Suggested Videos
Part 17 - Adapter Design Pattern - Text - Slides
Part 18 - Bridge Design Pattern - Text - Slides
Part 19 - Composite Design Pattern - Text - Slides
In this video we will learn
Decorator Design Pattern : As per the GOF definition, decorator Pattern states that we need to “Attach additional responsibilities to an object dynamically. Decorators provide A flexible alternative to sub classing for extending functionality.”
This pattern Falls under the category of Structural Design Pattern and is also known as Wrapper.
Implementation Guidelines : We need to use Decorator Design Pattern when
Step 1 : Add Interface ICar
Step 2 : Create Concrete component Hyndai to implement ICar
Step 3 : Create Concrete component Suzuki to implement ICar
Step 4 : Create Decorator Class CarDecorator to decorate the Icar using CarDecorator
Step 5 : Create Concrete Decorator OfferPrice to decorate the Icar using CarDecorator
Step 6 : Use the car decorator in the main program to achieve the output
Step 7 : Run the application to see the expected output
Make : Sedan Price:1000000 DiscountPrice: 800000
Step 8 : Above mentioned classes are created under the below folder structure. Again it’s not mandatory to follow this folder structure as we are just doing this for representation purpose.
Part 17 - Adapter Design Pattern - Text - Slides
Part 18 - Bridge Design Pattern - Text - Slides
Part 19 - Composite Design Pattern - Text - Slides
In this video we will learn
- What is Decorator Design Pattern
- Implementation guidelines of decorator design pattern
- Simple Decorator Design Pattern example
Decorator Design Pattern : As per the GOF definition, decorator Pattern states that we need to “Attach additional responsibilities to an object dynamically. Decorators provide A flexible alternative to sub classing for extending functionality.”
This pattern Falls under the category of Structural Design Pattern and is also known as Wrapper.
Implementation Guidelines : We need to use Decorator Design Pattern when
- We need to add responsibilities to individual objects dynamically and transparently,
- The extension by sub classing is impractical.
- Class definition may be hidden or otherwise unavailable for sub classing.

- Client : Clients use the Component interface to interact with objects
- Component : Defines the interface for objects that can have responsibilities added to them dynamically.
- ConcreteComponent : Defines an object to which additional responsibilities can be attached.
- Decorator : Maintains a reference to a Component object and defines an interface that conforms to Component's interface.
- ConcreteDecorator : Adds responsibilities to the component.
Step 1 : Add Interface ICar
using System;
using
System.Collections.Generic;
using
System.Linq;
using
System.Text;
using
System.Threading.Tasks;
namespace
DecoratorDemo.Component
{
public interface ICar
{
string Make { get; }
double
GetPrice();
}
}
Step 2 : Create Concrete component Hyndai to implement ICar
using
DecoratorDemo.Component;
using System;
using
System.Collections.Generic;
using
System.Linq;
using
System.Text;
using
System.Threading.Tasks;
namespace
DecoratorDemo.ConcreteComponent
{
public sealed class Hyndai : ICar
{
public string Make
{
get { return "HatchBack"; }
}
public double GetPrice()
{
return 800000;
}
}
}
Step 3 : Create Concrete component Suzuki to implement ICar
using
DecoratorDemo.Component;
using System;
using
System.Collections.Generic;
using
System.Linq;
using
System.Text;
using
System.Threading.Tasks;
namespace
DecoratorDemo.ConcreteComponent
{
public sealed class Suzuki : ICar
{
public string Make
{
get { return "Sedan"; }
}
public double GetPrice()
{
return 1000000;
}
}
}
Step 4 : Create Decorator Class CarDecorator to decorate the Icar using CarDecorator
using
DecoratorDemo.Component;
using System;
using
System.Collections.Generic;
using
System.Linq;
using
System.Text;
using
System.Threading.Tasks;
namespace
DecoratorDemo.Decorator
{
public abstract class CarDecorator : ICar
{
private ICar car;
public
CarDecorator(ICar Car)
{
car = Car;
}
public string Make { get { return car.Make;
} }
public double GetPrice()
{
return car.GetPrice();
}
public abstract double
GetDiscountedPrice();
}
}
Step 5 : Create Concrete Decorator OfferPrice to decorate the Icar using CarDecorator
using
DecoratorDemo.Component;
using
DecoratorDemo.Decorator;
using System;
using
System.Collections.Generic;
using
System.Linq;
using
System.Text;
using
System.Threading.Tasks;
namespace
DecoratorDemo.ConcreteDecorator
{
public class OfferPrice :
CarDecorator
{
public
OfferPrice(ICar car) : base(car)
{
}
public override double
GetDiscountedPrice()
{
return .8 * base.GetPrice();
}
}
}
Step 6 : Use the car decorator in the main program to achieve the output
using
DecoratorDemo.Component;
using
DecoratorDemo.ConcreteComponent;
using
DecoratorDemo.ConcreteDecorator;
using
DecoratorDemo.Decorator;
using System;
using
System.Collections.Generic;
using
System.Linq;
using
System.Text;
using
System.Threading.Tasks;
namespace
DecoratorDemo
{
class Program
{
static void Main(string[] args)
{
ICar car = new Suzuki();
CarDecorator decorator = new
OfferPrice(car);
Console.WriteLine(string.Format("Make
:{0} Price:{1} " +
"DiscountPrice
: {2}"
, decorator.Make, decorator.GetPrice().ToString(),
decorator.GetDiscountedPrice().ToString()));
Console.ReadLine();
}
}
}
Step 7 : Run the application to see the expected output
Make : Sedan Price:1000000 DiscountPrice: 800000
Step 8 : Above mentioned classes are created under the below folder structure. Again it’s not mandatory to follow this folder structure as we are just doing this for representation purpose.

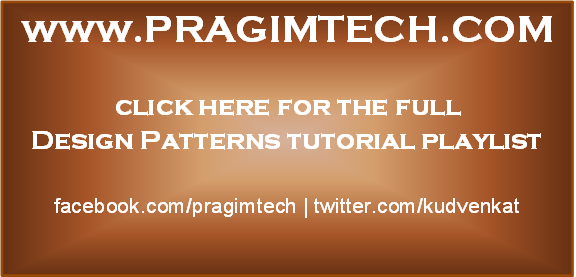
No comments:
Post a Comment
It would be great if you can help share these free resources