Suggested Videos
Part 89 - Remote validation in asp.net mvc
Part 90 - Remote validation in mvc when javascript is disabled
Part 91 - Create a custom remote attribute and override IsValid() method
ASP.NET AJAX enables a Web application to retrieve data from the server asynchronously and to update portions of the existing page. So these, partial page updates make web application more responsive and hence improves user experience.
In this video, let's discus using Ajax.ActionLink helper. By the end of this video, we should be able to load either
1. All students or
2. Top 3 students or
3. Bottom 3 students
depending on the link we have clicked.
Here are the steps to achieve this
Step 1: Sql script to create and populate table tblStudents
Create table tblStudents
(
Id int identity primary key,
Name nvarchar(50),
TotalMarks int
)
Insert into tblStudents values ('Mark', 900)
Insert into tblStudents values ('Pam', 760)
Insert into tblStudents values ('John', 980)
Insert into tblStudents values ('Ram', 990)
Insert into tblStudents values ('Ron', 440)
Insert into tblStudents values ('Able', 320)
Insert into tblStudents values ('Steve', 983)
Insert into tblStudents values ('James', 720)
Insert into tblStudents values ('Mary', 870)
Insert into tblStudents values ('Nick', 680)
Step 2: Create an ado.net entity data model using table tblStudents. Upon creating the entity model, change the name of the entity to Student. Save changes and build the solution, so that the Student entity class gets compiled.
Step 3: Add "Shared" folder (if it doesn't already exists) in "Views" folder. Right click on "Shared" folder and add a partial view, with name = _Student.cshtml.
@model IEnumerable<MVCDemo.Models.Student>
<table style="border:1px solid black; background-color:Silver">
<tr>
<th>
@Html.DisplayNameFor(model => model.Name)
</th>
<th>
@Html.DisplayNameFor(model => model.TotalMarks)
</th>
</tr>
@foreach (var item in Model)
{
<tr>
<td>
@Html.DisplayFor(modelItem => item.Name)
</td>
<td>
@Html.DisplayFor(modelItem => item.TotalMarks)
</td>
</tr>
}
</table>
Step 4: Right click on the "Controllers" folder and add a controller with the following settings
1. Controller Name = HomeController
2. Template = Empty MVC controller
Copy and paste the following code. Please make sure to include MVCDemo.Models namespace.
public class HomeController : Controller
{
// Create an instance of DatabaseContext class
DatabaseContext db = new DatabaseContext();
public ActionResult Index()
{
return View();
}
// Return all students
public PartialViewResult All()
{
List<Student> model = db.Students.ToList();
return PartialView("_Student", model);
}
// Return Top3 students
public PartialViewResult Top3()
{
List<Student> model = db.Students.OrderByDescending(x => x.TotalMarks).Take(3).ToList();
return PartialView("_Student", model);
}
// Return Bottom3 students
public PartialViewResult Bottom3()
{
List<Student> model = db.Students.OrderBy(x => x.TotalMarks).Take(3).ToList();
return PartialView("_Student", model);
}
}
Step 5: Right click on the "Views" folder and add a folder with name = "Home". Right click on the "Home" folder and add a view with Name = "Index".
Points to remember:
a) For AJAX to work, jquery and jquery.unobtrusive-ajax javascript files need to be referenced. Reference to jquery.unobtrusive-ajax.js file should be after jquery.js reference.
b) Ajax.ActionLink() html helper has several overloaded versions. We have used the following version
ActionLink(string linkText, string actionName, AjaxOptions ajaxOptions);
First parameter : is the link text that we see on the user interface
Second parameter : is the name of the action method that needs to be invoked, when the link is cicked.
Third parameter : is the ajaxOptions object.
