Suggested Videos
Part 6 - Restriction Operators in LINQ
Part 7 - Projection Operators in LINQ
Part 8 - SelectMany Operator in LINQ
Let us understand the difference between Select and SelectMany with an example.
We will be using the following Student class in this demo. Subjects property in this class is a collection of strings.
In this example, the Select() method returns List of List<string>. To print all the subjects we will have to use 2 nested foreach loops.
SelectMany() on the other hand, flattens queries that return lists of lists into a single list. So in this case to print all the subjects we have to use just one foreach loop.
Output:
Part 6 - Restriction Operators in LINQ
Part 7 - Projection Operators in LINQ
Part 8 - SelectMany Operator in LINQ
Let us understand the difference between Select and SelectMany with an example.
We will be using the following Student class in this demo. Subjects property in this class is a collection of strings.
public class Student
{
public string Name
{ get; set;
}
public string Gender
{ get; set;
}
public List<string> Subjects { get; set; }
public static List<Student> GetAllStudetns()
{
List<Student> listStudents
= new List<Student>
{
new Student
{
Name = "Tom",
Gender = "Male",
Subjects = new List<string> { "ASP.NET",
"C#" }
},
new Student
{
Name = "Mike",
Gender = "Male",
Subjects = new List<string> { "ADO.NET",
"C#", "AJAX" }
},
new Student
{
Name = "Pam",
Gender = "Female",
Subjects = new List<string> { "WCF",
"SQL Server", "C#" }
},
new Student
{
Name = "Mary",
Gender = "Female",
Subjects = new List<string> { "WPF",
"LINQ", "ASP.NET" }
},
};
return listStudents;
}
}
In this example, the Select() method returns List of List<string>. To print all the subjects we will have to use 2 nested foreach loops.
IEnumerable<List<string>> result = Student.GetAllStudetns().Select(s
=> s.Subjects);
foreach (List<string>
stringList in result)
{
foreach (string str
in stringList)
{
Console.WriteLine(str);
}
}
SelectMany() on the other hand, flattens queries that return lists of lists into a single list. So in this case to print all the subjects we have to use just one foreach loop.
IEnumerable<string>
result = Student.GetAllStudetns().SelectMany(s
=> s.Subjects);
foreach (string str in result)
{
Console.WriteLine(str);
}
Output:
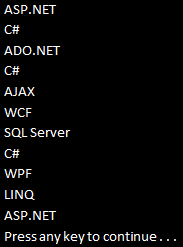
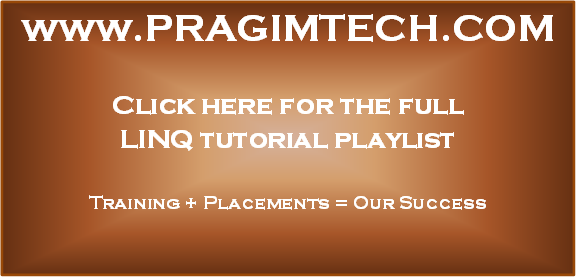
Hi Venkat Sir,
ReplyDeletePlease help us to understand the difference between IEnumerable and IQueryable
The main difference, from a user's perspective, is that, when you use IQueryable (with a provider that supports things correctly), you can save a lot of resources.
ReplyDeleteFor example, if you're working against a remote database, with many ORM systems, you have the option of fetching data from a table in two ways, one which returns IEnumerable, and one which returns an IQueryable. Say, for example, you have a Products table, and you want to get all of the products whose cost is >$25.
If you do:
IEnumerable products = myORM.GetProducts();
var productsOver25 = products.Where(p => p.Cost >= 25.00);
What happens here, is the database loads all of the products, and passes them across the wire to your program. Your program then filters the data. In essense, the database does a "SELECT * FROM Products", and returns EVERY product to you.
With the right IQueryable provider, on the other hand, you can do:
IQueryable products = myORM.GetQueryableProducts();
var productsOver25 = products.Where(p => p.Cost >= 25.00);
The code looks the same, but the difference here is that the SQL executed will be "SELECT * FROM Products WHERE Cost >= 25".
From your POV as a developer, this looks the same. However, from a performance standpoint, you may only return 2 records across the network instead of 20,000....
share|improve this answer
thank you very much !!! Esteban
ReplyDelete