Suggested Videos
Part 9 - Difference between Select and SelectMany
Part 10 - Ordering Operators in LINQ
Part 11 - Ordering Operators in LINQ - II
The following 4 standard LINQ query operators belong to Partitioning Operators category
Take
Skip
TakeWhile
SkipWhile
Take method returns a specified number of elements from the start of the collection. The number of items to return is specified using the count parameter this method expects.
Skip method skips a specified number of elements in a collection and then returns the remaining elements. The number of items to skip is specified using the count parameter this method expects.
Please Note: For the same argument value, the Skip method returns all of the items that the Take method would not return.
TakeWhile method returns elements from a collection as long as the given condition specified by the predicate is true.
SkipWhile method skips elements in a collection as long as the given condition specified by the predicate is true, and then returns the remaining elements.
Example 1: Retrieves only the first 3 countries of the array.
Output:
Example 2: Rewrite Example 1 using SQL like syntax
Example 3: Skips the first 3 countries and retrieves the rest of them
Output:
Example 4: Return countries starting from the beginning of the array until a country name is hit that does not have length greater than 2 characters.
Output:
Example 5: Skip elements starting from the beginning of the array, until a country name is hit that does not have length greater than 2 characters, and then return the remaining elements.
Output:
Part 9 - Difference between Select and SelectMany
Part 10 - Ordering Operators in LINQ
Part 11 - Ordering Operators in LINQ - II
The following 4 standard LINQ query operators belong to Partitioning Operators category
Take
Skip
TakeWhile
SkipWhile
Take method returns a specified number of elements from the start of the collection. The number of items to return is specified using the count parameter this method expects.
Skip method skips a specified number of elements in a collection and then returns the remaining elements. The number of items to skip is specified using the count parameter this method expects.
Please Note: For the same argument value, the Skip method returns all of the items that the Take method would not return.
TakeWhile method returns elements from a collection as long as the given condition specified by the predicate is true.
SkipWhile method skips elements in a collection as long as the given condition specified by the predicate is true, and then returns the remaining elements.
Example 1: Retrieves only the first 3 countries of the array.
string[] countries = { "Australia",
"Canada", "Germany", "US", "India",
"UK", "Italy" };
IEnumerable<string>
result = countries.Take(3);
foreach (string country in result)
{
Console.WriteLine(country);
}
Output:
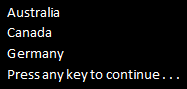
Example 2: Rewrite Example 1 using SQL like syntax
string[] countries = { "Australia",
"Canada", "Germany", "US", "India",
"UK", "Italy" };
IEnumerable<string>
result = (from country
in countries
select country).Take(3);
foreach (string country in result)
{
Console.WriteLine(country);
}
Example 3: Skips the first 3 countries and retrieves the rest of them
string[] countries = { "Australia",
"Canada", "Germany", "US", "India",
"UK", "Italy" };
IEnumerable<string>
result = countries.Skip(3);
foreach (string country in result)
{
Console.WriteLine(country);
}
Output:

Example 4: Return countries starting from the beginning of the array until a country name is hit that does not have length greater than 2 characters.
string[] countries = { "Australia",
"Canada", "Germany", "US", "India",
"UK", "Italy" };
IEnumerable<string>
result = countries.TakeWhile(s => s.Length
> 2);
foreach (string country in result)
{
Console.WriteLine(country);
}
Output:

Example 5: Skip elements starting from the beginning of the array, until a country name is hit that does not have length greater than 2 characters, and then return the remaining elements.
string[] countries = { "Australia",
"Canada", "Germany", "US", "India",
"UK", "Italy" };
IEnumerable<string>
result = countries.SkipWhile(s => s.Length
> 2);
foreach (string country in result)
{
Console.WriteLine(country);
}
Output:
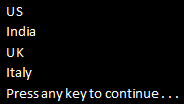
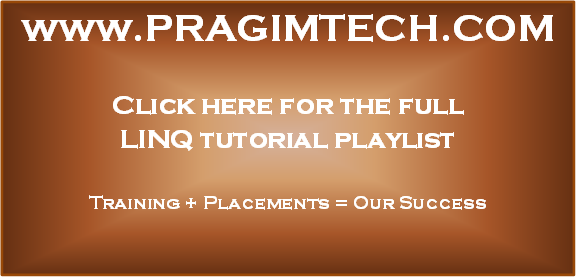
Hii Kudvenkat Sir,
ReplyDeleteYour sharing related to .NET to so nice. I really enjoy and helpful to learning more. I want to learn related SharePoint in Asp.Net. I search on google its just find thoery, but doesn`t find too much of practical example. Please Can you share video related to sharepoint that help to learn..??