<div style="font-family:Arial">
<script src="~/Scripts/jquery-1.7.1.min.js" type="text/javascript"></script>
<script src="~/Scripts/jquery.unobtrusive-ajax.min.js" type="text/javascript"></script>
<h2>Students</h2>
@Ajax.ActionLink("All", "All",
new AjaxOptions
{
HttpMethod = "GET", // HttpMethod to use, GET or POST
UpdateTargetId = "divStudents", // ID of the HTML element to update
InsertionMode = InsertionMode.Replace // Replace the existing contents
})
<span style="color:Blue">|</span>
@Ajax.ActionLink("Top 3", "Top3",
new AjaxOptions
{
HttpMethod = "GET", // HttpMethod to use, GET or POST
UpdateTargetId = "divStudents", // ID of the HTML element to update
InsertionMode = InsertionMode.Replace // Replace the existing contents
})
<span style="color:Blue">|</span>
@Ajax.ActionLink("Bottom 3", "Bottom3",
new AjaxOptions
{
HttpMethod = "GET", // HttpMethod to use, GET or POST
UpdateTargetId = "divStudents", // ID of the HTML element to update
InsertionMode = InsertionMode.Replace // Replace the existing contents
})
<br /><br />
<div id="divStudents">
</div>
</div>
Part 89 - Remote validation in asp.net mvc
Part 90 - Remote validation in mvc when javascript is disabled
Part 91 - Create a custom remote attribute and override IsValid() method
ASP.NET AJAX enables a Web application to retrieve data from the server asynchronously and to update portions of the existing page. So these, partial page updates make web application more responsive and hence improves user experience.
In this video, let's discus using Ajax.ActionLink helper. By the end of this video, we should be able to load either
1. All students or
2. Top 3 students or
3. Bottom 3 students
depending on the link we have clicked.

Here are the steps to achieve this
Step 1: Sql script to create and populate table tblStudents
Create table tblStudents
(
Id int identity primary key,
Name nvarchar(50),
TotalMarks int
)
Insert into tblStudents values ('Mark', 900)
Insert into tblStudents values ('Pam', 760)
Insert into tblStudents values ('John', 980)
Insert into tblStudents values ('Ram', 990)
Insert into tblStudents values ('Ron', 440)
Insert into tblStudents values ('Able', 320)
Insert into tblStudents values ('Steve', 983)
Insert into tblStudents values ('James', 720)
Insert into tblStudents values ('Mary', 870)
Insert into tblStudents values ('Nick', 680)
Step 2: Create an ado.net entity data model using table tblStudents. Upon creating the entity model, change the name of the entity to Student. Save changes and build the solution, so that the Student entity class gets compiled.
Step 3: Add "Shared" folder (if it doesn't already exists) in "Views" folder. Right click on "Shared" folder and add a partial view, with name = _Student.cshtml.
@model IEnumerable<MVCDemo.Models.Student>
<table style="border:1px solid black; background-color:Silver">
<tr>
<th>
@Html.DisplayNameFor(model => model.Name)
</th>
<th>
@Html.DisplayNameFor(model => model.TotalMarks)
</th>
</tr>
@foreach (var item in Model)
{
<tr>
<td>
@Html.DisplayFor(modelItem => item.Name)
</td>
<td>
@Html.DisplayFor(modelItem => item.TotalMarks)
</td>
</tr>
}
</table>
Step 4: Right click on the "Controllers" folder and add a controller with the following settings
1. Controller Name = HomeController
2. Template = Empty MVC controller
Copy and paste the following code. Please make sure to include MVCDemo.Models namespace.
public class HomeController : Controller
{
// Create an instance of DatabaseContext class
DatabaseContext db = new DatabaseContext();
public ActionResult Index()
{
return View();
}
// Return all students
public PartialViewResult All()
{
List<Student> model = db.Students.ToList();
return PartialView("_Student", model);
}
// Return Top3 students
public PartialViewResult Top3()
{
List<Student> model = db.Students.OrderByDescending(x => x.TotalMarks).Take(3).ToList();
return PartialView("_Student", model);
}
// Return Bottom3 students
public PartialViewResult Bottom3()
{
List<Student> model = db.Students.OrderBy(x => x.TotalMarks).Take(3).ToList();
return PartialView("_Student", model);
}
}
Step 5: Right click on the "Views" folder and add a folder with name = "Home". Right click on the "Home" folder and add a view with Name = "Index".
