Suggested Videos
Part 42 - Subscribe to angular route parameter changes | Text | Slides
Part 43 - Angular optional route parameters | Text | Slides
Part 44 - Angular required route parameter vs optional route parameter | Text | Slides
In this video we will discuss, why and how to rest a form in angular.
Sometimes we must reset the form, before we can redirect the user to another route. Let me explain this with an example.
Angular form reset
There are 2 ways to RESET the form in code. One way is to pass the form (NgForm) to the Submit() method and then call reset() method.
saveEmployee(empForm: NgForm): void {
this._employeeService.save(this.employee);
empForm.reset();
this._router.navigate(['list']);
}
The other way is to use the @ViewChild decorator to access form in code and then call reset() method.
@ViewChild('employeeForm') public createEmployeeForm: NgForm;
saveEmployee(): void {
this._employeeService.save(this.employee);
this.createEmployeeForm.reset();
this._router.navigate(['list']);
}
Please note : @ViewChild() decorator provides access to the NgForm directive in the component class. employeeForm which is passed as the selector to the @ViewChild() decorator is the form template reference variable.
To reset and initialise the form controls with some default values, pass an object to the reset() method with key/value pairs. The key is the form control name and the value is the default value.
this.createEmployeeForm.reset({
name: 'Test Value',
email: 'kudvenkat@pragimtech.com'
});
Part 42 - Subscribe to angular route parameter changes | Text | Slides
Part 43 - Angular optional route parameters | Text | Slides
Part 44 - Angular required route parameter vs optional route parameter | Text | Slides
In this video we will discuss, why and how to rest a form in angular.
Sometimes we must reset the form, before we can redirect the user to another route. Let me explain this with an example.
- We have a form to create a new employee
- After we fill the form and when we click the "Save" button, the employee data must be saved and the user should be redirected to the "LIST" route.
- But canDeactivate guard is added on the "CREATE" route
- This means if the form is dirty, the canDecativate guard will prevent us from leaving the "CREATE" route
- So there is a need to to RESET the form, which will reset all the form control values to their intial state and also resets the form flags like dirty, pristine, valid, touched etc.
- Once the form is REST, the form is no longer dirty, so the canDeactivate guard will not prevent use from leaving the CREATE route
Angular form reset
- Clears all the form controls
- Optionally the form controls can be initialised with default values
- Resets the form state and individual controls state like dirty, pristine, valid, touched etc.
<form #employeeForm="ngForm"
(ngSubmit)="saveEmployee(employeeForm);
employeeForm.reset()">
There are 2 ways to RESET the form in code. One way is to pass the form (NgForm) to the Submit() method and then call reset() method.
saveEmployee(empForm: NgForm): void {
this._employeeService.save(this.employee);
empForm.reset();
this._router.navigate(['list']);
}
The other way is to use the @ViewChild decorator to access form in code and then call reset() method.
@ViewChild('employeeForm') public createEmployeeForm: NgForm;
saveEmployee(): void {
this._employeeService.save(this.employee);
this.createEmployeeForm.reset();
this._router.navigate(['list']);
}
Please note : @ViewChild() decorator provides access to the NgForm directive in the component class. employeeForm which is passed as the selector to the @ViewChild() decorator is the form template reference variable.
To reset and initialise the form controls with some default values, pass an object to the reset() method with key/value pairs. The key is the form control name and the value is the default value.
this.createEmployeeForm.reset({
name: 'Test Value',
email: 'kudvenkat@pragimtech.com'
});
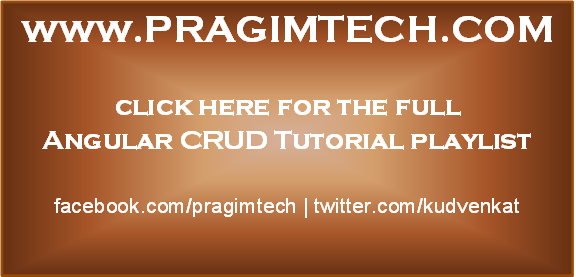
No comments:
Post a Comment
It would be great if you can help share these free resources