Suggested Videos
Part 7 - Angular setvalue and patchvalue methods | Text | Slides
Part 8 - Angular formbuilder example | Text | Slides
Part 9 - Angular reactive forms validation | Text | Slides
In this video we will discuss how to monitor and react when a form control or form group value changes.
Angular valueChanges Observable
For example, if you want to monitor and log to the console as the value of a fullName form control changes, subscribe to it's valueChanges observable as shown below.
We placed the code to subscribe to the valueChanges Observable in ngOnInit lifecycle hook. This is because, we want to start monitoring and reacting to fullName form control value immediately after the component is initialised.
Every time the value of the fullName form control changes, the value is passed as a parameter to the subscribe method and the associated code is executed.
Since FormGroup class also inherit from AbstractControl class, we can also subscribe to the FormGroup valueChanges observable. This allows us to monitor and react when any control value in that FormGroup changes.
Subscribing to valueChanges observable and there by monitoring a form control or form group allow us to do several things like
Part 7 - Angular setvalue and patchvalue methods | Text | Slides
Part 8 - Angular formbuilder example | Text | Slides
Part 9 - Angular reactive forms validation | Text | Slides
In this video we will discuss how to monitor and react when a form control or form group value changes.
Angular valueChanges Observable
- Both FormControl and FormGroup classes inherit from the base AbstractControl class
- AbstractControl class has valueChanges property
- valueChanges property is an observable that emits an event every time the value of the control changes
- To be able to monitor and react when the FormControl or FormGroup value changes, subscribe to the valueChanges observable

For example, if you want to monitor and log to the console as the value of a fullName form control changes, subscribe to it's valueChanges observable as shown below.
ngOnInit() {
this.employeeForm = this.fb.group({
fullName: ['',
[
Validators.required,
Validators.minLength(2),
Validators.maxLength(10)]
],
email: [''],
skills: this.fb.group({
skillName: ['C#'],
experienceInYears: [''],
proficiency: ['beginner']
}),
});
// Subscribe to valueChanges observable
this.employeeForm.get('fullName').valueChanges.subscribe(
value => {
console.log(value);
}
);
}
We placed the code to subscribe to the valueChanges Observable in ngOnInit lifecycle hook. This is because, we want to start monitoring and reacting to fullName form control value immediately after the component is initialised.
Every time the value of the fullName form control changes, the value is passed as a parameter to the subscribe method and the associated code is executed.
Since FormGroup class also inherit from AbstractControl class, we can also subscribe to the FormGroup valueChanges observable. This allows us to monitor and react when any control value in that FormGroup changes.
// Subscribe to FormGroup valueChanges observable
this.employeeForm.valueChanges.subscribe(
value => {
console.log(JSON.stringify(value));
}
);
Subscribing to valueChanges observable and there by monitoring a form control or form group allow us to do several things like
- Implementing auto-complete feature
- Dynamically validating form controls
- Move validation messages from the view template to the component class
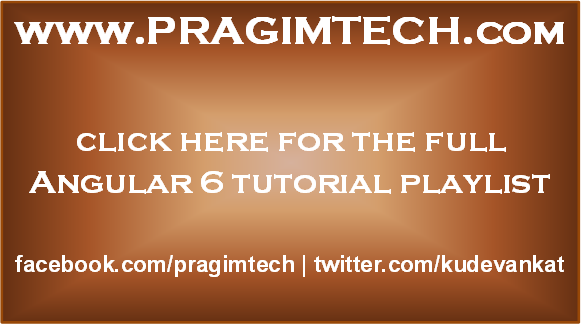
No comments:
Post a Comment
It would be great if you can help share these free resources