Suggested Videos
Part 45 - Sorting a gridview that uses objectdatasource control and a dataset
Part 46 - Sorting a gridview that uses objectdatasource control and business objects
Part 47 - Sorting a gridview that does not use any datasource control
Please watch Part 47, before proceeding with this video. The problem in Part 47 was, we were not able to sort the data in descending order. In this video we will discuss about fixing this issue.
Step 1: Drag and drop a gridview on webform1.aspx
Step 2: Add a class file with name = "EmployeeDataAccessLayer.cs". Copy and paste the following code.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
namespace Demo
{
public class Employee
{
public int EmployeeId { get; set; }
public string Name { get; set; }
public string Gender { get; set; }
public string City { get; set; }
}
public class EmployeeDataAccessLayer
{
public static List<Employee> GetAllEmployees(string sortColumn)
{
List<Employee> listEmployees = new List<Employee>();
string CS = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(CS))
{
string sqlQuery = "Select * from tblEmployee";
if (!string.IsNullOrEmpty(sortColumn))
{
sqlQuery += " order by " + sortColumn;
}
SqlCommand cmd = new SqlCommand(sqlQuery, con);
con.Open();
SqlDataReader rdr = cmd.ExecuteReader();
while (rdr.Read())
{
Employee employee = new Employee();
employee.EmployeeId = Convert.ToInt32(rdr["EmployeeId"]);
employee.Name = rdr["Name"].ToString();
employee.Gender = rdr["Gender"].ToString();
employee.City = rdr["City"].ToString();
listEmployees.Add(employee);
}
}
return listEmployees;
}
}
}
Step 3: Generate event handler method, for Sorting event of GridView1 control.
Step 4: Flip webform1.aspx to html source mode and set the following 2 custom attributes on GridView1 control.
CurrentSortField="EmployeeId"
CurrentSortDirection="ASC"
At this point the HTML of your webform, should be as shown below.
<asp:GridView ID="GridView1"
runat="server"
AllowSorting="True"
onsorting="GridView1_Sorting"
CurrentSortField="EmployeeId"
CurrentSortDirection="ASC">
</asp:GridView>
Step 5: Copy and paste the following code in WebForm1.aspx.cs
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
GridView1.DataSource = EmployeeDataAccessLayer.GetAllEmployees("EmployeeId");
GridView1.DataBind();
}
}
protected void GridView1_Sorting(object sender, GridViewSortEventArgs e)
{
//Response.Write("Sort Expression = " + e.SortExpression);
//Response.Write("<br/>");
//Response.Write("Sort Direction = " + e.SortDirection.ToString());
SortDirection sortDirection = SortDirection.Ascending;
string sortField = string.Empty;
SortGridview((GridView)sender, e, out sortDirection, out sortField);
string strSortDirection = sortDirection == SortDirection.Ascending ? "ASC" : "DESC";
GridView1.DataSource = EmployeeDataAccessLayer.GetAllEmployees(e.SortExpression + " " + strSortDirection);
GridView1.DataBind();
}
private void SortGridview(GridView gridView, GridViewSortEventArgs e, out SortDirection sortDirection, out string sortField)
{
sortField = e.SortExpression;
sortDirection = e.SortDirection;
if (gridView.Attributes["CurrentSortField"] != null && gridView.Attributes["CurrentSortDirection"] != null)
{
if (sortField == gridView.Attributes["CurrentSortField"])
{
if (gridView.Attributes["CurrentSortDirection"] == "ASC")
{
sortDirection = SortDirection.Descending;
}
else
{
sortDirection = SortDirection.Ascending;
}
}
gridView.Attributes["CurrentSortField"] = sortField;
gridView.Attributes["CurrentSortDirection"] = (sortDirection == SortDirection.Ascending ? "ASC" : "DESC");
}
}
Part 45 - Sorting a gridview that uses objectdatasource control and a dataset
Part 46 - Sorting a gridview that uses objectdatasource control and business objects
Part 47 - Sorting a gridview that does not use any datasource control
Please watch Part 47, before proceeding with this video. The problem in Part 47 was, we were not able to sort the data in descending order. In this video we will discuss about fixing this issue.
