Suggested Videos
Part 10 - Ordering Operators in LINQ
Part 11 - Ordering Operators in LINQ - II
Part 12 - Partitioning Operators
In this video, we will discuss implementing paging using Skip and Take operators in LINQ.
We will use the following Student class in this demo. Notice that, there are 11 total Students. We want to display a maximum of 3 students per page. So there will be 4 total pages. The last page, i.e Page 4 will display the last 2 students.
Here is what we want to do
1. The program should prompt the user to enter a page number. The Page number must be between 1 and 4.
2. If the user does not enter a valid page number, the program should prompt the user to enter a valid page number.
3. Once a valid page number is entered, the program should display the correct set of Students
For example, the output of the program should be as shown below.
The following console application use Skip() and Take() operators to achieve this.
Please Note: The condition in the while loop puts the program in an infinite loop. To end the program, simply close the console window.
Part 10 - Ordering Operators in LINQ
Part 11 - Ordering Operators in LINQ - II
Part 12 - Partitioning Operators
In this video, we will discuss implementing paging using Skip and Take operators in LINQ.
We will use the following Student class in this demo. Notice that, there are 11 total Students. We want to display a maximum of 3 students per page. So there will be 4 total pages. The last page, i.e Page 4 will display the last 2 students.
public class Student
{
public int StudentID
{ get; set;
}
public string Name
{ get; set;
}
public int TotalMarks
{ get; set;
}
public static List<Student> GetAllStudetns()
{
List<Student> listStudents
= new List<Student>
{
new Student { StudentID= 101, Name = "Tom", TotalMarks = 800
},
new Student { StudentID= 102, Name = "Mary", TotalMarks = 900
},
new Student { StudentID= 103, Name = "Pam", TotalMarks = 800
},
new Student { StudentID= 104, Name = "John", TotalMarks = 800
},
new Student { StudentID= 105, Name = "John", TotalMarks = 800
},
new Student { StudentID= 106, Name = "Brian", TotalMarks = 700
},
new Student { StudentID= 107, Name = "Jade", TotalMarks = 750
},
new Student { StudentID= 108, Name = "Ron", TotalMarks = 850
},
new Student { StudentID= 109, Name = "Rob", TotalMarks = 950
},
new Student { StudentID= 110, Name = "Alex", TotalMarks = 750
},
new Student { StudentID= 111, Name = "Susan", TotalMarks = 860
},
};
return listStudents;
}
}
Here is what we want to do
1. The program should prompt the user to enter a page number. The Page number must be between 1 and 4.
2. If the user does not enter a valid page number, the program should prompt the user to enter a valid page number.
3. Once a valid page number is entered, the program should display the correct set of Students
For example, the output of the program should be as shown below.

The following console application use Skip() and Take() operators to achieve this.
using System;
using System.Collections.Generic;
using System.Linq;
namespace Demo
{
class Program
{
public static void Main()
{
IEnumerable<Student>
students = Student.GetAllStudetns();
do
{
Console.WriteLine("Please enter Page Number - 1,2,3 or 4");
int pageNumber = 0;
if (int.TryParse(Console.ReadLine(), out pageNumber))
{
if (pageNumber >= 1
&& pageNumber <= 4)
{
int pageSize = 3;
IEnumerable<Student>
result = students
.Skip((pageNumber - 1)
* pageSize).Take(pageSize);
Console.WriteLine();
Console.WriteLine("Displaying Page " + pageNumber);
foreach (Student student in result)
{
Console.WriteLine(student.StudentID
+ "\t" +
student.Name
+ "\t" + student.TotalMarks);
}
Console.WriteLine();
}
else
{
Console.WriteLine("Page number must be an integer between 1 and
4");
}
}
else
{
Console.WriteLine("Page number must be an integer between 1 and
4");
}
} while (1 == 1);
}
}
}
Please Note: The condition in the while loop puts the program in an infinite loop. To end the program, simply close the console window.
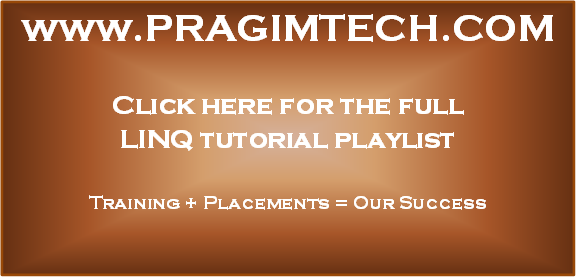
No comments:
Post a Comment
It would be great if you can help share these free resources