Suggested Videos
Part 7 - Where should the script tag be placed in html
Part 8 - JavaScript Basics
Part 9 - Converting strings to numbers in JavaScript
In this video we will discuss different functions that are available to manipulate strings in JavaScript.
A string is any text inside quotes. You can use either single or double quotes.
Concatenating strings : There are 2 options to concatenate strings in JavaScript. You could either use + operator or concat() method
Example : Concatenating strings using + operator
Output : Hello JavaScript
Example : Concatenating strings using concat() method
Output : Hello JavaScript
If you want single quotes inside a string, there are 2 options
Option 1 : Place your entire string in double quotes, and use single quotes inside the string wherever you need them.
Example :
Output : Welcome to 'JavaScript' Training
Option 2 : If you prefer to place your entire string in single quotes, then use escape sequence character \ with a single quote inside the string.
Example :
Output : Welcome to 'JavaScript' Training
Please Note : You can use the above 2 ways if you need double quotes inside a string
Converting a string to uppercase : Use toUpperCase() method
Example :
Output : JAVASCRIPT
Converting a string to lowercase : Use toLowerCase() method
Example :
Output : javascript
Length of string javascript : Use length property
Example : alert("JavaScript".length);
Output : 10
Example :
Output : 16
Remove whitespace from both ends of a string : Use trim() method
Example :
Output : ABCD
Replacing strings in javascript : Use replace() method. This method searches a given string for a specified value or a regular expression, replaces the specified values with the replacement values and returns a new string. This method does not change the original string.
Example : Replaces JavaScript with World
Output : Hello World
Example : Perform a case-sensitive global replacement. In this example, we are using a regular expression between the 2 forward slashes(//). The letter g after the forward slash specifies a global replacement. The match here is case sensitive. This means Blue(with capital B) is not replaced with green.
Output : A Blue bottle with a green liquid is on a green table
Example : Perform a case-insensitive global replacement. The letters gi after the forward slash indicates to do global case-insensitive replacement. Notice that the word Blue(with capital B) is also replaced with green.
Output : A green bottle with a green liquid is on a green table
Part 7 - Where should the script tag be placed in html
Part 8 - JavaScript Basics
Part 9 - Converting strings to numbers in JavaScript
In this video we will discuss different functions that are available to manipulate strings in JavaScript.
A string is any text inside quotes. You can use either single or double quotes.
var string1 = "string
in double quotes"
var string2 = 'string
in single quotes'
Concatenating strings : There are 2 options to concatenate strings in JavaScript. You could either use + operator or concat() method
Example : Concatenating strings using + operator
var string1 = "Hello"
var string2 = "JavaScript"
var result = string1 + " " + string2;
alert(result);
Output : Hello JavaScript
Example : Concatenating strings using concat() method
var string1 = "Hello"
var string2 = "JavaScript"
var result = string1.concat(" ", string2);
alert(result);
Output : Hello JavaScript
If you want single quotes inside a string, there are 2 options
Option 1 : Place your entire string in double quotes, and use single quotes inside the string wherever you need them.
Example :
var myString = "Welcome
to 'JavaScript' Training";
alert(myString);
Output : Welcome to 'JavaScript' Training
Option 2 : If you prefer to place your entire string in single quotes, then use escape sequence character \ with a single quote inside the string.
Example :
var myString = 'Welcome
to \'JavaScript\' Training';
alert(myString);
Output : Welcome to 'JavaScript' Training
Please Note : You can use the above 2 ways if you need double quotes inside a string
Converting a string to uppercase : Use toUpperCase() method
Example :
var upperCaseString = "JavaScript";
alert(upperCaseString.toUpperCase());
Output : JAVASCRIPT
Converting a string to lowercase : Use toLowerCase() method
Example :
var lowerCaseString = "JavaScript";
alert(lowerCaseString.toLowerCase());
Output : javascript
Length of string javascript : Use length property
Example : alert("JavaScript".length);
Output : 10
Example :
var myString = "Hello
JavaScript";
alert(myString.length);
Output : 16
Remove whitespace from both ends of a string : Use trim() method
Example :
var string1 = "
AB ";
var string2 = "
CD ";
var result = string1.trim() + string2.trim();
alert(result);
Output : ABCD
Replacing strings in javascript : Use replace() method. This method searches a given string for a specified value or a regular expression, replaces the specified values with the replacement values and returns a new string. This method does not change the original string.
Example : Replaces JavaScript with World
var myString = "Hello
JavaScript";
var result = myString.replace("JavaScript", "World");
alert(result);
Output : Hello World
Example : Perform a case-sensitive global replacement. In this example, we are using a regular expression between the 2 forward slashes(//). The letter g after the forward slash specifies a global replacement. The match here is case sensitive. This means Blue(with capital B) is not replaced with green.
var myString = "A
Blue bottle with a blue liquid is on a blue table";
var result = myString.replace(/blue/g,
"green");
alert(result);
Output : A Blue bottle with a green liquid is on a green table
Example : Perform a case-insensitive global replacement. The letters gi after the forward slash indicates to do global case-insensitive replacement. Notice that the word Blue(with capital B) is also replaced with green.
var myString = "A
Blue bottle with a blue liquid is on a blue table";
var result = myString.replace(/blue/gi,
"green");
alert(result);
Output : A green bottle with a green liquid is on a green table
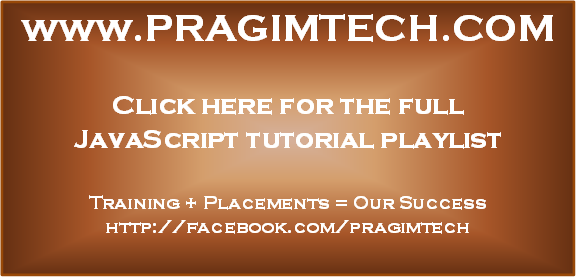
Venkat,
ReplyDeleteCould you please help me in this replace scenario?
In this tutorial you said we can replace a word with /blue/g. In case of dynamic http tag, how could we replace a url string with desired word? for example I have a string that starts with https://...... . This entire url string I want to replace with say Hello world. How can I extract the entire url string starting from https:// and end with unknown as it is dynamic.
The url could be http://www.google.com or http://www.pragim.com or could be any other unknown text.
For special characters which has special meaning in javascript like single quote ,double quote and forward slash(/).if you want to treat them as regular printable characters then we can make use of the escape sequences(\).
Deleteyou can handle the forward slash / with the help of backslash \.use the back slash before the forward slash to treat it as string
for example
var url ="This is google Url http://www.google.com and yahoo URL http://www.yahoo.com"
We have above string and we want to replace the http:// with Hello.
document.write(url.replace(/http:\/\//gi,"Hello"))
var myString = "A Blue bottle with a blue liquid is on a blue table";
ReplyDeletevar name='blue';
var result = myString.replace(/'name'/g, "green");
alert(result);
Hi Venkat,
ReplyDeleteIn recent interview i have faced one question which i could not answer. The questions is what is the difference between below two lines of code.
var name = "Kud Venkat";
var anothername = new String("Kud Venkat");
Can you please explain me, what is the difference between them. Thanks in advance.