Suggested Videos:
Part 1 - Can you store different types in an array in c#
What is jagged array?
A jagged array is an array of arrays.
Let us understand jagged array with an example
1. There are 3 employees - Mark, Matt, John
2. Mark has a bachelor, master and doctorate degree (3 qualifications)
3. Matt has only a bachelor degree (1 qualification)
4. John has a bachelor and a master degree (2 qualifications)
Different employees have different number of qualifications. To store their qualifications in a data structure, Jagged array is one of the choices.
public class Program
{
public static void Main()
{
// Create a string array to store the names of the employees
string[] employeeNames = new string[3];
employeeNames[0] = "Mark";
employeeNames[1] = "Matt";
employeeNames[2] = "John";
// Create a jagged array. As we want to store varying number of qualifications
// and as qualifications are strings, let's create an array of string arrays.
string[][] jaggedArray = new string[3][];
// Set the size of the first string array to 3, as we want to store 3 qualifications for Mark
jaggedArray[0] = new string[3];
// Set the size of the second string array to 1, as we want to store
// only 1 qualification for Matt
jaggedArray[1] = new string[1];
// Set the size of the third string array to 2, as we want to store 2 qualifications for John
jaggedArray[2] = new string[2];
// Set the values for the first string array
jaggedArray[0][0] = "Bachelor";
jaggedArray[0][1] = "Master";
jaggedArray[0][2] = "Doctorate";
// Set the values for the second string array
jaggedArray[1][0] = "Bachelor";
// Set the values for the thrid string array
jaggedArray[2][0] = "Bachelor";
jaggedArray[2][1] = "Master";
// Loop thru and print out the elements of Jagged array
for (int i = 0; i < jaggedArray.Length; i++)
{
Console.WriteLine(employeeNames[i]);
Console.WriteLine("--------");
string[] innerArray = jaggedArray[i];
for (int j = 0; j < innerArray.Length; j++)
{
Console.WriteLine(innerArray[j]);
}
Console.WriteLine();
}
}
}
In this example, we have created an array of string arrays. In a similar way, we create an array of integer arrays, an array of decimal arrays etc.
Part 1 - Can you store different types in an array in c#
What is jagged array?
A jagged array is an array of arrays.
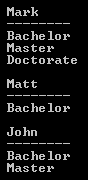
Let us understand jagged array with an example
1. There are 3 employees - Mark, Matt, John
2. Mark has a bachelor, master and doctorate degree (3 qualifications)
3. Matt has only a bachelor degree (1 qualification)
4. John has a bachelor and a master degree (2 qualifications)
Different employees have different number of qualifications. To store their qualifications in a data structure, Jagged array is one of the choices.
public class Program
{
public static void Main()
{
// Create a string array to store the names of the employees
string[] employeeNames = new string[3];
employeeNames[0] = "Mark";
employeeNames[1] = "Matt";
employeeNames[2] = "John";
// Create a jagged array. As we want to store varying number of qualifications
// and as qualifications are strings, let's create an array of string arrays.
string[][] jaggedArray = new string[3][];
// Set the size of the first string array to 3, as we want to store 3 qualifications for Mark
jaggedArray[0] = new string[3];
// Set the size of the second string array to 1, as we want to store
// only 1 qualification for Matt
jaggedArray[1] = new string[1];
// Set the size of the third string array to 2, as we want to store 2 qualifications for John
jaggedArray[2] = new string[2];
// Set the values for the first string array
jaggedArray[0][0] = "Bachelor";
jaggedArray[0][1] = "Master";
jaggedArray[0][2] = "Doctorate";
// Set the values for the second string array
jaggedArray[1][0] = "Bachelor";
// Set the values for the thrid string array
jaggedArray[2][0] = "Bachelor";
jaggedArray[2][1] = "Master";
// Loop thru and print out the elements of Jagged array
for (int i = 0; i < jaggedArray.Length; i++)
{
Console.WriteLine(employeeNames[i]);
Console.WriteLine("--------");
string[] innerArray = jaggedArray[i];
for (int j = 0; j < innerArray.Length; j++)
{
Console.WriteLine(innerArray[j]);
}
Console.WriteLine();
}
}
}
In this example, we have created an array of string arrays. In a similar way, we create an array of integer arrays, an array of decimal arrays etc.
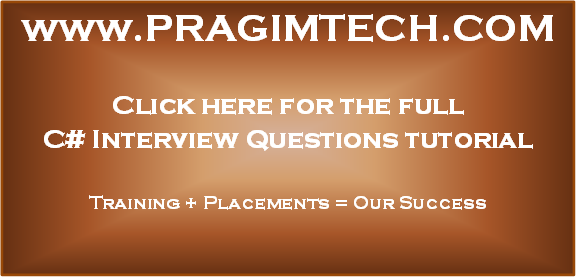
Hi Venkat Sir,
ReplyDeleteI came across a confusion, I am using a textbox, I made the viewstate of textbox control to off as well as on page level, I insert the value in textbox and the made the postback on the page using a button, ideally on postback the textbox value should reset the value to blank but its retaining its value.. How is it happening.. ?
Hi Manish, very good question. Unfortunately that's how an asp.net textbox works as far as viewstate is concerned. Let me record a video to clarify this.
Delete