Suggested Videos
Part 54 - Custom paging in gridview without using datasource controls
Part 55 - Custom sorting and paging in gridview
Part 56 - Gridview paging using a dropdownlist
In this video, we will discuss about exporting gridview data to excel. We will be using tblEmployee table for this demo. Please refer to Part 13 by clicking here, if you need the sql script to create and populate these tables.
Step 1: Create an asp.net web application project.
Step 2: Drag and drop a gridview control and a button control on webform1.aspx. Autoformat the gridview control to use "BrownSugar" scheme. Set the following properties of the button control.
ID="btnExportToExcel"
Text="Export to excel"
OnClick="btnExportToExcel_Click"
At this point the HTML on webform1.aspx should be as shown below.
<asp:GridView ID="GridView1" runat="server" BackColor="#DEBA84"
BorderColor="#DEBA84" BorderStyle="None" BorderWidth="1px"
CellPadding="3" CellSpacing="2">
<FooterStyle BackColor="#F7DFB5" ForeColor="#8C4510" />
<HeaderStyle BackColor="#A55129" Font-Bold="True"
ForeColor="White" />
<PagerStyle ForeColor="#8C4510" HorizontalAlign="Center" />
<RowStyle BackColor="#FFF7E7" ForeColor="#8C4510" />
<SelectedRowStyle BackColor="#738A9C" Font-Bold="True"
ForeColor="White" />
<SortedAscendingCellStyle BackColor="#FFF1D4" />
<SortedAscendingHeaderStyle BackColor="#B95C30" />
<SortedDescendingCellStyle BackColor="#F1E5CE" />
<SortedDescendingHeaderStyle BackColor="#93451F" />
</asp:GridView>
<asp:Button ID="btnExportToExcel" runat="server"
Text="Export to excel"
OnClick="btnExportToExcel_Click" />
Step 3: Copy and paste the following code in webform1.aspx.cs. The code is well commented and is self-explanatory.
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
BindData();
}
}
private void BindData()
{
string CS = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(CS))
{
SqlDataAdapter da = new SqlDataAdapter("Select * from tblEmployee", con);
DataSet ds = new DataSet();
da.Fill(ds);
GridView1.DataSource = ds;
GridView1.DataBind();
}
}
protected void btnExportToExcel_Click(object sender, EventArgs e)
{
// Clear all content output from the buffer stream
Response.ClearContent();
// Specify the default file name using "content-disposition" RESPONSE header
Response.AppendHeader("content-disposition", "attachment; filename=Employees.xls");
// Set excel as the HTTP MIME type
Response.ContentType = "application/excel";
// Create an instance of stringWriter for writing information to a string
System.IO.StringWriter stringWriter = new System.IO.StringWriter();
// Create an instance of HtmlTextWriter class for writing markup
// characters and text to an ASP.NET server control output stream
HtmlTextWriter htw = new HtmlTextWriter(stringWriter);
// Set white color as the background color for gridview header row
GridView1.HeaderRow.Style.Add("background-color", "#FFFFFF");
// Set background color of each cell of GridView1 header row
foreach (TableCell tableCell in GridView1.HeaderRow.Cells)
{
tableCell.Style["background-color"] = "#A55129";
}
// Set background color of each cell of each data row of GridView1
foreach (GridViewRow gridViewRow in GridView1.Rows)
{
gridViewRow.BackColor = System.Drawing.Color.White;
foreach (TableCell gridViewRowTableCell in gridViewRow.Cells)
{
gridViewRowTableCell.Style["background-color"] = "#FFF7E7";
}
}
GridView1.RenderControl(htw);
Response.Write(stringWriter.ToString());
Response.End();
}
Step 4: Run the application and click on "Export to excel" button. You will get an error stating - Control 'GridView1' of type 'GridView' must be placed inside a form tag with runat=server. This is because, we are calling GridView1.RenderControl(htmlTextWriter) method. This causes .net to think that a Server-Control is being rendered outside of a Form. To fix this error, we need to override VerifyRenderingInServerForm() method. Copy and paste the following method in webform1.aspx.cs.
public override void VerifyRenderingInServerForm(Control control)
{
}
At this point, run the application and click on "Export to excel button. The data gets exported to excel as expected. However, when you try to open the file you will get a warning message stating - The file you are trying to open, 'Employee.xls', is in a different format than specified by the file extension. Verify that the file is not corrupted and is from a trusted source before opening the file. Do you want to open the file now?
