Suggested Videos:
Part 3 - Why and when should we use an abstract class
Part 4 - What are the advantages of using interfaces
Part 5 - Recursive function c# example
This is continuation to Part 5. Please watch Part 5 from C# Interview Questions tutorial before proceeding.
Probably in an interview, an interviewer may ask you to give an example, where you have used recursion in your project.
To find all the files in a folder and in all the sub-folders in the hierarchy. This is a very good example of where we could use recursion.
Please make sure to include the following using declaration.
using System.IO;
public class Program
{
public static void Main()
{
// Prompt the user to enter folder path
Console.WriteLine("Please enter folder path");
// Read the path from the console
string folderPath = Console.ReadLine();
// Invoke FindFiles() recursive function
FindFiles(folderPath);
}
private static void FindFiles(string path)
{
// Loop thru each file in the current directory and print it's name
foreach (string fileName in Directory.GetFiles(path))
{
Console.WriteLine(fileName);
}
// If there is a subdirectory in the current directory, then recursively
// call FindFiles() method. The recursion will break when the innermost
// directory with no subdirectory is found.
foreach (string directory in Directory.GetDirectories(path))
{
// Notice that FindFiles() is calling itself
FindFiles(directory);
}
}
}
Part 3 - Why and when should we use an abstract class
Part 4 - What are the advantages of using interfaces
Part 5 - Recursive function c# example
This is continuation to Part 5. Please watch Part 5 from C# Interview Questions tutorial before proceeding.
Probably in an interview, an interviewer may ask you to give an example, where you have used recursion in your project.
To find all the files in a folder and in all the sub-folders in the hierarchy. This is a very good example of where we could use recursion.

Please make sure to include the following using declaration.
using System.IO;
public class Program
{
public static void Main()
{
// Prompt the user to enter folder path
Console.WriteLine("Please enter folder path");
// Read the path from the console
string folderPath = Console.ReadLine();
// Invoke FindFiles() recursive function
FindFiles(folderPath);
}
private static void FindFiles(string path)
{
// Loop thru each file in the current directory and print it's name
foreach (string fileName in Directory.GetFiles(path))
{
Console.WriteLine(fileName);
}
// If there is a subdirectory in the current directory, then recursively
// call FindFiles() method. The recursion will break when the innermost
// directory with no subdirectory is found.
foreach (string directory in Directory.GetDirectories(path))
{
// Notice that FindFiles() is calling itself
FindFiles(directory);
}
}
}
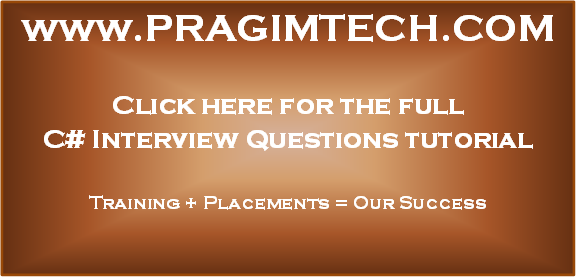
Hi Venkat,
ReplyDeleteYour tutorials are awesome. Can you post video on Design patterns?
Venkat Sir
ReplyDeleteThank you so much for all these helpful videos.
Sir if Possible please share some videos related:
1. AJAX
2. WPF
3. Silverlight
4. Azure
Thanks & Regards