Points to remember:
a) For AJAX to work, jquery and jquery.unobtrusive-ajax javascript files need to be referenced. Reference to jquery.unobtrusive-ajax.js file should be after jquery.js reference.
b) Ajax.ActionLink() html helper has several overloaded versions. We have used the following version
ActionLink(string linkText, string actionName, AjaxOptions ajaxOptions);
First parameter : is the link text that we see on the user interface
Second parameter : is the name of the action method that needs to be invoked, when the link is cicked.
Third parameter : is the ajaxOptions object.
<div style="font-family:Arial">
<script src="~/Scripts/jquery-1.7.1.min.js" type="text/javascript"></script>
<script src="~/Scripts/jquery.unobtrusive-ajax.min.js" type="text/javascript"></script>
<h2>Students</h2>
@Ajax.ActionLink("All", "All",
new AjaxOptions
{
HttpMethod = "GET", // HttpMethod to use, GET or POST
UpdateTargetId = "divStudents", // ID of the HTML element to update
InsertionMode = InsertionMode.Replace // Replace the existing contents
})
<span style="color:Blue">|</span>
@Ajax.ActionLink("Top 3", "Top3",
new AjaxOptions
{
HttpMethod = "GET", // HttpMethod to use, GET or POST
UpdateTargetId = "divStudents", // ID of the HTML element to update
InsertionMode = InsertionMode.Replace // Replace the existing contents
})
<span style="color:Blue">|</span>
@Ajax.ActionLink("Bottom 3", "Bottom3",
new AjaxOptions
{
HttpMethod = "GET", // HttpMethod to use, GET or POST
UpdateTargetId = "divStudents", // ID of the HTML element to update
InsertionMode = InsertionMode.Replace // Replace the existing contents
})
<br /><br />
<div id="divStudents">
</div>
</div>
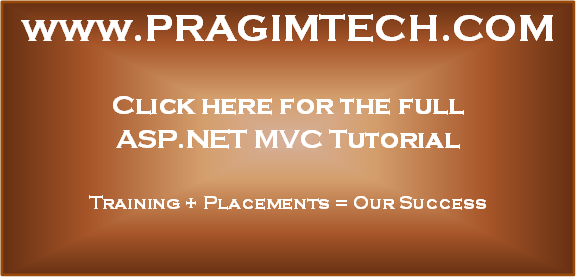
Please help me to understand the difference between view and view model..
ReplyDeleteView is just HTML Representation to the end user. But only one model item can be passed to a view.
DeleteBut in some scenarios you may like to use different models for a single view.
In that situation we create a different model in which we include all the required properties form different models. This particular model is called a ViewModel and then we create a view for this newly created Model.
Note: View for viewmodel is like normal view only one model object could be passed to it.
Akanksha,
ReplyDeleteView will have the user interface controls like button, text box ..where as ViewModel will have the data needed to show on the view. Or ViewModel will have data that will be bound to the View controls.
Hi Venkat
ReplyDeleteI have problem of loosing the model data when Partial view come back to controller from callback event. so every time I have to get the model from database.
what did I wrong here?
If you can help he, it would be really helpful.
structure:
(1). I have MVC project using UNIT/y for get the MODEL as service.
ex:
In App_Start --> UnityConfig.cs :
---------------------------------
public static void RegisterComponents()
{
UnityContainer container = new UnityContainer();
container.RegisterType();
container.RegisterType();
container.RegisterType();
DependencyResolver.SetResolver(new UnityDependencyResolver(container));
}
Service (in seperate dll):
-------------=-----------
public class SIBService : ISIBService
{
#region Construction.
