Suggested Video Tutorials
ADO.NET Tutorial
ASP.NET Tutorial
SQL Server Tutorial
In this video we will discuss
1. The use of JavaScript in a web application.
2. Advantages of using JavaScript
Example : The following web form captures user First Name, Last Name & Email.
When we click the Submit button, we want to save the data to the following SQL Server Users table
Here are the steps
Step 1 : Create Users Table
Step 2 : Create a stored procedure to insert user record into Users table
Step 3 : Create a new empty asp.net web application project. Name it Demo.
Step 4 : In the web.config file include connection string to the database
Step 5 : Add a WebForm to the project. Copy and paste the following HTML in the <form> tag of the webform.
Step 6 : Copy and paste the following code in the code-behind file.
Run the application. Leave all the fields blank and click the Submit button. The form is posted to the web server, the server validates the form and since all the fields are required fields the user is presented with the following error messages.
In this case the form validation is done on the server. Just to validate the form, there is a round trip between the client browser and the web server. The request has to be sent over the network to the web server for processing. This means if the network is slow or if the server is busy processing other requests, the end user may have to wait a few seconds.
Isn't there a way to validate the form on the client side?
Yes, we can and that's when we use JavaScript. JavaScript runs on the client broswer.
Copy and paste the following JavaScript function in the head section of WebForm1.aspx
For the Submit button set OnClientClick="return ValidateForm()". This calls the JavaScript ValidateForm() function. The JavaScript code runs on the client browser and validates the form fields.
If the fields are left blank the user is presented with an error message right away. This is much faster because there is no round trip between the client and the web server. This also means the load on the server is reduced, and we are using the client machine processing power. If all the form fields are populated the form will be submitted to the server and the server saves the data to the database table.
What are the advantages of using JavaScript
1. Form validation can be done on the client side, which reduces the unnecessary round trips between the client and the server. This also means the load on the server is reduced and the application is more responsive.
2. JavaScript uses the client machine processing power.
3. With JavaScript partial page updates are possible i.e only portions of the page can be updated, without reloading the entire web form. This is commonly called as AJAX.
4. JavaScript can also be used to animate elements on a page. For example, show or hide elements and sections of the page.
ADO.NET Tutorial
ASP.NET Tutorial
SQL Server Tutorial
In this video we will discuss
1. The use of JavaScript in a web application.
2. Advantages of using JavaScript
Example : The following web form captures user First Name, Last Name & Email.

When we click the Submit button, we want to save the data to the following SQL Server Users table

Here are the steps
Step 1 : Create Users Table
Create table Users
(
ID int primary
key identity,
FirstName nvarchar(50),
LastName nvarchar(50),
Email nvarchar(50)
)
Step 2 : Create a stored procedure to insert user record into Users table
Create procedure spInsertUser
@FirstName nvarchar(50),
@LastName nvarchar(50),
@Email nvarchar(50)
as
Begin
Insert into
Users values (@FirstName, @LastName, @Email)
End
Step 3 : Create a new empty asp.net web application project. Name it Demo.
Step 4 : In the web.config file include connection string to the database
<add name="CS"
connectionString="server=.;database=Sample;integrated
security=SSPI"/>
Step 5 : Add a WebForm to the project. Copy and paste the following HTML in the <form> tag of the webform.
<table style="border:1px solid black; font-family:Arial">
<tr>
<td>
<b>First Name</b>
</td>
<td>
<asp:TextBox ID="txtFirstName" runat="server"></asp:TextBox>
<asp:Label ID="lblFirstName" runat="server" ForeColor="Red"></asp:Label>
</td>
</tr>
<tr>
<td>
<b>Last Name</b>
</td>
<td>
<asp:TextBox ID="txtLastName" runat="server"></asp:TextBox>
<asp:Label ID="lblLastName" runat="server" ForeColor="Red"></asp:Label>
</td>
</tr>
<tr>
<td>
<b>Email</b>
</td>
<td>
<asp:TextBox ID="txtEmail" runat="server"></asp:TextBox>
<asp:Label ID="lblEmail" runat="server" ForeColor="Red"></asp:Label>
</td>
</tr>
<tr>
<td colspan="2">
<asp:Button ID="btnSubmit" runat="server" Text="Submit" onclick="btnSubmit_Click" />
</td>
</tr>
</table>
Step 6 : Copy and paste the following code in the code-behind file.
using System;
using System.Configuration;
using System.Data;
using System.Data.SqlClient;
namespace Demo
{
public partial class
WebForm1 : System.Web.UI.Page
{
protected void Page_Load(object sender,
EventArgs e)
{ }
protected void btnSubmit_Click(object sender,
EventArgs e)
{
if (ValidateForm())
{
SaveData();
}
}
private void SaveData()
{
string cs = ConfigurationManager.ConnectionStrings["CS"].ConnectionString;
using (SqlConnection con = new SqlConnection(cs))
{
SqlCommand cmd = new SqlCommand("spInsertUser", con);
cmd.CommandType = CommandType.StoredProcedure;
SqlParameter paramFirstName = new
SqlParameter("@FirstName", txtFirstName.Text);
SqlParameter paramLastName = new
SqlParameter("@LastName", txtLastName.Text);
SqlParameter paramEmail = new
SqlParameter("@Email", txtEmail.Text);
cmd.Parameters.Add(paramFirstName);
cmd.Parameters.Add(paramLastName);
cmd.Parameters.Add(paramEmail);
con.Open();
cmd.ExecuteNonQuery();
}
}
private bool ValidateForm()
{
bool ret = true;
//System.Threading.Thread.Sleep(3000);
if (string.IsNullOrEmpty(txtFirstName.Text))
{
ret = false;
lblFirstName.Text = "First Name is required";
}
else
{
lblFirstName.Text = "";
}
if (string.IsNullOrEmpty(txtLastName.Text))
{
ret = false;
lblLastName.Text = "Last Name is required";
}
else
{
lblLastName.Text = "";
}
if (string.IsNullOrEmpty(txtEmail.Text))
{
ret = false;
lblEmail.Text = "Email required";
}
else
{
lblEmail.Text = "";
}
return ret;
}
}
}
Run the application. Leave all the fields blank and click the Submit button. The form is posted to the web server, the server validates the form and since all the fields are required fields the user is presented with the following error messages.

