Suggested Videos
Part 4 - How to debug javascript in visual studio
Part 5 - Tools for learning JavaScript
Part 6 - Inline vs external javascript
In this video we will discuss, where should the script tag be placed in the html. Should it be placed in the body or head section of the page. In general the script tag can be placed either in the head or body section.
Let's look at a few examples :
Example 1 : Script tag in the head section
Example 2 : Script tag in the body section
In Example 1 we placed the script tag in the head section and in Example 2, we placed it in body section. In both the cases JavaScript works as expected.
Example 3 : Sets the background color of the TextBox to red.
Example 4 : This is same as Example 3, except we moved the script tag to the head section. In this case the script will not work as expected. Depending on the browser you are using you get one of the following JavaScript error.
Firefox - TypeError: document.getElementById(...) is null
Chrome - Uncaught TypeError: Cannot read property 'style' of null
IE - Unable to get property 'style' of undefined or null reference
To see these JavaScript errors press F12 which launches developer tools. F12 works for all the 3 browsers.
Why did the JavaScript did not work in this case
JavaScript code is present before the textbox control. By the time the JavaScript code is executed, the textbox is still not loaded into browser DOM (Document Object Model). This is the reason JavaScript can't find the textbox and throws a NULL reference error.
In Part 6, we discussed that, in general it is a good practice to store JavaScript in an external .js file. So, if the JavaScript is present in an external file and if you are referencing it on a page using <script> element, where should such <script> element be placed.
To answer this question, first let's understand what happens when a browser starts loading a web page.
1. The browser starts parsing the HTML
2. When the parser encounters a <script> tag that references an external JavaScript file. The parser stops parsing the HTML and the browser makes a request to download the script file. Until the download is complete, the parser is blocked from parsing the rest of the HTML on the page.
3. When the download is complete, that's when the parser will resume to parse the rest of the HTML.
This means the page loading is stopped until all the scripts are loaded which affects user experience.
In general, the suggested good practice is to place the <script> tag just before the closing <body> tag, so it doesn't block the HTML parser. However, modern browsers support async and defer attributes on scripts. These attributes tell the browser it's safe to continue parsing while the scripts are being downloaded. But even with these attributes, from a performance standpoint it is still better to place <script> tag just before closing <body> tag.
According to HTTP/1.1 specification browsers download no more than two components in parallel. So, if the page has several images to download and if you place <script> tags at the top of the page, the script files start to download first which blocks the images download which adds to the total page load time.
Part 4 - How to debug javascript in visual studio
Part 5 - Tools for learning JavaScript
Part 6 - Inline vs external javascript
In this video we will discuss, where should the script tag be placed in the html. Should it be placed in the body or head section of the page. In general the script tag can be placed either in the head or body section.
Let's look at a few examples :
Example 1 : Script tag in the head section
<html>
<head>
<script type="text/javascript">
alert("Welcome to
JavaScript Tutorial");
</script>
</head>
<body>
<form id="form1" runat="server">
</form>
</body>
</html>
Example 2 : Script tag in the body section
<html>
<head>
</head>
<body>
<form id="form1" runat="server">
</form>
<script type="text/javascript">
alert("Welcome to
JavaScript Tutorial");
</script>
</body>
</html>
In Example 1 we placed the script tag in the head section and in Example 2, we placed it in body section. In both the cases JavaScript works as expected.
Example 3 : Sets the background color of the TextBox to red.
<html>
<head>
</head>
<body>
<form id="form1" runat="server">
<asp:TextBox ID="TextBox1" runat="server"></asp:TextBox>
</form>
<script type="text/javascript">
document.getElementById("TextBox1").style.backgroundColor
= "red";
</script>
</body>
</html>
Example 4 : This is same as Example 3, except we moved the script tag to the head section. In this case the script will not work as expected. Depending on the browser you are using you get one of the following JavaScript error.
Firefox - TypeError: document.getElementById(...) is null
Chrome - Uncaught TypeError: Cannot read property 'style' of null
IE - Unable to get property 'style' of undefined or null reference
To see these JavaScript errors press F12 which launches developer tools. F12 works for all the 3 browsers.
<html>
<head>
<script type="text/javascript">
document.getElementById("TextBox1").style.backgroundColor
= "red";
</script>
</head>
<body>
<form id="form1" runat="server">
<asp:TextBox ID="TextBox1" runat="server"></asp:TextBox>
</form>
</body>
</html>
Why did the JavaScript did not work in this case
JavaScript code is present before the textbox control. By the time the JavaScript code is executed, the textbox is still not loaded into browser DOM (Document Object Model). This is the reason JavaScript can't find the textbox and throws a NULL reference error.
In Part 6, we discussed that, in general it is a good practice to store JavaScript in an external .js file. So, if the JavaScript is present in an external file and if you are referencing it on a page using <script> element, where should such <script> element be placed.
To answer this question, first let's understand what happens when a browser starts loading a web page.
1. The browser starts parsing the HTML
2. When the parser encounters a <script> tag that references an external JavaScript file. The parser stops parsing the HTML and the browser makes a request to download the script file. Until the download is complete, the parser is blocked from parsing the rest of the HTML on the page.
3. When the download is complete, that's when the parser will resume to parse the rest of the HTML.
This means the page loading is stopped until all the scripts are loaded which affects user experience.
In general, the suggested good practice is to place the <script> tag just before the closing <body> tag, so it doesn't block the HTML parser. However, modern browsers support async and defer attributes on scripts. These attributes tell the browser it's safe to continue parsing while the scripts are being downloaded. But even with these attributes, from a performance standpoint it is still better to place <script> tag just before closing <body> tag.
According to HTTP/1.1 specification browsers download no more than two components in parallel. So, if the page has several images to download and if you place <script> tags at the top of the page, the script files start to download first which blocks the images download which adds to the total page load time.
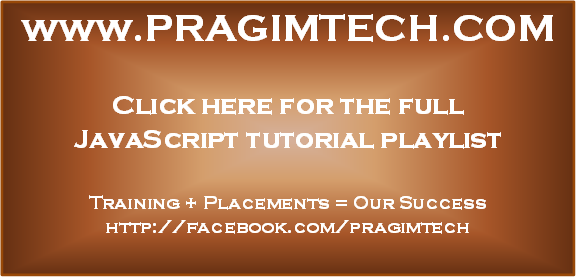
its good to know about the page loading of html n javascript..really helpful information.
ReplyDeleteSir your great like god to us , taking time and explaining us god bless you sir
ReplyDeletesir honestly I cant stop myself to prevent to watchiing your tutorials... honesty looking foorward to get involved in your class
ReplyDeletewhat happens if user makes an action involving javascript execution before javascript file is downloaded
ReplyDeleteI have tried this and its working
ReplyDeletewindow.onload = function () {
document.getElementById("TextBox1").style.backgroundColor = "red";
} so we can apply this as well sir