According to MSDN - The warning message is a user-notification function that was added to Excel 2007. The warning message can help prevent unexpected problems that might occur because of possible incompatibility between the actual content of the file and the file name extension.
One way to resolve this error, is to edit the registry. Microsoft knowledge base article related to this error
http://support.microsoft.com/kb/948615
If you want to export the same data to Microsoft word, there are only 2 changes that you need to do.
First Change: Change the file extension to ".doc" instead of ".xls"
Response.AppendHeader("content-disposition", "attachment; filename=Employees.doc");
Second Change: Set the content type to word
Response.ContentType = "application/word";
Part 54 - Custom paging in gridview without using datasource controls
Part 55 - Custom sorting and paging in gridview
Part 56 - Gridview paging using a dropdownlist
In this video, we will discuss about exporting gridview data to excel. We will be using tblEmployee table for this demo. Please refer to Part 13 by clicking here, if you need the sql script to create and populate these tables.
Step 1: Create an asp.net web application project.
Step 2: Drag and drop a gridview control and a button control on webform1.aspx. Autoformat the gridview control to use "BrownSugar" scheme. Set the following properties of the button control.
ID="btnExportToExcel"
Text="Export to excel"
OnClick="btnExportToExcel_Click"
At this point the HTML on webform1.aspx should be as shown below.
<asp:GridView ID="GridView1" runat="server" BackColor="#DEBA84"
BorderColor="#DEBA84" BorderStyle="None" BorderWidth="1px"
CellPadding="3" CellSpacing="2">
<FooterStyle BackColor="#F7DFB5" ForeColor="#8C4510" />
<HeaderStyle BackColor="#A55129" Font-Bold="True"
ForeColor="White" />
<PagerStyle ForeColor="#8C4510" HorizontalAlign="Center" />
<RowStyle BackColor="#FFF7E7" ForeColor="#8C4510" />
<SelectedRowStyle BackColor="#738A9C" Font-Bold="True"
ForeColor="White" />
<SortedAscendingCellStyle BackColor="#FFF1D4" />
<SortedAscendingHeaderStyle BackColor="#B95C30" />
<SortedDescendingCellStyle BackColor="#F1E5CE" />
<SortedDescendingHeaderStyle BackColor="#93451F" />
</asp:GridView>
<asp:Button ID="btnExportToExcel" runat="server"
Text="Export to excel"
OnClick="btnExportToExcel_Click" />
Step 3: Copy and paste the following code in webform1.aspx.cs. The code is well commented and is self-explanatory.
