Suggested Videos
Part 30 - Advantages and disadvantages of hosting WCF service in IIS
Part 31 - WAS hosting in WCF
Part 32 - Message Exchange Patterns in WCF
In this video we will discuss OneWay Message Exchange Pattern in WCF
This is continuation to Part 32. Please watch Part 32 before proceeding.
In a Request-Reply pattern, the client sends a message to the WCF service and then waits for the reply message, even if the service operation's return type is void.
In case of One-Way operation, only one message is exchanged between the client and the service. The client makes a call to the service method, but does not wait for a response message. So, in short, the receiver of the message does not send a reply message, nor does the sender of the message expects one.
As messages are exchanged only in one way, faults if any while processing the request does not get reported.
Clients are unaware of the server channel faults until a subsequent call is made.
An exception will be thrown, if operations marked with IsOneWay=true declares output parameters, by-reference parameters or return value.
Are OneWay calls same as asynchronous calls?
No, they are not. When a oneway call is received at the service, and if the service is busy serving other requests, then the call gets queued and the client is unblocked and can continue executing while the service processes the operation in the background. One-way calls can still block the client, if the number of messages waiting to be processed has exceeded the server queue limit. So, OneWay calls are not asynchronous calls, they just appear to be asynchronous.
To make an operation one-way, set IsOneWay=true.
Service Implementation:
Client code:
Part 30 - Advantages and disadvantages of hosting WCF service in IIS
Part 31 - WAS hosting in WCF
Part 32 - Message Exchange Patterns in WCF
In this video we will discuss OneWay Message Exchange Pattern in WCF
This is continuation to Part 32. Please watch Part 32 before proceeding.
In a Request-Reply pattern, the client sends a message to the WCF service and then waits for the reply message, even if the service operation's return type is void.
In case of One-Way operation, only one message is exchanged between the client and the service. The client makes a call to the service method, but does not wait for a response message. So, in short, the receiver of the message does not send a reply message, nor does the sender of the message expects one.
As messages are exchanged only in one way, faults if any while processing the request does not get reported.
Clients are unaware of the server channel faults until a subsequent call is made.
An exception will be thrown, if operations marked with IsOneWay=true declares output parameters, by-reference parameters or return value.
Are OneWay calls same as asynchronous calls?
No, they are not. When a oneway call is received at the service, and if the service is busy serving other requests, then the call gets queued and the client is unblocked and can continue executing while the service processes the operation in the background. One-way calls can still block the client, if the number of messages waiting to be processed has exceeded the server queue limit. So, OneWay calls are not asynchronous calls, they just appear to be asynchronous.
To make an operation one-way, set IsOneWay=true.
[OperationContract(IsOneWay = true)]
string OneWayOperation();
[OperationContract(IsOneWay = true)]
void OneWayOperation_ThrowsException();
Service Implementation:
public string OneWayOperation()
{
Thread.Sleep(2000);
return "";
}
public void OneWayOperation_ThrowsException()
{
throw new NotImplementedException();
}
private void btnOneWayOperation_Click(object sender, EventArgs e)
{
try
{
listBox1.Items.Add("OneWay
Operation Started @ "
+ DateTime.Now.ToString());
btnOneWayOperation.Enabled = false;
client.OneWayOperation();
btnOneWayOperation.Enabled = true;
listBox1.Items.Add("OneWay
Operation Completed @ "
+ DateTime.Now.ToString());
listBox1.Items.Add("");
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
private void btnOneWayOperation_ThrowsException_Click(object sender, EventArgs e)
{
try
{
listBox1.Items.Add("OneWay
Throws Exception Operation Started @ "
+ DateTime.Now.ToString());
client.OneWayOperation_ThrowsException();
listBox1.Items.Add("OneWay
Throws Exception Operation Completed @ "
+ DateTime.Now.ToString());
listBox1.Items.Add("");
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
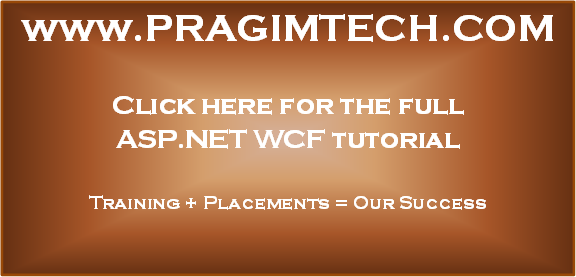
Can you please try to explain Payment Gateway with a real time example.
ReplyDeleteLooks like you took string as return Type and declared IsOneWay=true, which actually leads to error
ReplyDeleteHi Venkat,
ReplyDeleteI tried the Oneway with exception using netTcpBinding. But I did not get the server channel faulted exception and client side exception in the 2nd and 3rd time. Could you please tell what is the issue behind this?