Suggested Videos
Part 47 - Sorting a gridview that does not use any datasource control
Part 48 - Sorting an asp.net gridview in ascending and descending order
Part 49 - How to include sort arrows when sorting an asp.net gridview control
In this video we will discuss about implementing paging in a gridview control that uses sqldatasource control.
We will be using tblEmployee table for this demo. Please refer to Part 13 by clicking here, if you need the sql script to create and populate these tables.
Step 1: Drag and drop a gridview, a label and a sqldatasource control on webform1.aspx
Step 2: Configure SqlDataSource1 control to retrieve data from tblEmployee table.
Step 3: Associate GridView1, with SqlDataSource1 control and make sure to select "Enable Paging" checkbox.
Step 4: To control the number of rows displayed in the gridview, set PageSize property. Since, I want to display 3 rows on a page, I have set the PageSize to 3.
Step 5: Generate GridView1 control's PreRender eventhandler method. Copy and paste the following code in code-behind file.
protected void GridView1_PreRender(object sender, EventArgs e)
{
Label1.Text = "Displaying Page " + (GridView1.PageIndex + 1).ToString()
+ " of " + GridView1.PageCount.ToString();
}
That's it we are done. Run the application. Since we have 6 records in the database table, and as we have set the pagesize to 3, the GridView1 control shows 2 pages with 3 records on each page.
Here is the HTML
<asp:GridView ID="GridView1" runat="server" AllowPaging="True"
AutoGenerateColumns="False" DataKeyNames="EmployeeId"
DataSourceID="SqlDataSource1" PageSize="3"
onprerender="GridView1_PreRender">
<Columns>
<asp:BoundField DataField="EmployeeId" ReadOnly="True"
InsertVisible="False" HeaderText="EmployeeId"
SortExpression="EmployeeId" />
<asp:BoundField DataField="Name" HeaderText="Name"
SortExpression="Name" />
<asp:BoundField DataField="Gender" HeaderText="Gender"
SortExpression="Gender" />
<asp:BoundField DataField="City" HeaderText="City"
SortExpression="City" />
</Columns>
</asp:GridView>
<asp:Label ID="Label1" runat="server"></asp:Label>
<asp:SqlDataSource ID="SqlDataSource1" runat="server"
ConnectionString="<%$ ConnectionStrings:SampleConnectionString %>"
SelectCommand="SELECT * FROM [tblEmployee]">
</asp:SqlDataSource>
Points to remember:
1. To control the number of rows displayed per page use PageSize property of GridView control.
2. When paging is enabled AllowPaging attribute of gridview control is set to True
AllowPaging="True"
3. PageIndex property of gridview control can be used to retrieve the page that is currently being viewed. PageIndex is ZERO based, so add 1 to it, to compute the correct page number that is being displayed in the gridview.
4. To get the total number of pages available, use PageCount property of GridView control.
5. When sqldatasource control is used, we get default paging. This means that, though we are displaying only 3 records on a page all the rows will be retrieved from the database and the gridview control then displays the correct set of 3 records depending on the page you are viewing. Everytime you click on a page, all the rows will be retrieved. Obviously this is bad for performance. We will discuss about custom paging in a later video session, which solves this problem. With custom paging it is possible to retrieve only the required number of rows and not all of them.
Part 47 - Sorting a gridview that does not use any datasource control
Part 48 - Sorting an asp.net gridview in ascending and descending order
Part 49 - How to include sort arrows when sorting an asp.net gridview control
In this video we will discuss about implementing paging in a gridview control that uses sqldatasource control.
We will be using tblEmployee table for this demo. Please refer to Part 13 by clicking here, if you need the sql script to create and populate these tables.
Step 1: Drag and drop a gridview, a label and a sqldatasource control on webform1.aspx
Step 2: Configure SqlDataSource1 control to retrieve data from tblEmployee table.
Step 3: Associate GridView1, with SqlDataSource1 control and make sure to select "Enable Paging" checkbox.
Step 4: To control the number of rows displayed in the gridview, set PageSize property. Since, I want to display 3 rows on a page, I have set the PageSize to 3.
Step 5: Generate GridView1 control's PreRender eventhandler method. Copy and paste the following code in code-behind file.
protected void GridView1_PreRender(object sender, EventArgs e)
{
Label1.Text = "Displaying Page " + (GridView1.PageIndex + 1).ToString()
+ " of " + GridView1.PageCount.ToString();
}
That's it we are done. Run the application. Since we have 6 records in the database table, and as we have set the pagesize to 3, the GridView1 control shows 2 pages with 3 records on each page.
Here is the HTML
<asp:GridView ID="GridView1" runat="server" AllowPaging="True"
AutoGenerateColumns="False" DataKeyNames="EmployeeId"
DataSourceID="SqlDataSource1" PageSize="3"
onprerender="GridView1_PreRender">
<Columns>
<asp:BoundField DataField="EmployeeId" ReadOnly="True"
InsertVisible="False" HeaderText="EmployeeId"
SortExpression="EmployeeId" />
<asp:BoundField DataField="Name" HeaderText="Name"
SortExpression="Name" />
<asp:BoundField DataField="Gender" HeaderText="Gender"
SortExpression="Gender" />
<asp:BoundField DataField="City" HeaderText="City"
SortExpression="City" />
</Columns>
</asp:GridView>
<asp:Label ID="Label1" runat="server"></asp:Label>
<asp:SqlDataSource ID="SqlDataSource1" runat="server"
ConnectionString="<%$ ConnectionStrings:SampleConnectionString %>"
SelectCommand="SELECT * FROM [tblEmployee]">
</asp:SqlDataSource>
Points to remember:
1. To control the number of rows displayed per page use PageSize property of GridView control.
2. When paging is enabled AllowPaging attribute of gridview control is set to True
AllowPaging="True"
3. PageIndex property of gridview control can be used to retrieve the page that is currently being viewed. PageIndex is ZERO based, so add 1 to it, to compute the correct page number that is being displayed in the gridview.
4. To get the total number of pages available, use PageCount property of GridView control.
5. When sqldatasource control is used, we get default paging. This means that, though we are displaying only 3 records on a page all the rows will be retrieved from the database and the gridview control then displays the correct set of 3 records depending on the page you are viewing. Everytime you click on a page, all the rows will be retrieved. Obviously this is bad for performance. We will discuss about custom paging in a later video session, which solves this problem. With custom paging it is possible to retrieve only the required number of rows and not all of them.
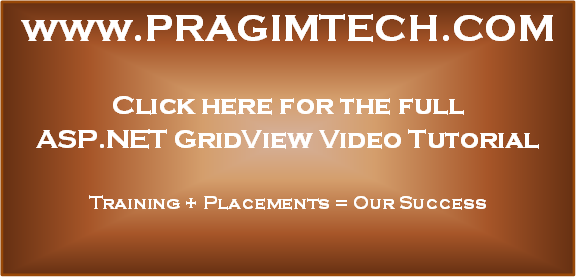
The Gridview.Pagecount returns 0 in the Page PreRender event for the get request which is wrong. Please help to solve this...
ReplyDelete