Suggested Videos
Part 44 - Sorting a gridview that uses sqldatasource control
Part 45 - Sorting a gridview that uses objectdatasource control and a dataset
Part 46 - Sorting a gridview that uses objectdatasource control and business objects
In this video we will discuss about sorting a gridview control that does not use any datasource control.
We will be using tblEmployee table for this demo. Please refer to Part 13 by clicking here, if you need the sql script to create and populate these tables.
Please watch Part 46, before proceeding with this video.
Step 1: Drag and drop a gridview on webform1.aspx
Step 2: Add a class file with name = "EmployeeDataAccessLayer.cs". Copy and paste the following code.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
namespace Demo
{
public class Employee
{
public int EmployeeId { get; set; }
public string Name { get; set; }
public string Gender { get; set; }
public string City { get; set; }
}
public class EmployeeDataAccessLayer
{
public static List<Employee> GetAllEmployees(string sortColumn)
{
List<Employee> listEmployees = new List<Employee>();
string CS = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(CS))
{
string sqlQuery = "Select * from tblEmployee";
if (!string.IsNullOrEmpty(sortColumn))
{
sqlQuery += " order by " + sortColumn;
}
SqlCommand cmd = new SqlCommand(sqlQuery, con);
con.Open();
SqlDataReader rdr = cmd.ExecuteReader();
while (rdr.Read())
{
Employee employee = new Employee();
employee.EmployeeId = Convert.ToInt32(rdr["EmployeeId"]);
employee.Name = rdr["Name"].ToString();
employee.Gender = rdr["Gender"].ToString();
employee.City = rdr["City"].ToString();
listEmployees.Add(employee);
}
}
return listEmployees;
}
}
}
Step 3: Generate event handler method, for Sorting event of GridView1 control.
Step 4: Copy and paste the following code in WebForm1.aspx.cs
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
GridView1.DataSource = EmployeeDataAccessLayer.GetAllEmployees("EmployeeId");
GridView1.DataBind();
}
}
protected void GridView1_Sorting(object sender, GridViewSortEventArgs e)
{
string strSortDirection =
e.SortDirection == SortDirection.Ascending ? "ASC" : "DESC";
GridView1.DataSource = EmployeeDataAccessLayer.GetAllEmployees
(e.SortExpression + " " + strSortDirection);
GridView1.DataBind();
}
Run the application. Click the column header to sort data. The data gets sorted in ascending order. Now, click again to sort data in descending order. It does not work.
In our next video, we will discuss about correcting this issue.
Part 44 - Sorting a gridview that uses sqldatasource control
Part 45 - Sorting a gridview that uses objectdatasource control and a dataset
Part 46 - Sorting a gridview that uses objectdatasource control and business objects
In this video we will discuss about sorting a gridview control that does not use any datasource control.
We will be using tblEmployee table for this demo. Please refer to Part 13 by clicking here, if you need the sql script to create and populate these tables.
Please watch Part 46, before proceeding with this video.
Step 1: Drag and drop a gridview on webform1.aspx
Step 2: Add a class file with name = "EmployeeDataAccessLayer.cs". Copy and paste the following code.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
namespace Demo
{
public class Employee
{
public int EmployeeId { get; set; }
public string Name { get; set; }
public string Gender { get; set; }
public string City { get; set; }
}
public class EmployeeDataAccessLayer
{
public static List<Employee> GetAllEmployees(string sortColumn)
{
List<Employee> listEmployees = new List<Employee>();
string CS = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(CS))
{
string sqlQuery = "Select * from tblEmployee";
if (!string.IsNullOrEmpty(sortColumn))
{
sqlQuery += " order by " + sortColumn;
}
SqlCommand cmd = new SqlCommand(sqlQuery, con);
con.Open();
SqlDataReader rdr = cmd.ExecuteReader();
while (rdr.Read())
{
Employee employee = new Employee();
employee.EmployeeId = Convert.ToInt32(rdr["EmployeeId"]);
employee.Name = rdr["Name"].ToString();
employee.Gender = rdr["Gender"].ToString();
employee.City = rdr["City"].ToString();
listEmployees.Add(employee);
}
}
return listEmployees;
}
}
}
Step 3: Generate event handler method, for Sorting event of GridView1 control.
Step 4: Copy and paste the following code in WebForm1.aspx.cs
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
GridView1.DataSource = EmployeeDataAccessLayer.GetAllEmployees("EmployeeId");
GridView1.DataBind();
}
}
protected void GridView1_Sorting(object sender, GridViewSortEventArgs e)
{
string strSortDirection =
e.SortDirection == SortDirection.Ascending ? "ASC" : "DESC";
GridView1.DataSource = EmployeeDataAccessLayer.GetAllEmployees
(e.SortExpression + " " + strSortDirection);
GridView1.DataBind();
}
Run the application. Click the column header to sort data. The data gets sorted in ascending order. Now, click again to sort data in descending order. It does not work.
In our next video, we will discuss about correcting this issue.
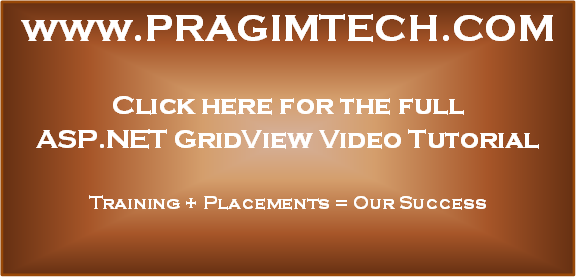
No comments:
Post a Comment
It would be great if you can help share these free resources