Suggested Videos
Part 3 - Entity Framework Code First Approach
Part 4 - Customizing table & column names
Part 5 - How to handle model changes in entity framework
So far in this video series, we have been manually populating the database with test data using the insert sql script. Entity Framework can automate this. We will be working with the example we worked with in Part 5. Here are the steps.
Step 1: Right click on the project in solution explorer and add a class file with name = EmployeeDBContextSeeder.cs
Step 2: Copy and paste the following code in EmployeeDBContextSeeder.cs file
Step 3: Copy and paste the following line in Application_Start() method Global.asax file
Step 4: Remove the following Table and Column attributes from Employee.cs file.
[Table("tblEmployees")]
[Column("First_Name")]
At this point Employee class should look as shown below
Step 5: Run the application and notice that the Sample database, Departments and Employees tables are created and populated with test data automatically.
Part 3 - Entity Framework Code First Approach
Part 4 - Customizing table & column names
Part 5 - How to handle model changes in entity framework
So far in this video series, we have been manually populating the database with test data using the insert sql script. Entity Framework can automate this. We will be working with the example we worked with in Part 5. Here are the steps.
Step 1: Right click on the project in solution explorer and add a class file with name = EmployeeDBContextSeeder.cs
Step 2: Copy and paste the following code in EmployeeDBContextSeeder.cs file
using System.Collections.Generic;
using System.Data.Entity;
namespace EntityFrameworkDemo
{
public class EmployeeDBContextSeeder :
DropCreateDatabaseIfModelChanges<EmployeeDBContext>
{
protected override void Seed(EmployeeDBContext context)
{
Department department1 = new Department()
{
Name = "IT",
Location = "New York",
Employees = new List<Employee>()
{
new Employee()
{
FirstName = "Mark",
LastName = "Hastings",
Gender = "Male",
Salary = 60000,
JobTitle = "Developer"
},
new Employee()
{
FirstName = "Ben",
LastName = "Hoskins",
Gender = "Male",
Salary = 70000,
JobTitle = "Sr. Developer"
},
new Employee()
{
FirstName = "John",
LastName = "Stanmore",
Gender = "Male",
Salary = 80000,
JobTitle = "Project Manager"
}
}
};
Department department2 = new Department()
{
Name = "HR",
Location = "London",
Employees = new List<Employee>()
{
new Employee()
{
FirstName = "Philip",
LastName = "Hastings",
Gender = "Male",
Salary = 45000,
JobTitle = "Recruiter"
},
new Employee()
{
FirstName = "Mary",
LastName = "Lambeth",
Gender = "Female",
Salary = 30000,
JobTitle = "Sr.
Recruiter"
}
}
};
Department department3 = new Department()
{
Name = "Payroll",
Location = "Sydney",
Employees = new List<Employee>()
{
new Employee()
{
FirstName = "Steve",
LastName = "Pound",
Gender = "Male",
Salary = 45000,
JobTitle = "Sr. Payroll Admin",
},
new Employee()
{
FirstName = "Valarie",
LastName = "Vikings",
Gender = "Female",
Salary = 35000,
JobTitle = "Payroll Admin",
}
}
};
context.Departments.Add(department1);
context.Departments.Add(department2);
context.Departments.Add(department3);
base.Seed(context);
}
}
}
Step 3: Copy and paste the following line in Application_Start() method Global.asax file
Database.SetInitializer(new EmployeeDBContextSeeder());
Step 4: Remove the following Table and Column attributes from Employee.cs file.
[Table("tblEmployees")]
[Column("First_Name")]
At this point Employee class should look as shown below
public class Employee
{
public int Id
{ get; set;
}
public string FirstName
{ get; set;
}
public string LastName
{ get; set;
}
public string Gender
{ get; set;
}
public int Salary
{ get; set;
}
public int DepartmentId
{ get; set;
}
[ForeignKey("DepartmentId")]
public Department Department { get; set; }
public string JobTitle
{ get; set;
}
}
Step 5: Run the application and notice that the Sample database, Departments and Employees tables are created and populated with test data automatically.
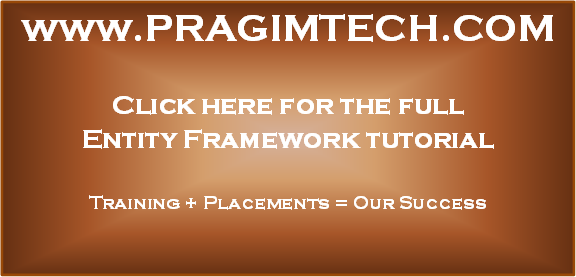
Can you please suggest me where it specifies, ID column is Primary key and identity column
ReplyDeleteno need to specify.. its autogenrated by sql server... Idendity
DeleteOK. It's working fine. What the problem is after executing above code if I add a new employee entry in any department then that new employee's entry is not saving into the database table. Why?
ReplyDeleteBut if I manually drop the database then it displays the record but it's wrong way. How to do this?
In some scenario, Seed method is not working, At this time we need to do below changes in global.asax file
ReplyDeleteprotected void Application_Start(object sender, EventArgs e)
{
Database.SetInitializer(new EmployeeDBContextSeeder());
var db = new EmployeeDBContext();
db.Database.Initialize(true);
}
If your code doesn't work, it's because here seeder class is inheriting from 'DropCreateDatabaseIfModelChanges', so if you don't change your entity class each time in any form e.g. adding new property like 'JobTitle', it won't recreate the database.
DeleteSo to solve the problem you can make seeder class inherit from 'DropCreateDatabaseAlaways' instead.