Suggested Videos
Part 1 - Introduction to asp.net web services
This is continuation to Part 1. Please watch Part 1 from ASP.NET Web Services tutorial before proceeding.
Here are the steps to consume the web service that we have created in Part 1.
Step 1: Right click on WebServicesDemo solution in solution explorer and add new asp.net web application project and name it CalculatorWebApplication.
Step 2: Now we need to add a reference to web service. To achieve this
a) Right click on References folder in the CalculatorWebApplication project and select Add Service Reference option
b) In the Address textbox of the Add Service Reference window, type the web service address and click GO button. In the namespace textbox type CalculatorService and click OK.
Step 3: Right click on CalculatorWebApplication project in solution explorer and add new webform.
Step 3: Copy and paste the following HTML
<table style="font-family: Arial">
<tr>
<td>
<b>First Number</b>
</td>
<td>
<asp:TextBox ID="txtFirstNumber" runat="server"></asp:TextBox>
</td>
</tr>
<tr>
<td>
<b>Second Number</b>
</td>
<td>
<asp:TextBox ID="txtSecondNumber" runat="server"></asp:TextBox>
</td>
</tr>
<tr>
<td>
<b>Result</b>
</td>
<td>
<asp:Label ID="lblResult" runat="server"></asp:Label>
</td>
</tr>
<tr>
<td colspan="2">
<asp:Button ID="btnAdd" runat="server" Text="Add"
OnClick="btnAdd_Click" />
</td>
</tr>
</table>
Step 4: Copy and paste the following code in the code-behind file
protected void btnAdd_Click(object sender, EventArgs e)
{
CalculatorService.CalculatorWebServicesSoapClient client =
new CalculatorService.CalculatorWebServicesSoapClient();
int result = client.Add(Convert.ToInt32(txtFirstNumber.Text),
Convert.ToInt32(txtSecondNumber.Text));
lblResult.Text = result.ToString();
}
In an interview the interviewer may ask you the following questions related to consuming a web service.
1. What is WSDL and what is it's purpose
2. How is a proxy class generated
3. What is the use of a proxy class
4. What actually happens when a web service reference is added
The following 2 paragraphs should provide the answers for the above questions
Visual studio generates a proxy class using the WSDL (Web Service Description Language) document of the web service. The WSDL document formally defines a web service. It contains
1. All the methods that are exposed by the web service
2. The parameters and their types
3. The return types of the methods
This information is then used by visual studio to create the proxy class. The client application calls the proxy class method. The proxy class will then serialize the parameters, prepares a SOAP request message and sends it to the web service. The web service executes the method and returns a SOAP response message to the proxy. The proxy class will then deserialize the SOAP response message and hands it the client application. We don't have to serialize or deserialize dot net CLR objects to and from SOAP format. The proxy class takes care of serialization and deserialization and makes the life of a developer much easier.
Part 1 - Introduction to asp.net web services
This is continuation to Part 1. Please watch Part 1 from ASP.NET Web Services tutorial before proceeding.
Here are the steps to consume the web service that we have created in Part 1.
Step 1: Right click on WebServicesDemo solution in solution explorer and add new asp.net web application project and name it CalculatorWebApplication.
Step 2: Now we need to add a reference to web service. To achieve this
a) Right click on References folder in the CalculatorWebApplication project and select Add Service Reference option
b) In the Address textbox of the Add Service Reference window, type the web service address and click GO button. In the namespace textbox type CalculatorService and click OK.
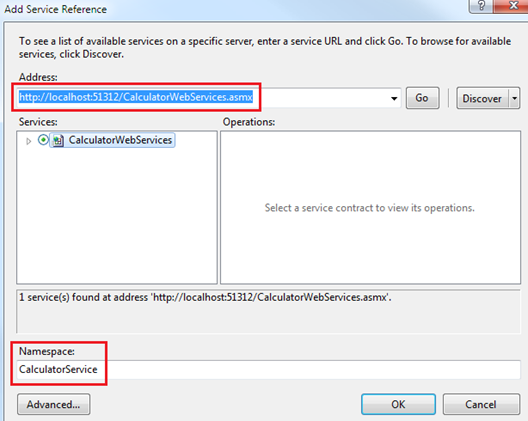
Step 3: Right click on CalculatorWebApplication project in solution explorer and add new webform.
