Suggested Videos
Part 8 - Angular formbuilder example | Text | Slides
Part 9 - Angular reactive forms validation | Text | Slides
Part 10 - Angular form control valuechanges | Text | Slides
In this video we will discuss, how to loop through all form controls in a formgroup including nested form groups in a reactive form.
Understanding this technique is very useful, as it can help us perform the following on all the form controls in a reactive form
Here is what we want to do : Loop through each form control in the following employeeForm form group including nested skills form group and log the form control key and value to the console.
For example, if we have the following sample data on the form
We want every form control key and value to be logged to the console as shown below.
Here is the method. It is commented and self-explanatory
In our next video we will discuss, moving validation messages into the component class from the view template.
Part 8 - Angular formbuilder example | Text | Slides
Part 9 - Angular reactive forms validation | Text | Slides
Part 10 - Angular form control valuechanges | Text | Slides
In this video we will discuss, how to loop through all form controls in a formgroup including nested form groups in a reactive form.
Understanding this technique is very useful, as it can help us perform the following on all the form controls in a reactive form
- Reset form controls
- Enable or disable all form controls or just the nested formgroup controls
- Set validators and clear validators
- Mark form controls as dirty, touched, untouched, pristine etc.
- Move validation messages and the logic to show and hide them into the component class from the view template.
Here is what we want to do : Loop through each form control in the following employeeForm form group including nested skills form group and log the form control key and value to the console.
this.employeeForm = this.fb.group({
fullName: [''],
email: [''],
skills: this.fb.group({
skillName: [''],
experienceInYears: [''],
proficiency: ['beginner']
}),
});
For example, if we have the following sample data on the form

We want every form control key and value to be logged to the console as shown below.

Here is the method. It is commented and self-explanatory
logKeyValuePairs(group: FormGroup):
void {
// loop through each key in the FormGroup
Object.keys(group.controls).forEach((key: string) =>
{
// Get a reference to the control using the
FormGroup.get() method
const abstractControl = group.get(key);
// If the control is an instance of FormGroup i.e a
nested FormGroup
// then recursively call this same method
(logKeyValuePairs) passing it
// the FormGroup so we can get to the form controls
in it
if (abstractControl instanceof
FormGroup) {
this.logKeyValuePairs(abstractControl);
// If the control is not a FormGroup then we know
it's a FormControl
} else {
console.log('Key = '
+ key + ' && Value = '
+ abstractControl.value);
}
});
}
In our next video we will discuss, moving validation messages into the component class from the view template.
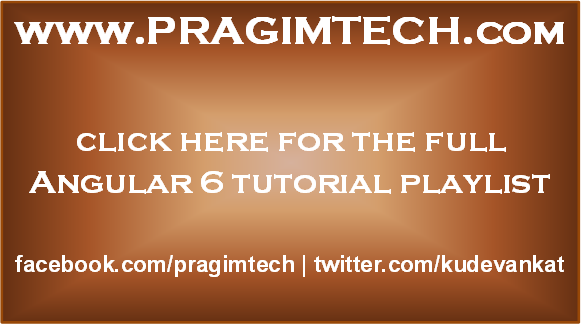
No comments:
Post a Comment
It would be great if you can help share these free resources