Suggested Videos:
Part 6 - Real time example of recursion
Part 7 - Storing different list types in a single generic list
Part 8 - Can an abstract class have a constructor
Can you call an abstract method from an abstract class constructor? If so, what is the use of it?
Yes, an abstract method can be called from an abstract class constructor. Here is an example.
public class Program
{
public static void Main()
{
CorporateCustomer CC = new CorporateCustomer();
SavingsCustomer SC = new SavingsCustomer();
}
}
public abstract class Customer
{
protected Customer()
{
Print();
}
public abstract void Print();
}
public class CorporateCustomer : Customer
{
public override void Print()
{
Console.WriteLine("CorporateCustomer Print() method called");
}
}
public class SavingsCustomer : Customer
{
public override void Print()
{
Console.WriteLine("SavingsCustomer Print() method called");
}
}
An abstract method in an abstract class does not have any implementation, so what is the use of calling it from the abstract class constructor?
If you want the abstract method to be invoked automatically whenever an instance of the class that is derived from the abstract class is created, then we would call it in the constructor of the abstract class.
Part 6 - Real time example of recursion
Part 7 - Storing different list types in a single generic list
Part 8 - Can an abstract class have a constructor
Can you call an abstract method from an abstract class constructor? If so, what is the use of it?
Yes, an abstract method can be called from an abstract class constructor. Here is an example.
public class Program
{
public static void Main()
{
CorporateCustomer CC = new CorporateCustomer();
SavingsCustomer SC = new SavingsCustomer();
}
}
public abstract class Customer
{
protected Customer()
{
Print();
}
public abstract void Print();
}
public class CorporateCustomer : Customer
{
public override void Print()
{
Console.WriteLine("CorporateCustomer Print() method called");
}
}
public class SavingsCustomer : Customer
{
public override void Print()
{
Console.WriteLine("SavingsCustomer Print() method called");
}
}
An abstract method in an abstract class does not have any implementation, so what is the use of calling it from the abstract class constructor?
If you want the abstract method to be invoked automatically whenever an instance of the class that is derived from the abstract class is created, then we would call it in the constructor of the abstract class.
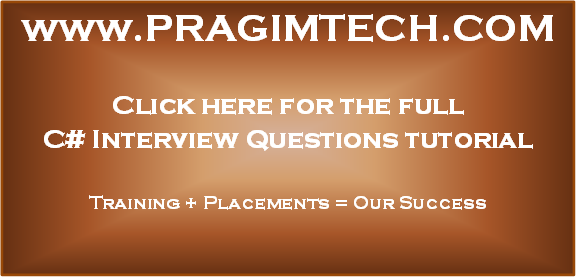
When you said, the constructor should be protected, it totally makes sense, as it is only called when a child class instance is created.
ReplyDeleteWhy do we even need any public variables or public abstract methods in abstract class, everything could be protected in the place of public?