Suggested Videos
Part 45 - Customize display and edit templates
Part 46 - Accessing model metada from custom templated helpers
Part 47 - Displaying images in MVC
In this video, we will discuss, creating custom html helpers. We will be using the example, that we worked with in Part 47. Please watch Part 47, before proceeding.
In Part 47, to render the image, we used the following code. We are building the image tag, by passing values for "src" and "alt" attributes.
<img src="@Url.Content(@Model.Photo)" alt="@Model.AlternateText" />
Though the above code is not very complex, it still makes sense to move this logic into it's own helper method. We don't want any complicated logic in our views. Views should be as simple as possible. Don't you think, it would be very nice, if we can render the image, using Image() html helper method as shown below. Unfortunately, MVC does not have built-in Image() html helper. So, let's build our own custom image html helper method.
@Html.Image(Model.Photo, Model.AlternateText)
Let's take a step back and understand html heper methods. The HTML helper method simply returns a string. To generate a textbox, we use the following code in our view.
@Html.TextBox("TextBox Name")
So, here TextBox() is an extension method defined in HtmlHelper class. In the above code, Html is the property of the View, which returns an instance of the HtmlHelper class.
Let's now add, Image() extension method, to HtmlHelper class.
1. Right click on MVCDemo project and add "CustomHtmlHelpers" folder.
2. Right click on "CustomHtmlHelpers" folder and add "CustomHtmlHelpers.cs" class file.
3. Copy and paste the following code. The code is commented and self-explanatory. TagBuilder class is in System.Web.Mvc namespace.
namespace MVCDemo.CustomHtmlHelpers
{
public static class CustomHtmlHelpers
{
public static IHtmlString Image(this HtmlHelper helper, string src, string alt)
{
// Build <img> tag
TagBuilder tb = new TagBuilder("img");
// Add "src" attribute
tb.Attributes.Add("src", VirtualPathUtility.ToAbsolute(src));
// Add "alt" attribute
tb.Attributes.Add("alt", alt);
// return MvcHtmlString. This class implements IHtmlString
// interface. IHtmlStrings will not be html encoded.
return new MvcHtmlString(tb.ToString(TagRenderMode.SelfClosing));
}
}
}
To use the custom Image() html helper in Details.cshtml view, please include the folowing using statement in Details.cshtml
@using MVCDemo.CustomHtmlHelpers;
As we intend to use this Image() html helper, in all our views, let's include "MVCDemo.CustomHtmlHelpers" namespace in web.config. This eliminates the need to include the namespace, in every view.
<system.web.webPages.razor>
<pages pageBaseType="System.Web.Mvc.WebViewPage">
<namespaces>
<add namespace="System.Web.Mvc" />
<add namespace="System.Web.Mvc.Ajax" />
<add namespace="System.Web.Mvc.Html" />
<add namespace="System.Web.Routing" />
<add namespace="MVCDemo.CustomHtmlHelpers" />
</namespaces>
</pages>
<host ....../>
</system.web.webPages.razor>
If you intend to use the Image() custom html helper, only with a set of views, then, include a web.config file in the specific views folder, and then specify the namespace in it.
Part 45 - Customize display and edit templates
Part 46 - Accessing model metada from custom templated helpers
Part 47 - Displaying images in MVC
In this video, we will discuss, creating custom html helpers. We will be using the example, that we worked with in Part 47. Please watch Part 47, before proceeding.
In Part 47, to render the image, we used the following code. We are building the image tag, by passing values for "src" and "alt" attributes.
<img src="@Url.Content(@Model.Photo)" alt="@Model.AlternateText" />
Though the above code is not very complex, it still makes sense to move this logic into it's own helper method. We don't want any complicated logic in our views. Views should be as simple as possible. Don't you think, it would be very nice, if we can render the image, using Image() html helper method as shown below. Unfortunately, MVC does not have built-in Image() html helper. So, let's build our own custom image html helper method.
@Html.Image(Model.Photo, Model.AlternateText)
Let's take a step back and understand html heper methods. The HTML helper method simply returns a string. To generate a textbox, we use the following code in our view.