Step 1: Drag and drop a gridview on webform1.aspx
Step 2: Add a class file with name = "EmployeeDataAccessLayer.cs". Copy and paste the following code.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
namespace Demo
{
public class Employee
{
public int EmployeeId { get; set; }
public string Name { get; set; }
public string Gender { get; set; }
public string City { get; set; }
}
public class EmployeeDataAccessLayer
{
public static List<Employee> GetAllEmployees(string sortColumn)
{
List<Employee> listEmployees = new List<Employee>();
string CS = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(CS))
{
string sqlQuery = "Select * from tblEmployee";
if (!string.IsNullOrEmpty(sortColumn))
{
sqlQuery += " order by " + sortColumn;
}
SqlCommand cmd = new SqlCommand(sqlQuery, con);
con.Open();
SqlDataReader rdr = cmd.ExecuteReader();
while (rdr.Read())
{
Employee employee = new Employee();
employee.EmployeeId = Convert.ToInt32(rdr["EmployeeId"]);
employee.Name = rdr["Name"].ToString();
employee.Gender = rdr["Gender"].ToString();
employee.City = rdr["City"].ToString();
listEmployees.Add(employee);
}
}
return listEmployees;
}
}
}
Step 3: Generate event handler method, for Sorting event of GridView1 control.
Step 4: Flip webform1.aspx to html source mode and set the following 2 custom attributes on GridView1 control.
CurrentSortField="EmployeeId"
CurrentSortDirection="ASC"
At this point the HTML of your webform, should be as shown below.
<asp:GridView ID="GridView1"
runat="server"
AllowSorting="True"
onsorting="GridView1_Sorting"
CurrentSortField="EmployeeId"
CurrentSortDirection="ASC">
</asp:GridView>
Step 5: Copy and paste the following code in WebForm1.aspx.cs
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
GridView1.DataSource = EmployeeDataAccessLayer.GetAllEmployees("EmployeeId");
GridView1.DataBind();
}
}
protected void GridView1_Sorting(object sender, GridViewSortEventArgs e)
{
//Response.Write("Sort Expression = " + e.SortExpression);
//Response.Write("<br/>");
//Response.Write("Sort Direction = " + e.SortDirection.ToString());
SortDirection sortDirection = SortDirection.Ascending;
string sortField = string.Empty;
SortGridview((GridView)sender, e, out sortDirection, out sortField);
string strSortDirection = sortDirection == SortDirection.Ascending ? "ASC" : "DESC";
GridView1.DataSource = EmployeeDataAccessLayer.GetAllEmployees(e.SortExpression + " " + strSortDirection);
GridView1.DataBind();
}
private void SortGridview(GridView gridView, GridViewSortEventArgs e, out SortDirection sortDirection, out string sortField)
{
sortField = e.SortExpression;
sortDirection = e.SortDirection;
if (gridView.Attributes["CurrentSortField"] != null && gridView.Attributes["CurrentSortDirection"] != null)
{
if (sortField == gridView.Attributes["CurrentSortField"])
{
if (gridView.Attributes["CurrentSortDirection"] == "ASC")
{
sortDirection = SortDirection.Descending;
}
else
{
sortDirection = SortDirection.Ascending;
}
}
gridView.Attributes["CurrentSortField"] = sortField;
gridView.Attributes["CurrentSortDirection"] = (sortDirection == SortDirection.Ascending ? "ASC" : "DESC");
}
}
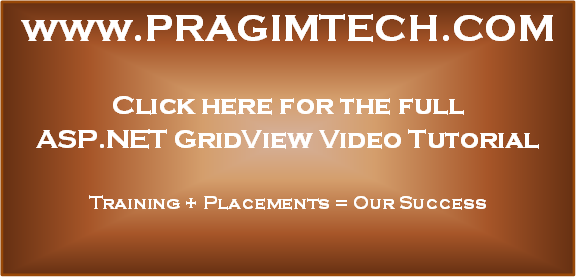
Dear Mr. Vanket
ReplyDeleteI write this below code without any custom attributes on GridView, and its working
correctly
''''xsort Variable is defined in the Module
If xsort = 0 Then
''''chksort Variable is defined in this class
chksort = "ASC"
GridView1.DataSource = Demo.EmployeeDataAccessLayer6.GetAllEmployees(e.SortExpression + " " + chksort)
xsort = 1
Else
chksort = "DESC"
GridView1.DataSource = Demo.EmployeeDataAccessLayer6.GetAllEmployees(e.SortExpression + " " + chksort)
xsort = 0
End If
GridView1.DataBind()
if (gridView.Attributes["CurrentSortField"] != null && gridView.Attributes["CurrentSortDirection"] != null)
ReplyDelete{
if (sortField == gridView.Attributes["CurrentSortField"])
{
if (gridView.Attributes["CurrentSortDirection"] == "ASC")
{
sortDirection = SortDirection.Descending;
}
else
{
sortDirection = SortDirection.Ascending;
}
}
In this above code you have written only checks if sortField is equals to CurrentSortField attribute, this is not true if we click on Name column of gridview, So how this code is working!!
Could you please elaborate on this