//public SIBService(IWebRepository webRepository, IClinicalRepository ABCRepository)
public SIBService(IWebRepository webRepository, IClinicalRepository ABCRepository)
{
this.WebRepository = webRepository;
this.ABCRepository = ABCRepository;
}
#endregion
#region Properies.
protected IWebRepository WebRepository { get; set; }
protected IClinicalRepository ABCRepository { get; set; }
#endregion
public SituationsberichtModel GetSituationsberichtModel(string svnr)
{
if (this.WebRepository == null)
return null;
SituationsberichtModel model = this.WebRepository.GetPerson(svnr);
if (model == null)
return null;
model.ClinicSummaries = GetClinicalSummary(svnr);
return model;
}
..........
controller :
-----------
public SIBController(ISIBService service)
{
this.SIBService = service;
}
SituationsberichtModel modelContext = new SituationsberichtModel();
public ActionResult Index(string svnr)
{
if (string.IsNullOrEmpty(svnr))
{
string CallCmd = !String.IsNullOrEmpty(Request.Params["HTTP_REFERER"]) ? Request.Params["HTTP_REFERER"] : "";
this.Svnr = GetSvnr(CallCmd);
modelContext = this.SIBService.GetSituationsberichtModel(this.Svnr);
return View(modelContext);
}
}
public PartialViewResult SituationsberichtPartial()
{
return PartialView("SituationsberichtPartial", modelContext.ClinicSummaries);
}
Index.cshtml
-------------
@{Html.RenderAction("SituationsberichtPartial", new { model = Model });}
then the partial view :
----------------------
show the data using DevExpress
Html.DevExpress().GridView(
settings =>
{
settings.Name = "grid";
settings.CallbackRouteValues = new { Controller = "Situationsbericht", Action = "SituationsberichtPartial", clinicalSummary = Model };
hello sir, first of all your work is marvelous,
ReplyDeletei got All, top 3, bottom 3, output at new page. instead i completely followed you and made partial view name of _student.
please help me.
just add this line of code at the bottom of index view
Deleteyour problem will we solve for sure
@section scripts{
@Scripts.Render("~/Scripts/jquery.unobtrusive-ajax.js")
}
I even tried this and its opening it in whole new Page
Deletecan you tell me another solution.
thanks in advance.
Make sure you have the following installed:
Deletehttps://www.nuget.org/packages/Microsoft.jQuery.Unobtrusive.Ajax/
it also happend to me just now. the solution is that your take note of the code you used to install the jquery unobtrusive ajax .
DeleteInstall-Package jQuery.Ajax.Unobtrusive this is wrong.
Install-Package Microsoft.jQuery.Unobtrusive.Ajax and this is correct. the difference is that the correct one has Microsoft in it while the wrong one does not.
Microsoft.jQuery.Unobtrusive.Ajax SOLVED MY PROBLEM
Deletehello sir, first of all your work is marvelous,
ReplyDeletei got All, top 3, bottom 3, output at new page. instead i completely followed you and made partial view name of _student.
please help me.
it happened to me too..in scripts add ..jquery.obstrusive-ajax.min.js....i thinkn you are not including this one..once i include this i got the output as expected
Deleteuse
ReplyDelete@Ajax.ActionLink()
not @Html.ActionLink()
In above code table name is changed through entity design but in my solution it does not reflect...still taking tblStudents. why?
ReplyDeleteWhen ever the form appears and i click on the save button, it sends me to the create view which i dont want.. I hope to hear from you thank you
ReplyDeletehttps://blog.jquery.com/2011/11/21/jquery-1-7-1-released/
Deleteyou have to download the jquery 1.7.1 min js
-Hiro
showing an error model does not exist in the current context when i added the it in the controller return partialview("_student", model)
ReplyDeleteplease check the action name and may be debug model..
DeleteHello Everyone!
ReplyDeleteI want to start mvc with good knowledge of c# ado.net Linq and sql server....
Is asp.net webforms essential for me before learning mvc????
Plzz help me...
No,There is no need of webforms in MVC
DeleteWhat happens when you click "Top 3" link button and it shows you the top 3 student names and then you click back button on your browser? Please answer as soon as its possible for you. Thanks in advance.
ReplyDelete