@Html.TextBox("TextBox Name")
So, here TextBox() is an extension method defined in HtmlHelper class. In the above code, Html is the property of the View, which returns an instance of the HtmlHelper class.
Let's now add, Image() extension method, to HtmlHelper class.
1. Right click on MVCDemo project and add "CustomHtmlHelpers" folder.
2. Right click on "CustomHtmlHelpers" folder and add "CustomHtmlHelpers.cs" class file.
3. Copy and paste the following code. The code is commented and self-explanatory. TagBuilder class is in System.Web.Mvc namespace.
namespace MVCDemo.CustomHtmlHelpers
{
public static class CustomHtmlHelpers
{
public static IHtmlString Image(this HtmlHelper helper, string src, string alt)
{
// Build <img> tag
TagBuilder tb = new TagBuilder("img");
// Add "src" attribute
tb.Attributes.Add("src", VirtualPathUtility.ToAbsolute(src));
// Add "alt" attribute
tb.Attributes.Add("alt", alt);
// return MvcHtmlString. This class implements IHtmlString
// interface. IHtmlStrings will not be html encoded.
return new MvcHtmlString(tb.ToString(TagRenderMode.SelfClosing));
}
}
}
To use the custom Image() html helper in Details.cshtml view, please include the folowing using statement in Details.cshtml
@using MVCDemo.CustomHtmlHelpers;
As we intend to use this Image() html helper, in all our views, let's include "MVCDemo.CustomHtmlHelpers" namespace in web.config. This eliminates the need to include the namespace, in every view.
<system.web.webPages.razor>
<pages pageBaseType="System.Web.Mvc.WebViewPage">
<namespaces>
<add namespace="System.Web.Mvc" />
<add namespace="System.Web.Mvc.Ajax" />
<add namespace="System.Web.Mvc.Html" />
<add namespace="System.Web.Routing" />
<add namespace="MVCDemo.CustomHtmlHelpers" />
</namespaces>
</pages>
<host ....../>
</system.web.webPages.razor>
If you intend to use the Image() custom html helper, only with a set of views, then, include a web.config file in the specific views folder, and then specify the namespace in it.
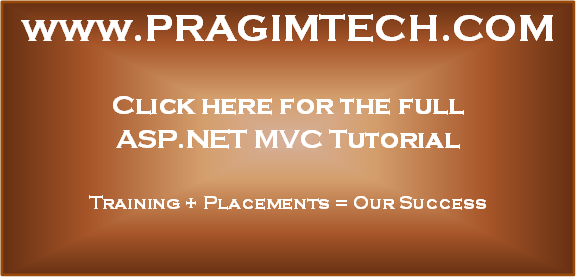
Can I Download power point slide used in these video tutorial if yes then provide us download link.
ReplyDeleteThese tutorials are very useful for me and my friends so thank you very much for the tutorials
Hi Manoj, the information that is present in the slides is available on my blog at the following link. Hope you will find it useful.
Deletehttp://csharp-video-tutorials.blogspot.com
I have organised all the ASP .NET, C#, and SQL Server video tutorials in to playlists, which could be useful to you.
http://www.youtube.com/user/kudvenkat/videos?view=1&flow=grid
Tips to effectively use my youtube channel.
http://www.youtube.com/watch?v=nT9uF09RMkw
If you want to receive email alerts, when new videos are uploaded, please feel free to subscribe to my youtube channel.
http://youtube.com/kudvenkat
If you like these videos, please click on the THUMBS UP button below the video.
May I ask you for a favour. I want these tutorials to be helpful for as many people as possible. Please free to share the link with your friends and family who you think would also benefit from them.
Good Luck
Venkat
Hi If the value of photo is null or empty then it throws an exception rather than showing alternate text
ReplyDeleteHere I would like to tell you my great admiration.
ReplyDeleteYour work is super wonderful.
Hi Venkat,
ReplyDeleteThis custom built Html helper Image tag is not working fro me.
I did everything as you said in the video.
Added the namespace in web.config & restarted the VS2010.
But i'm not getting the output. Instead I'm getting error.