Suggested Videos:
Part 5 - Recursive function c# example
Part 6 - Real time example of recursion
Part 7 - Storing different list types in a single generic list
Can an abstract class have a constructor? If so what is the use?
Yes, an abstract class can have a constructor. In general, a class constructor is used to initialise fields. Along the same lines, an abstract class constructor is used to initialise fields of the abstract class. You would provide a constructor for an abstract class if you want to initialise certain fields of the abstract class before the instantiation of a child-class takes place. An abstract class constructor can also be used to execute code that is relevant for every child class. This prevents duplicate code.
Before an instance of CorporateCustomer or SavingsCustomer class is created, a Globally Unique Identifier (GUID) must be stored in the _id field of the abstract class. This can be achieved using abstract class constructor as shown below. Since initialisation is done in the base abstract Customer class, we don't have to duplicate this logic in CorporateCustomer and SavingsCustomer classes.
public class Program
{
public static void Main()
{
CorporateCustomer CC = new CorporateCustomer();
Console.WriteLine(CC.Id);
SavingsCustomer SC = new SavingsCustomer();
Console.WriteLine(SC.Id);
}
}
public abstract class Customer
{
public Customer()
{
this._id = Guid.NewGuid();
}
public Guid Id
{
get
{
return this._id;
}
}
private Guid _id;
// Other abstract members
// public abstract void Save();
}
public class CorporateCustomer : Customer
{
}
public class SavingsCustomer : Customer
{
}
If we were to move the initialisation code to respective derived classes, the design would look as shown below. Notice the amount of duplicate code.
public abstract class Customer
{
public abstract Guid Id { get; }
// Other abstract members
// public abstract void Save();
}
public class CorporateCustomer : Customer
{
public CorporateCustomer()
{
this._id = Guid.NewGuid();
}
private Guid _id;
public override Guid Id
{
get { return this._id; }
}
}
public class SavingsCustomer : Customer
{
public SavingsCustomer()
{
this._id = Guid.NewGuid();
}
private Guid _id;
public override Guid Id
{
get { return this._id; }
}
}
You cannot create an instance of an abstract class. So, what is the use of a constructor in an abstract class?
Though you cannot create an instance of an abstract class, we can create instances of the classes that are derived from the abstract class. So, when an instance of derived class is created, the parent abstract class constructor is automatically called.
Note: Abstract classes can't be directly instantiated. The abstract class constructor gets executed thru a derived class. So, it is a good practice to use protected access modifier with abstract class constructor. Using public doesn’t make sense.
Part 5 - Recursive function c# example
Part 6 - Real time example of recursion
Part 7 - Storing different list types in a single generic list
Can an abstract class have a constructor? If so what is the use?
Yes, an abstract class can have a constructor. In general, a class constructor is used to initialise fields. Along the same lines, an abstract class constructor is used to initialise fields of the abstract class. You would provide a constructor for an abstract class if you want to initialise certain fields of the abstract class before the instantiation of a child-class takes place. An abstract class constructor can also be used to execute code that is relevant for every child class. This prevents duplicate code.
Before an instance of CorporateCustomer or SavingsCustomer class is created, a Globally Unique Identifier (GUID) must be stored in the _id field of the abstract class. This can be achieved using abstract class constructor as shown below. Since initialisation is done in the base abstract Customer class, we don't have to duplicate this logic in CorporateCustomer and SavingsCustomer classes.
public class Program
{
public static void Main()
{
CorporateCustomer CC = new CorporateCustomer();
Console.WriteLine(CC.Id);
SavingsCustomer SC = new SavingsCustomer();
Console.WriteLine(SC.Id);
}
}
public abstract class Customer
{
public Customer()
{
this._id = Guid.NewGuid();
}
public Guid Id
{
get
{
return this._id;
}
}
private Guid _id;
// Other abstract members
// public abstract void Save();
}
public class CorporateCustomer : Customer
{
}
public class SavingsCustomer : Customer
{
}
If we were to move the initialisation code to respective derived classes, the design would look as shown below. Notice the amount of duplicate code.
public abstract class Customer
{
public abstract Guid Id { get; }
// Other abstract members
// public abstract void Save();
}
public class CorporateCustomer : Customer
{
public CorporateCustomer()
{
this._id = Guid.NewGuid();
}
private Guid _id;
public override Guid Id
{
get { return this._id; }
}
}
public class SavingsCustomer : Customer
{
public SavingsCustomer()
{
this._id = Guid.NewGuid();
}
private Guid _id;
public override Guid Id
{
get { return this._id; }
}
}
You cannot create an instance of an abstract class. So, what is the use of a constructor in an abstract class?
Though you cannot create an instance of an abstract class, we can create instances of the classes that are derived from the abstract class. So, when an instance of derived class is created, the parent abstract class constructor is automatically called.
Note: Abstract classes can't be directly instantiated. The abstract class constructor gets executed thru a derived class. So, it is a good practice to use protected access modifier with abstract class constructor. Using public doesn’t make sense.
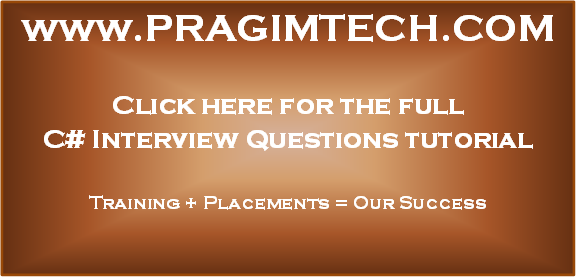
Hi Venkat,your videos are very use full.Please make a videos on WCF and Design Patterns.thanks
ReplyDeleteHi Venkat, I agree with Odelu.
ReplyDeleteHi Venkat,We are very lucky to have a tutor like u..!!! Really appreciate your efforts. :)
ReplyDeleteplease make vedio for node js
ReplyDelete