In this case the form validation is done on the server. Just to validate the form, there is a round trip between the client browser and the web server. The request has to be sent over the network to the web server for processing. This means if the network is slow or if the server is busy processing other requests, the end user may have to wait a few seconds.
Isn't there a way to validate the form on the client side?
Yes, we can and that's when we use JavaScript. JavaScript runs on the client broswer.
Copy and paste the following JavaScript function in the head section of WebForm1.aspx
<script type="text/JavaScript" language="JavaScript">
function ValidateForm()
{
var ret = true;
if (document.getElementById("txtFirstName").value
== "")
{
document.getElementById("lblFirstName").innerText = "First
Name is required";
ret = false;
}
else
{
document.getElementById("lblFirstName").innerText = "";
}
if (document.getElementById("txtLastName").value
== "")
{
document.getElementById("lblLastName").innerText = "Last
Name is required";
ret = false;
}
else
{
document.getElementById("lblLastName").innerText = "";
}
if (document.getElementById("txtEmail").value
== "")
{
document.getElementById("lblEmail").innerText = "Email
is required";
ret = false;
}
else
{
document.getElementById("lblEmail").innerText = "";
}
return ret;
}
</script>
For the Submit button set OnClientClick="return ValidateForm()". This calls the JavaScript ValidateForm() function. The JavaScript code runs on the client browser and validates the form fields.
If the fields are left blank the user is presented with an error message right away. This is much faster because there is no round trip between the client and the web server. This also means the load on the server is reduced, and we are using the client machine processing power. If all the form fields are populated the form will be submitted to the server and the server saves the data to the database table.
<asp:Button ID="btnSubmit" runat="server" Text="Submit"
OnClientClick="return ValidateForm()" onclick="btnSubmit_Click" />
What are the advantages of using JavaScript
1. Form validation can be done on the client side, which reduces the unnecessary round trips between the client and the server. This also means the load on the server is reduced and the application is more responsive.
2. JavaScript uses the client machine processing power.
3. With JavaScript partial page updates are possible i.e only portions of the page can be updated, without reloading the entire web form. This is commonly called as AJAX.
4. JavaScript can also be used to animate elements on a page. For example, show or hide elements and sections of the page.
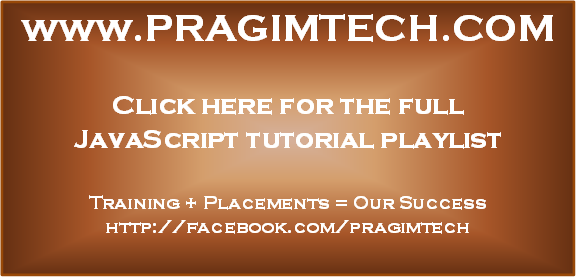
in my pc javascript validation does not working...
ReplyDeletei also try in another pc, but it not working well..
I think in your browser javascript is disabled so enable it(for chrom the process is go to setting and search for javascript click on content setting and choose allow all site to run
Deleteon javascript )
Please upload JQuery videos
ReplyDeleteHi Sir,
ReplyDeletePlease explain us about Angular JS and post the videos.
Thanks
Raghavendra
Hi Sir,
ReplyDeleteYou are helping thousands of people trying to lean JS other stuff. This great act of your are helping many fresher who can not pay for the coaching class and still want to learn and work in this competitive environment, Hats off to you, you will get paid by god for this act of kindness.
Keep it up.
Dear Sir,
ReplyDeleteYour video lessons are helpful to me in great extent, in such a way that creating
self-confidence.
I am ever thankful to you sir.
K.Baburao,Hyderabad.
Your videos are quite helpful to me. I am learning so much from your youtube lessons.
ReplyDeleteThanks a lot.
the java script code doesn't work
ReplyDeletewhat i can do
Thanks for Such a greatfull explanation Please Include a real project to apply all these concepts asp.net etc
ReplyDeleteI am beginner. First of all thank you so much for all your tutorials. I am learning from your tutorials. Thanks.
ReplyDeletein above example i have all the fields plus i have one image field which browse image and save into Database.. but with validation it shows me error"Must declare scalar variable with "@pic". How do i add image in sqlparamter with image in SaveData() ?? any help
hii Sir , recently i face one question related to JS and JQuery , they asked me how to encrypt the JS and JQuery code in browser. means page source and developer tool
ReplyDeleteIn an Interview ,there is a question can we call C# Method using javascript,if yes then how
ReplyDeletehi sir,thank you very much for all tutorials in text format...it's very easy to understand....
ReplyDeleteHi Sir, I tried to add value to the label when I click a button. But the value which I added is not stable. Stable I mean value is appearing when I click and disappearing after page load. I used the client-side validation. What can be the cause? I used above code exactly still issue persist. I have done only client side validation not server side. Is it mandatory to have both side validations ?
ReplyDeleteCould not find stored procedure 'spInsertUser'.
ReplyDelete