Step 3: Copy and paste the following HTML
<table style="font-family: Arial">
<tr>
<td>
<b>First Number</b>
</td>
<td>
<asp:TextBox ID="txtFirstNumber" runat="server"></asp:TextBox>
</td>
</tr>
<tr>
<td>
<b>Second Number</b>
</td>
<td>
<asp:TextBox ID="txtSecondNumber" runat="server"></asp:TextBox>
</td>
</tr>
<tr>
<td>
<b>Result</b>
</td>
<td>
<asp:Label ID="lblResult" runat="server"></asp:Label>
</td>
</tr>
<tr>
<td colspan="2">
<asp:Button ID="btnAdd" runat="server" Text="Add"
OnClick="btnAdd_Click" />
</td>
</tr>
</table>
Step 4: Copy and paste the following code in the code-behind file
protected void btnAdd_Click(object sender, EventArgs e)
{
CalculatorService.CalculatorWebServicesSoapClient client =
new CalculatorService.CalculatorWebServicesSoapClient();
int result = client.Add(Convert.ToInt32(txtFirstNumber.Text),
Convert.ToInt32(txtSecondNumber.Text));
lblResult.Text = result.ToString();
}
In an interview the interviewer may ask you the following questions related to consuming a web service.
1. What is WSDL and what is it's purpose
2. How is a proxy class generated
3. What is the use of a proxy class
4. What actually happens when a web service reference is added
The following 2 paragraphs should provide the answers for the above questions
Visual studio generates a proxy class using the WSDL (Web Service Description Language) document of the web service. The WSDL document formally defines a web service. It contains
1. All the methods that are exposed by the web service
2. The parameters and their types
3. The return types of the methods
This information is then used by visual studio to create the proxy class. The client application calls the proxy class method. The proxy class will then serialize the parameters, prepares a SOAP request message and sends it to the web service. The web service executes the method and returns a SOAP response message to the proxy. The proxy class will then deserialize the SOAP response message and hands it the client application. We don't have to serialize or deserialize dot net CLR objects to and from SOAP format. The proxy class takes care of serialization and deserialization and makes the life of a developer much easier.
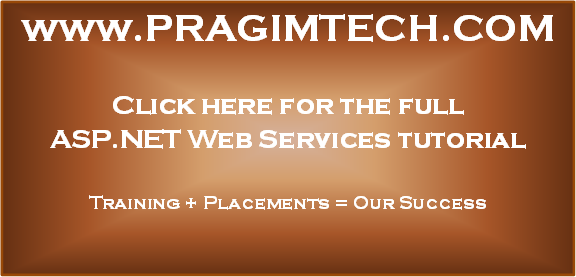
Thank your Sir... It was awesome and crystal clear...
ReplyDeleteSir, In an interview I was asked to called a webservice from the Web form without reloading the Web page(means a post request), is it possible..
I answered using a AJAX wrapping a control in updatepanel . In response to it he said its also a partial postback..
Hi, You can call Web Service using ajax method using Javascript or Jquery.
DeleteThe method which you will called that should be [Web Method].
Example:
[Web Method]
Public void Add()
{
}
Thanks & Regards
Shailesh Kumar
Where can i found WSDL Document???
ReplyDeleteHi Sir,
ReplyDeleteActually I need to call a soap web service through the code and pass the parameter to it and get the response and display it on the screen(asp.net). But i dont want to use the Service Reference method to call the web service. I want to make call through the code. And I dont have any idea of in which technology the service is created. I only have the URL or service API. How can i do that?
System.Net.Sockets.SocketException: No connection could be made because the target machine actively refused it 127.0.0.1:49161
ReplyDelete