real time example of where we can use indexOf() and substring() methods
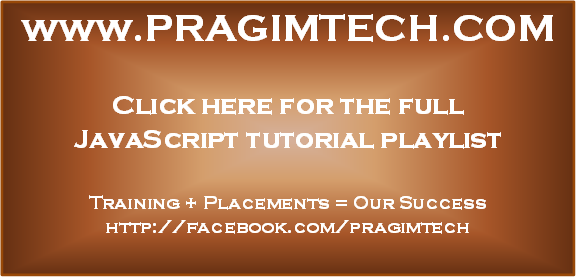
var result=$('input[id="checkbox"]:checked');
ReplyDeleteis this a valid statement if yes why not work it properly otherwise give the valid statement with explanation..
thank you ...