Suggested Videos
Part 9 - Eager loading in LINQ to SQL
Part 10 - Difference between eager loading and lazy loading
Part 11 - Single table inheritance in linq to sql
In Part 11, we discussed creating a single table inheritance model and retrieving data. In this video we will discuss saving data to the database table using the single table inheritance model. We will continue with the example we worked with in Part 11.
Drag and drop a button control on the webform and set the following properties
ID="btnAddEmployees"
Text="Add Employees"
Double click on the button control to generate the click event handler. Copy and paste the following code in the code-behind file.
Run the application and click "Add Employees" button
Please note:
1. Since Employee is an abstract class, an instance of this class cannot be created.
2. The Discriminator column in the database is updated depending on the type of the employee object (PermanentEmployee or ContractEmployee) being instantiated.
Part 9 - Eager loading in LINQ to SQL
Part 10 - Difference between eager loading and lazy loading
Part 11 - Single table inheritance in linq to sql
In Part 11, we discussed creating a single table inheritance model and retrieving data. In this video we will discuss saving data to the database table using the single table inheritance model. We will continue with the example we worked with in Part 11.
Drag and drop a button control on the webform and set the following properties
ID="btnAddEmployees"
Text="Add Employees"
Double click on the button control to generate the click event handler. Copy and paste the following code in the code-behind file.
protected void btnAddEmployees_Click(object sender, EventArgs
e)
{
using (SampleDataContext dbContext = new SampleDataContext())
{
PermanentEmployee permanentEmployee = new PermanentEmployee
{
Name = "Emma",
Gender = "Female",
AnuualSalary = 65000
};
ContractEmployee contractEmployee = new ContractEmployee
{
Name = "Kristie",
Gender = "Female",
HourlyPay = 50,
HoursWorked = 80
};
dbContext.Employees.InsertOnSubmit(permanentEmployee);
dbContext.Employees.InsertOnSubmit(contractEmployee);
dbContext.SubmitChanges();
}
}
Run the application and click "Add Employees" button
Please note:
1. Since Employee is an abstract class, an instance of this class cannot be created.
2. The Discriminator column in the database is updated depending on the type of the employee object (PermanentEmployee or ContractEmployee) being instantiated.

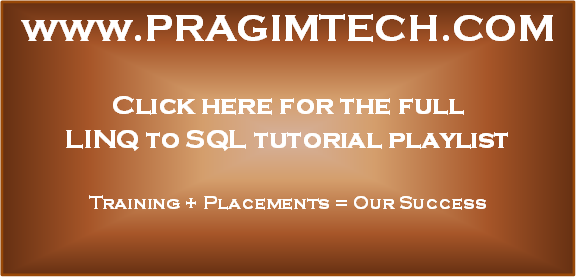
No comments:
Post a Comment
It would be great if you can help share these free resources