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
BindData();
}
}
private void BindData()
{
string CS = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(CS))
{
SqlDataAdapter da = new SqlDataAdapter("Select * from tblEmployee", con);
DataSet ds = new DataSet();
da.Fill(ds);
GridView1.DataSource = ds;
GridView1.DataBind();
}
}
protected void btnExportToExcel_Click(object sender, EventArgs e)
{
// Clear all content output from the buffer stream
Response.ClearContent();
// Specify the default file name using "content-disposition" RESPONSE header
Response.AppendHeader("content-disposition", "attachment; filename=Employees.xls");
// Set excel as the HTTP MIME type
Response.ContentType = "application/excel";
// Create an instance of stringWriter for writing information to a string
System.IO.StringWriter stringWriter = new System.IO.StringWriter();
// Create an instance of HtmlTextWriter class for writing markup
// characters and text to an ASP.NET server control output stream
HtmlTextWriter htw = new HtmlTextWriter(stringWriter);
// Set white color as the background color for gridview header row
GridView1.HeaderRow.Style.Add("background-color", "#FFFFFF");
// Set background color of each cell of GridView1 header row
foreach (TableCell tableCell in GridView1.HeaderRow.Cells)
{
tableCell.Style["background-color"] = "#A55129";
}
// Set background color of each cell of each data row of GridView1
foreach (GridViewRow gridViewRow in GridView1.Rows)
{
gridViewRow.BackColor = System.Drawing.Color.White;
foreach (TableCell gridViewRowTableCell in gridViewRow.Cells)
{
gridViewRowTableCell.Style["background-color"] = "#FFF7E7";
}
}
GridView1.RenderControl(htw);
Response.Write(stringWriter.ToString());
Response.End();
}
Step 4: Run the application and click on "Export to excel" button. You will get an error stating - Control 'GridView1' of type 'GridView' must be placed inside a form tag with runat=server. This is because, we are calling GridView1.RenderControl(htmlTextWriter) method. This causes .net to think that a Server-Control is being rendered outside of a Form. To fix this error, we need to override VerifyRenderingInServerForm() method. Copy and paste the following method in webform1.aspx.cs.
public override void VerifyRenderingInServerForm(Control control)
{
}
At this point, run the application and click on "Export to excel button. The data gets exported to excel as expected. However, when you try to open the file you will get a warning message stating - The file you are trying to open, 'Employee.xls', is in a different format than specified by the file extension. Verify that the file is not corrupted and is from a trusted source before opening the file. Do you want to open the file now?
According to MSDN - The warning message is a user-notification function that was added to Excel 2007. The warning message can help prevent unexpected problems that might occur because of possible incompatibility between the actual content of the file and the file name extension.
One way to resolve this error, is to edit the registry. Microsoft knowledge base article related to this error
http://support.microsoft.com/kb/948615
If you want to export the same data to Microsoft word, there are only 2 changes that you need to do.
First Change: Change the file extension to ".doc" instead of ".xls"
Response.AppendHeader("content-disposition", "attachment; filename=Employees.doc");
Second Change: Set the content type to word
Response.ContentType = "application/word";
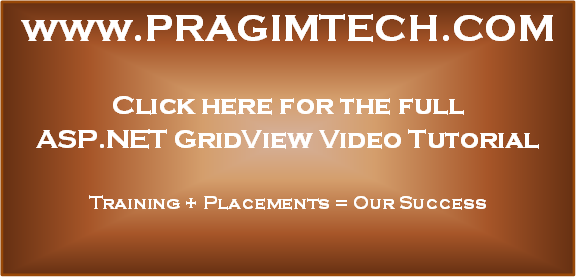
Excelent Sir!
ReplyDeleteBut if I set pagination, then it shows error.
And how to save Excel in the Server?
hi
ReplyDeletethanks for your videos it really helps, i was wondering if i can import from excel sheet to ASP.net, if it possible could you please tell me how, it will really be helpful in my graduation project.
thanks
am looking for a word to hear from you soon.
Tagwa Osman
tagwa-2009@hotmail.com
Thanks for your videos.Your explanation is very nice.
ReplyDeleteHow to freeze gridview header in exported excel?
how to hide pop up message : "do you want to open or save this file.?"
ReplyDeletebecause i want to save to server automatically without pop up message.
How could I implement this same operation in console application?
ReplyDeletehi. excellent tutorial. could you tell me how can i export data in multiple worksheets for the same excel file? Thanks
ReplyDeleteThe better way to do this might be to use the open xml library to get rid of that warning
ReplyDeletesir i have a query that i m getting try to export the data gridview to execl but my data is in form of dataset so on that sitution is not working
ReplyDeleteHello Sir, Thanks for supporting and teaching to beginners like us. one more request from my side is that please prepare a video on "Bulk Import Data from an Excel sheet to database table". please sir please please please please...
ReplyDeleteOnly the first page is downloaded, how can i download all the datatable data?
ReplyDelete